I am creating a website based on soundcloud and i need to modify the soundcloud waveform color to look like the following
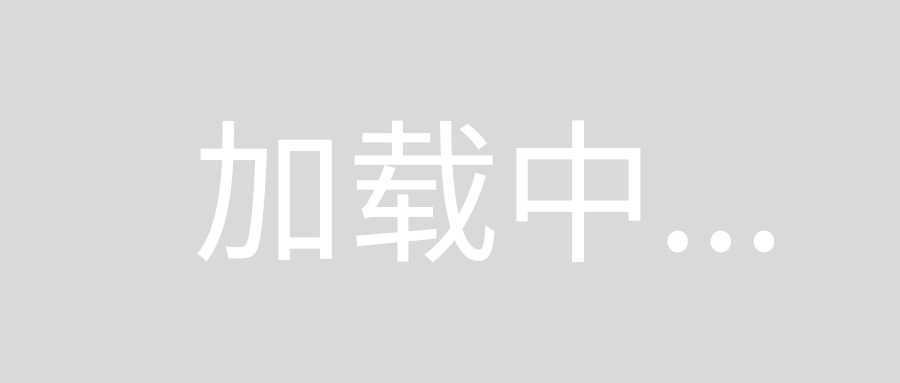
I read in the blog of soundcloud and it seemed like waveform.js will do the trick but still i am getting something like this
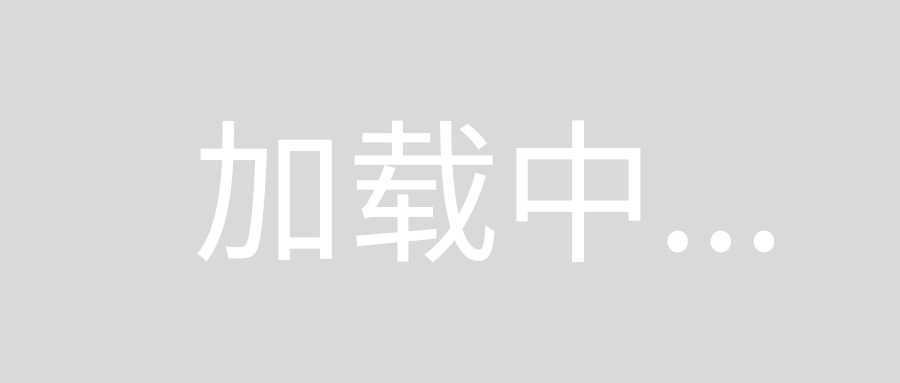
Can anyone let me know how can i get the exact same effect. I saw on soundcloud homepage they are doing same thing using canvas but i don't how exactly to do that. The image waveform returned from soundcloud api is as follows
http://w1.sndcdn.com/1m91TxE6jSOz_m.png
Can anyone guide me the right way to achieve this.
You can use a combination of composite mode and clipping to achieve this without iterating through pixels (which means CORS won't be a problem in this example):
Fiddle
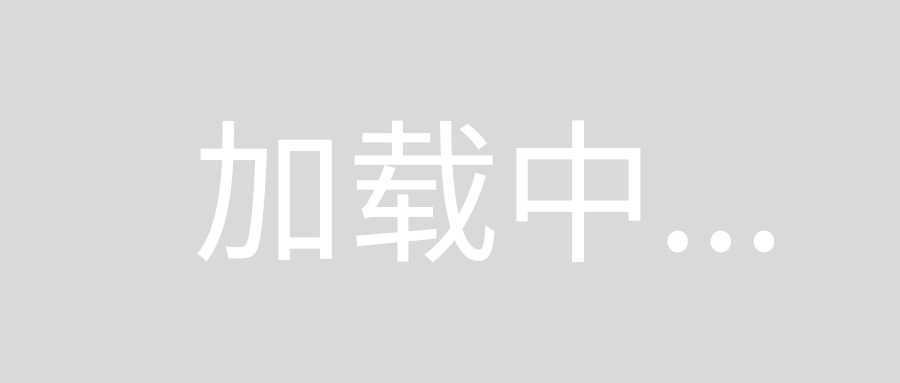
The essential code:
Assuming image has been loaded, canvas setup we are good to go:
function loop() {
x++; // sync this with actual progress
// since we will change composite mode and clip:
ctx.save();
// clear background with black
ctx.fillStyle = '#000';
ctx.fillRect(0, 0, w, h);
// remove waveform, change composite mode
ctx.globalCompositeOperation = 'destination-atop';
ctx.drawImage(img, 0, 0, w, h);
// fill new alpha, same mode, different region.
// as this will remove anything else we need to clip it
ctx.fillStyle = '#00a'; /// gradient
ctx.rect(0, 0, x, h);
ctx.clip(); /// set clipping rect
ctx.fill(); /// use the same rect to fill
// remove clip and use default composite mode
ctx.restore();
// loop until end
if (x < w) requestAnimationFrame(start);
}
If you want the "unplayed" waveform to have a different color simply style the canvas element with a background
.
If you are integrating soundcloud with iframe, you can't modify it.
Don't forget the cross-domain policy, otherwise it won't work.