I use Babel and Google Chrome Developer Tools with JavaScript source maps enabled. Given this code
function myFunc(elements) {
return elements
.map(element => element.value)
.filter(value => value >= 0);
}
how can I pause execution at execution of lambda function element => element.value
? If I set a breakpoint at line of .map(element => element.value)
it will only pause when map is executed, but not when the lambda function is executed.
This feature is finally available (at least in Google Chrome 58). Click on the line number of the line of your lambda you like to debug (here line 3). Then activate the marker in your lambda (here the second) by clicking it. Further, I disabled the first marker here, which would pause on the map
call (not the lambda):
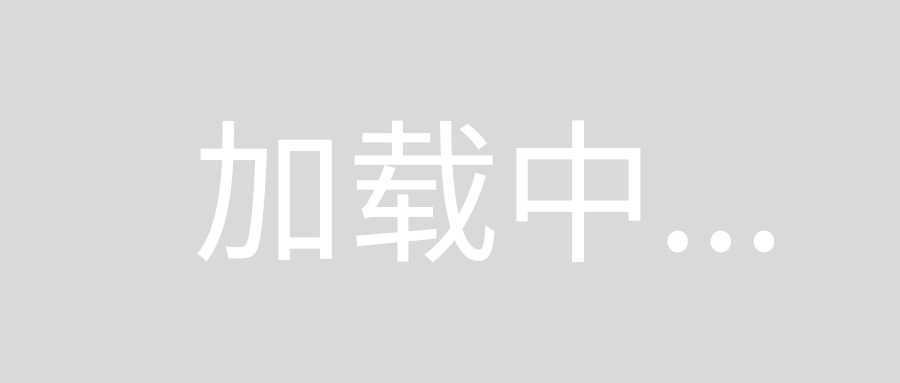
When your program runs and hits the breakpoint, it will pause and you can inspect variables:
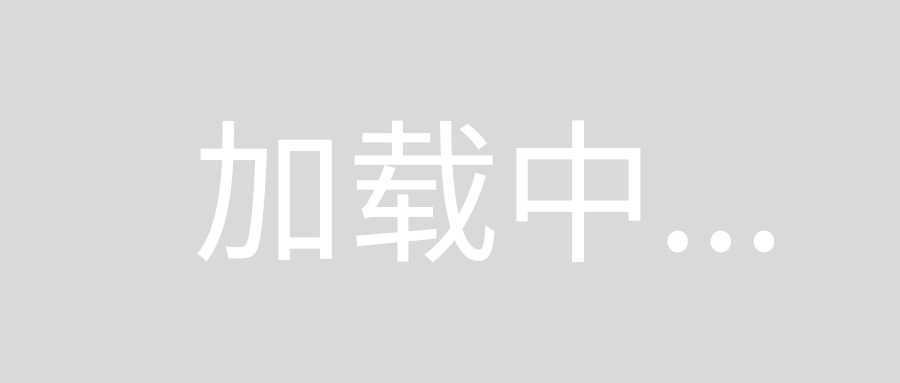
You can use the debugger
keyword to signal the debugger to pause at that location and it can be inserted just like any JavaScript statement.
function myFunc(elements) {
return elements
.map(element => {debugger; return element.value})
.filter(value => value >= 0);
}
You can do as follows:
function myFunc(elements) {
return elements
.map(element => {
element.value
})
.filter(value => value >= 0);
}
This way you can add a breakpoint in line element.value
Couldn't find a way to get it work without code changing.
If anybody can find a way please tell.