I requirement for an inline SVG chart which has a "more information" icon which should trigger "tooltip" on hover. See attached.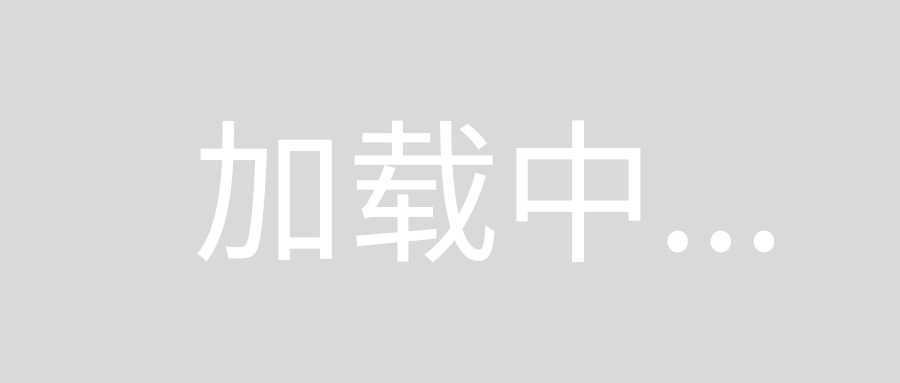
I have the tooltip div styled and is used in other places but I also need it to trigger on the information icon inside the SVG.
I know I can't add the tooltip html inside SVG, so what other options are available to me?
The "chart" is just inline SVG taken directly from a graphic programme (Sketch in this instance). Am not using any frameworks or libraries like D3 or chartjs. Please don't suggest to use a library or framework as it's not an option.
Similar SO question but they don't answer my question:
How to create an SVG "tooltip"-like box?
It's pretty simple. It just requires a few lines of Javascript.
When we mouse over the icon, we position the popup and show it. On mouseout, we hide it again.
Also note the pointer-events="all"
on the icon, which ensures that the mouse is considered "over" an icon element even for the bits that have an invisible fill.
var myicon = document.getElementById("myicon");
var mypopup = document.getElementById("mypopup");
myicon.addEventListener("mouseover", showPopup);
myicon.addEventListener("mouseout", hidePopup);
function showPopup(evt) {
var iconPos = myicon.getBoundingClientRect();
mypopup.style.left = (iconPos.right + 20) + "px";
mypopup.style.top = (window.scrollY + iconPos.top - 60) + "px";
mypopup.style.display = "block";
}
function hidePopup(evt) {
mypopup.style.display = "none";
}
body {
background-color: #33333f;
}
#mypopup {
width: 400px;
padding: 20px;
font-family: Arial, sans-serif;
font-size: 10pt;
background-color: white;
border-radius: 6px;
position: absolute;
display: none;
}
#mypopup::before {
content: "";
width: 12px;
height: 12px;
transform: rotate(45deg);
background-color: white;
position: absolute;
left: -6px;
top: 68px;
}
<svg width="400" height="400">
<g id="myicon" pointer-events="all">
<circle cx="100" cy="150" r="14" fill="none" stroke="gold" stroke-width="2"/>
<circle cx="100" cy="144" r="2" fill="gold"/>
<rect x="98.5" y="148" width="3" height="10" fill="gold"/>
</g>
</svg>
<div id="mypopup">
<h3>Popup title</h3>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</p>
<p>Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</p>
</div>