i want to send email programmatically.
i tried out the following code.
final Intent emailIntent = new Intent(
android.content.Intent.ACTION_SEND);
emailIntent.setType("plain/text");
emailIntent.putExtra(android.content.Intent.EXTRA_EMAIL,
new String[] { "abc@gmail.com" });
emailIntent.putExtra(android.content.Intent.EXTRA_SUBJECT,
"Email Subject");
emailIntent.putExtra(android.content.Intent.EXTRA_TEXT,
"Email Body");
startActivity(Intent.createChooser(
emailIntent, "Send mail..."));
but problem is that before sending email the application open the activity
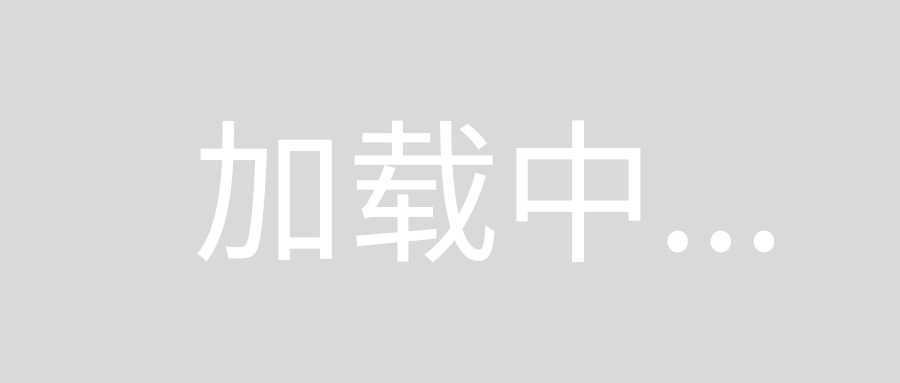
i want to send email directly without open compose activity. how this possible?
Look at the link, there is an answer for your question.
Sending Email in Android using JavaMail API without using the default/built-in app
Referred link has correct answer, but there are written some libraries to make your work easy.
- https://github.com/yesidlazaro/GmailBackground
- https://github.com/thegenuinegourav/Android-Email-App-using-Javamail-Api
- https://github.com/prashantwosti/BackgroundMailLibrary
So don't write all code again, just use any of these library and get your work done in little time.
It might be an easiest way-
String recipientList = mEditTextTo.getText().toString();
String[] recipients = recipientList.split(",");
String subject = mEditTextSubject.getText().toString();
String message = mEditTextMessage.getText().toString();
Intent intent = new Intent(Intent.ACTION_SEND);
intent.putExtra(Intent.EXTRA_EMAIL, recipients);
intent.putExtra(Intent.EXTRA_SUBJECT, subject);
intent.putExtra(Intent.EXTRA_TEXT, message);
intent.setType("message/rfc822");
startActivity(Intent.createChooser(intent, "Choose an email client"));