可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I want show a picture into imageView like the image contact (in a circle) But when I try show this, the imageView rescale his size and this doesn't show correctly in a circle..
image.layer.borderWidth=1.0
image.layer.masksToBounds = false
image.layer.borderColor = UIColor.whiteColor().CGColor
image.layer.cornerRadius = image.frame.size.height/2
image.clipsToBounds = true
I want show like this:
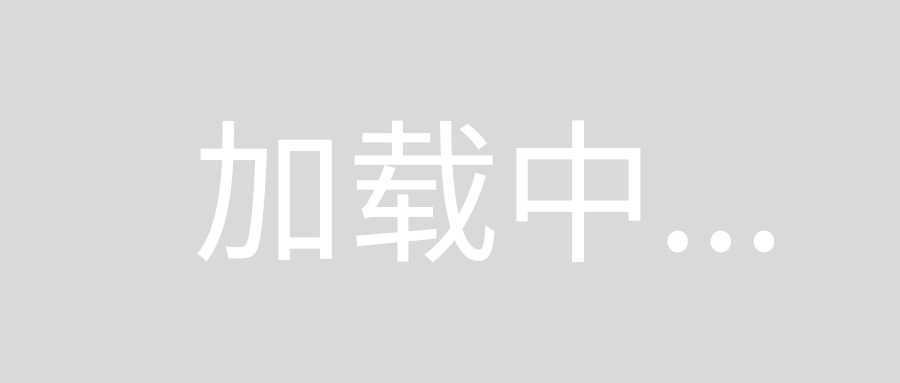
But I get this:
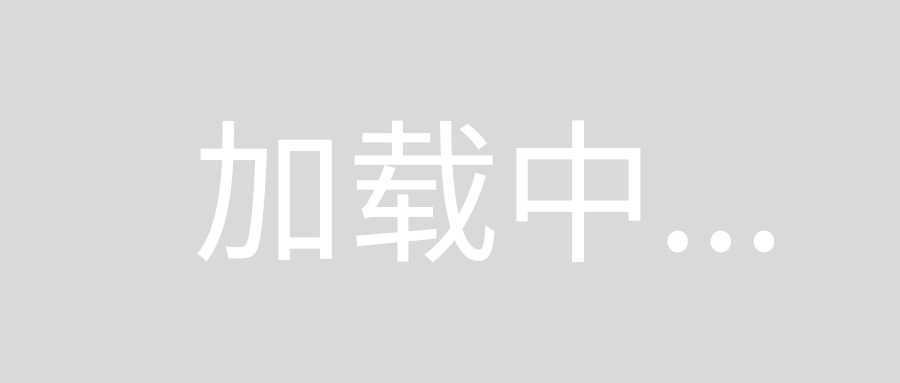
How can do that image resize to UIImageView size to show like circle?
Thanks!
回答1:
What frame
size are you using for image
? I can get a perfect circle if I set the frame to be a square.
let image = UIImageView(frame: CGRectMake(0, 0, 100, 100))
回答2:
This is solution which I have used in my app:
var image: UIImage = UIImage(named: "imageName")
imageView.layer.borderWidth = 1.0
imageView.layer.masksToBounds = false
imageView.layer.borderColor = UIColor.whiteColor().CGColor
imageView.layer.cornerRadius = image.frame.size.width/2
imageView.clipsToBounds = true
Swift 4.0
let image = UIImage(named: "imageName")
imageView.layer.borderWidth = 1.0
imageView.layer.masksToBounds = false
imageView.layer.borderColor = UIColor.white.cgColor
imageView.layer.cornerRadius = image.frame.size.width / 2
imageView.clipsToBounds = true
回答3:
Fast and Simple solution.
How to mask UIImage
to Circle without cropping with Swift.
extension UIImageView {
public func maskCircle(anyImage: UIImage) {
self.contentMode = UIViewContentMode.ScaleAspectFill
self.layer.cornerRadius = self.frame.height / 2
self.layer.masksToBounds = false
self.clipsToBounds = true
// make square(* must to make circle),
// resize(reduce the kilobyte) and
// fix rotation.
self.image = anyImage
}
}
How to call:
let anyAvatarImage:UIImage = UIImage(named: "avatar")!
avatarImageView.maskCircle(anyAvatarImage)
回答4:
I would suggest making your image file a perfect square to begin with. This can be done in almost any photo editing program. After that, this should work within viewDidLoad. Credit to this video
image.layer.cornerRadius = image.frame.size.width/2
image.clipsToBounds = true
回答5:
That is all you need....
profilepic = UIImageView(frame: CGRectMake(0, 0, self.view.bounds.width * 0.19 , self.view.bounds.height * 0.1))
profilepic.layer.borderWidth = 1
profilepic.layer.masksToBounds = false
profilepic.layer.borderColor = UIColor.blackColor().CGColor
profilepic.layer.cornerRadius = profilepic.frame.height/2
profilepic.clipsToBounds = true
slider.addSubview(profilepic)
回答6:
Create your custom circle UIImageView and create the circle under the layoutSubviews helps if you use Autolayout.
/*
+-------------+
| |
| |
| |
| |
| |
| |
+-------------+
The IMAGE MUST BE SQUARE
*/
class roundImageView: UIImageView {
override init(frame: CGRect) {
// 1. setup any properties here
// 2. call super.init(frame:)
super.init(frame: frame)
// 3. Setup view from .xib file
}
required init?(coder aDecoder: NSCoder) {
// 1. setup any properties here
// 2. call super.init(coder:)
super.init(coder: aDecoder)
// 3. Setup view from .xib file
}
override func layoutSubviews() {
super.layoutSubviews()
self.layer.borderWidth = 1
self.layer.masksToBounds = false
self.layer.borderColor = UIColor.white.cgColor
self.layer.cornerRadius = self.frame.size.width/2
self.clipsToBounds = true
}
}
回答7:
I fixed it doing modifying the view:
- Go to your Main.storyboard
- Click on your image
- View -> Mode -> Aspect Fill
It works perfectly
回答8:
This work perfectly for me.
The order of lines is important
func circularImage(photoImageView: UIImageView?)
{
photoImageView!.layer.frame = CGRectInset(photoImageView!.layer.frame, 0, 0)
photoImageView!.layer.borderColor = UIColor.grayColor().CGColor
photoImageView!.layer.cornerRadius = photoImageView!.frame.height/2
photoImageView!.layer.masksToBounds = false
photoImageView!.clipsToBounds = true
photoImageView!.layer.borderWidth = 0.5
photoImageView!.contentMode = UIViewContentMode.ScaleAspectFill
}
How to use:
@IBOutlet weak var photoImageView: UIImageView!
...
...
circularImage(photoImageView)
回答9:
what i found out is that your width and height of image view must return an even number when divided by 2 to get a perfect circle e.g
let image = UIImageView(frame: CGRectMake(0, 0, 120, 120))
it should not be something like
let image = UIImageView(frame: CGRectMake(0, 0, 130, 130))
回答10:
This also works for me. For perfect circle result, use the same size for width and height. like image.frame = CGRect(0,0,200, 200)
For non perfect circle, width and height should not be equal like this codes below.
image.frame = CGRect(0,0,200, 160)
image.layer.borderColor = UIColor.whiteColor().CGColor
image.layer.cornerRadius = image.frame.size.height/2
image.layer.masksToBounds = false
image.layer.clipsToBounds = true
image.contentMode = .scaleAspectFill
回答11:
I had a similar result (more of an oval than a circle). It turned out that the constraints I set on the UIImageView
forced it into an oval instead of a circle. After fixing that, the above solutions worked.
回答12:
reviewerImage.layer.cornerRadius = reviewerImage.frame.size.width / 2;
reviewerImage.clipsToBounds = true
回答13:
try this.
swift code
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(true)
perform(#selector(self.setCircleForImage(_:)), with: pickedImage, afterDelay: 0)
}
@objc func setCircleForImage(_ imageView : UIImageView){
imageView.layer.cornerRadius = pickedImage.frame.size.width/2
imageView.clipsToBounds = true
}
回答14:
Use this code to make image round
self.layoutIfNeeded()
self.imageView.layer.cornerRadius =
self.imageView.frame.width/2
self.imageView.layer.masksToBounds = true
回答15:
If your using a UIViewController
here's how do do it using Anchors. The key is to set the imageView's layer.cornerRadius
in viewWillLayoutSubviews
like so:
override func viewWillLayoutSubviews(){
imageView.layer.cornerRadius = imageView.frame.size.width / 2
}
Also make sure the heightAnchor
and widthAnchor
are the same size. They are both 100
in my example below
Code:
let imageView: UIImageView = {
let imageView = UIImageView()
imageView.translatesAutoresizingMaskIntoConstraints = false
imageView.layer.borderWidth = 1.0
imageView.clipsToBounds = true
imageView.layer.borderColor = UIColor.white.cgColor
return imageView
}()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(imageView)
imageView.heightAnchor.constraint(equalToConstant: 100).isActive = true
imageView.widthAnchor.constraint(equalToConstant: 100).isActive = true
imageView.centerXAnchor.constraint(equalTo: view.centerXAnchor).isActive = true
imageView.topAnchor.constraint(equalTo: view.safeAreaLayoutGuide.topAnchor, constant: 50).isActive = true
imageView.image = UIImage(named: "pizzaImage")
}
override func viewWillLayoutSubviews() {
imageView.layer.cornerRadius = imageView.frame.size.width / 2
}
If your using a CollectionView Cell
set the imageView's layer.cornerRadius
in layoutSubviews()
:
override init(frame: CGRect) {
super.init(frame: frame)
addSubview(imageView)
imageView.heightAnchor.constraint(equalToConstant: 100).isActive = true
imageView.widthAnchor.constraint(equalToConstant: 100).isActive = true
imageView.centerXAnchor.constraint(equalTo: self.centerXAnchor).isActive = true
imageView.topAnchor.constraint(equalTo: self.topAnchor, constant: 50).isActive = true
imageView.image = UIImage(named: "pizzaImage")
}
override func layoutSubviews() {
super.layoutSubviews() // call super.layoutSubviews()
imageView.layer.cornerRadius = imageView.frame.size.width / 2
}
回答16:
Try this it's work for me ,
set imageView width and height same .
Swift
imageview?.layer.cornerRadius = (imageview?.frame.size.width ?? 0.0) / 2
imageview?.clipsToBounds = true
imageview?.layer.borderWidth = 3.0
imageview?.layer.borderColor = UIColor.white.cgColor
Screenshot
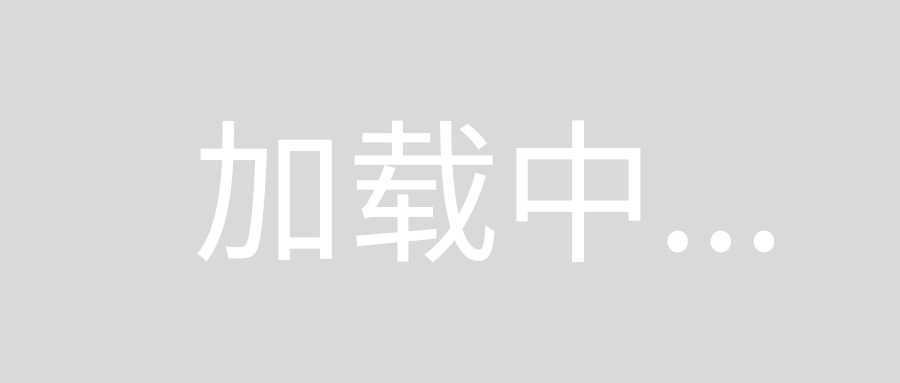
Objective C
self.imageView.layer.cornerRadius = self.imageView.frame.size.width / 2;
self.imageView.clipsToBounds = YES;
self.imageView.layer.borderWidth = 3.0f;
self.imageView.layer.borderColor = [UIColor whiteColor].CGColor;
Hope this will help to some one .