I am trying to get my head around using hypergraphs in pygraph, the following is a simple example I inserted:
hgr = hypergraph()
hgr.add_nodes(["A1", "B1", "C1", "D1"])
hgr.add_nodes(["A2", "B2", "C2", "D2"])
hgr.add_nodes(["A3", "B3", "C3", "D3"])
hgr.add_nodes(["A4", "B4", "C4", "D4"])
hgr.add_hyperedge(("A1", "A2", "A3", "A4"))
hgr.add_hyperedge(("B1", "B2", "B3", "B4"))
hgr.add_hyperedge(("C1", "C2", "C3", "C4"))
hgr.add_hyperedge(("D1", "D2", "D3", "D4"))
h_dot = write(hgr)
h_gvv = gv.readstring(h_dot)
gv.layout(h_gvv,'dot')
gv.render(h_gvv, 'png', 'hypergraph.png')
The image I am getting is (click to see full size version):
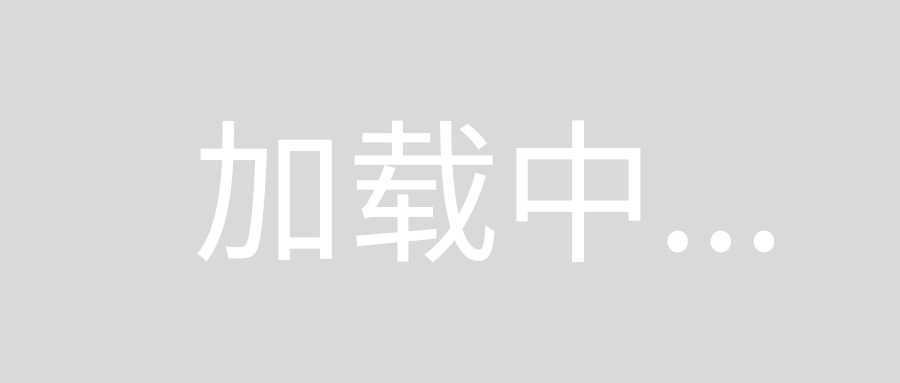
Please verify that this is the correct way to create hypergraphs using pygraph.
Much appreciated!
Since each hyperedge isn't a collection of nodes, but instead represented as a node, you should use an unique (simple) identifier for the hyperedges, just as you do for the nodes, and then link them to the nodes.
Consider the example graph in the Wikipedia article for hypergraphs:
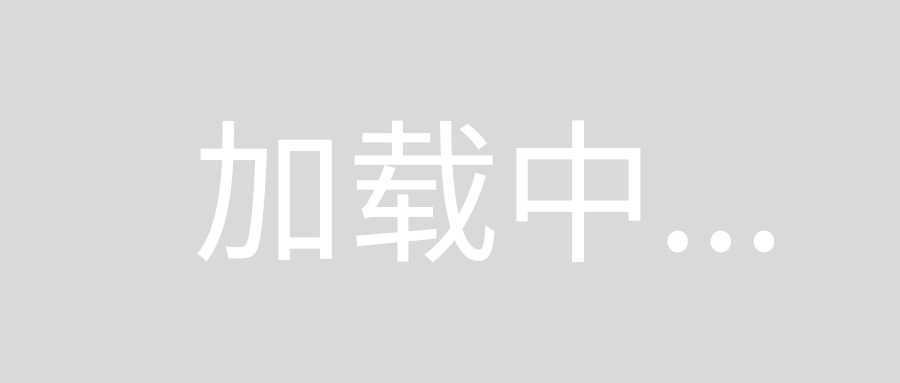
To create this graph in pygraph
you could do the following:
from pygraph.classes.hypergraph import hypergraph
from pygraph.readwrite.dot import write_hypergraph
h = hypergraph()
h.add_nodes(['v1', 'v2', 'v3', 'v4', 'v5', 'v6', 'v7'])
h.add_hyperedges(['e1', 'e2', 'e3', 'e4'])
h.link('v1', 'e1')
h.link('v2', 'e1')
h.link('v3', 'e1')
h.link('v2', 'e2')
h.link('v3', 'e2')
h.link('v3', 'e3')
h.link('v5', 'e3')
h.link('v6', 'e3')
h.link('v4', 'e4')
with open('hypergraph.dot', 'w') as f:
f.write(write_hypergraph(h))
which will produce this image output with dot
:
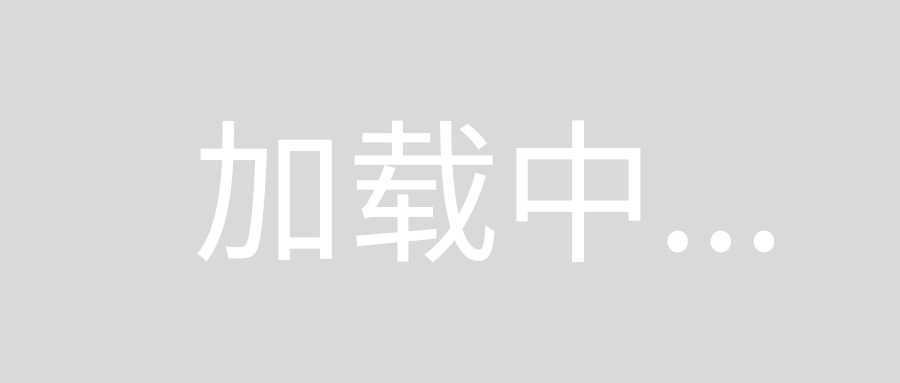
This is a correct representation I guess, but not as visual as the image from Wikipedia. If you are pursuing a visualization of hypergraphs, you should check out this question.