This question in regarding formatting plots produced using ggplot2 + ggExtra and is not related to any bug.
require(ggplot2)
#> Loading required package: ggplot2
require(ggExtra)
#> Loading required package: ggExtra
p1 <- ggplot(data = mpg,aes(x = cty,y = cty)) +
geom_point()+
xlab("City driving (miles/gallon)") +
ylab("City driving (miles/gallon)")
ggMarginal(p = p1,type= "boxplot")
The y-axis marginal plot in this chart is usually not similar to the x-axis marginal plot i.e. the width of the 2 boxplots are not similar. This problem become more acute when I change plot dimensions (in my case, using RStudio). Any suggestions how to make the width of the 2 boxplots similar while using different plot dimensions (width x height).
I face similar problems with other marginal plot type options provided by ggExtra package: histogram, density.
I suggest the axis_canvas
function from the cowplot package. (Disclaimer: I'm the package author.) It requires a little more work, but it allows you to draw any marginals you want. And you can specify the size exactly, in output units (e.g. inch).
require(cowplot)
pmain <- ggplot(data = mpg, aes(x = cty, y = hwy)) +
geom_point() +
xlab("City driving (miles/gallon)") +
ylab("Highway driving (miles/gallon)")
xbox <- axis_canvas(pmain, axis = "x", coord_flip = TRUE) +
geom_boxplot(data = mpg, aes(y = cty, x = 1)) + coord_flip()
ybox <- axis_canvas(pmain, axis = "y") +
geom_boxplot(data = mpg, aes(y = hwy, x = 1))
p1 <- insert_xaxis_grob(pmain, xbox, grid::unit(1, "in"), position = "top")
p2 <- insert_yaxis_grob(p1, ybox, grid::unit(1, "in"), position = "right")
ggdraw(p2)
See how the boxplots retain their width/height in the following two images with different aspect ratios. (Unfortunately Stackoverflow rescales the images, so the effect is somewhat obscured, but you can see that the height of the top boxplot is always equal to the width of the side one.)
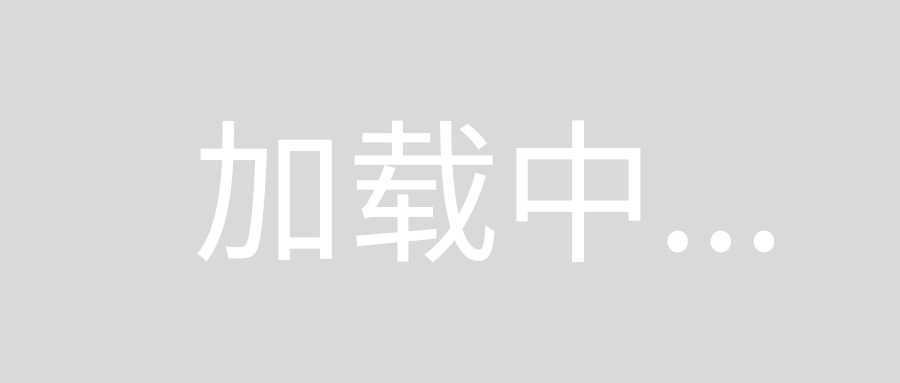
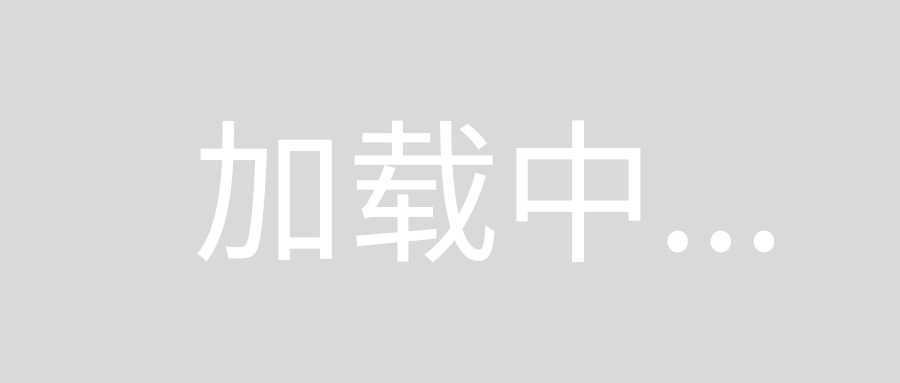
The second advantage is that because you can use full-blown ggplot2 for your marginal plots, you can draw anything you want, e.g. grouped box plots.
require(cowplot)
pmain <- ggplot(data = mpg, aes(x = cty, y = hwy, color = factor(cyl))) +
geom_point() +
xlab("City driving (miles/gallon)") +
ylab("Highway driving (miles/gallon)") +
theme_minimal()
xbox <- axis_canvas(pmain, axis = "x", coord_flip = TRUE) +
geom_boxplot(data = mpg, aes(y = cty, x = factor(cyl), color = factor(cyl))) +
scale_x_discrete() + coord_flip()
ybox <- axis_canvas(pmain, axis = "y") +
geom_boxplot(data = mpg, aes(y = hwy, x = factor(cyl), color = factor(cyl))) +
scale_x_discrete()
p1 <- insert_xaxis_grob(pmain, xbox, grid::unit(1, "in"), position = "top")
p2 <- insert_yaxis_grob(p1, ybox, grid::unit(1, "in"), position = "right")
ggdraw(p2)
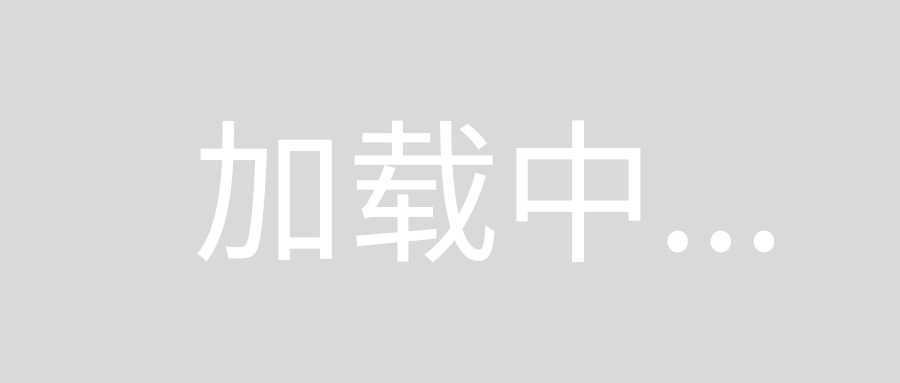
I'm not entirely sure what you mean. Setting width=height of your output plot ensures the same width of the boxplots.
For example, in RMarkdown, if I include
```{r, fig.width = 5, fig.height = 5}
ggMarginal(p1, type = "boxplot", size = 2);
```
I get the following output
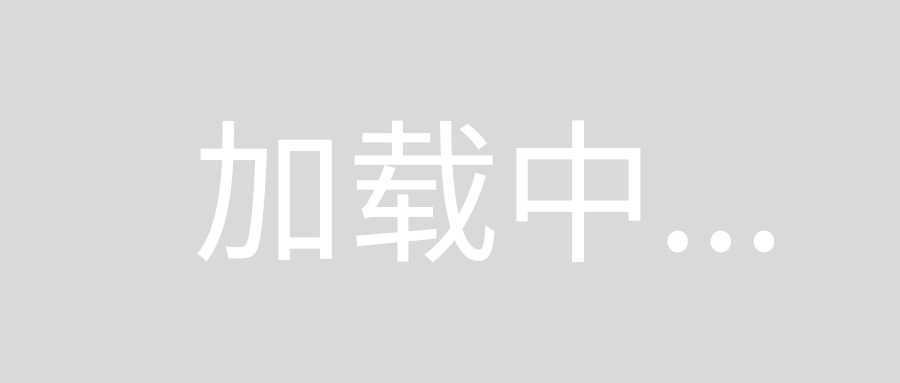
The box widths are identical.
Alternatively, if you save your plot make sure to set the same width and height.
ggsave(file = "test.png", ggMarginal(p1, type = "boxplot", size = 2), width = 5, height = 5);