I would like to get the same texture as the circle that indicates your position and bearing (blue) on the map in order to add other circles (in red) with the same texture and behaviour.
What I have:
I have access to all the params concerning the red point : LatLng, Bearing
...
I used a map.addCircle
to get the result displayed below.
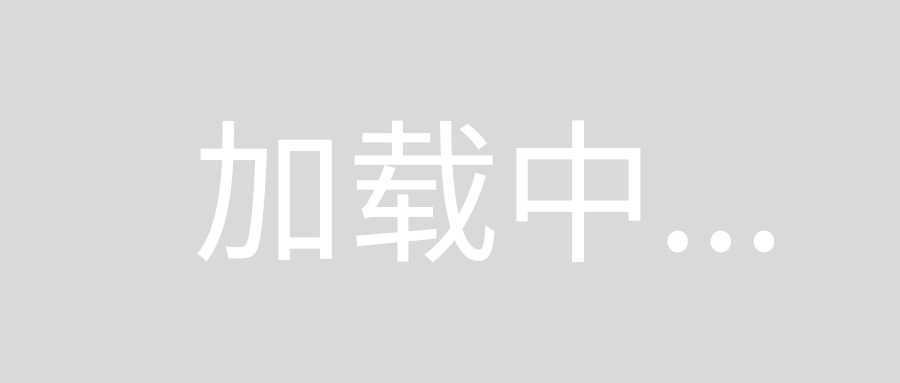
map.addCircle(new CircleOptions()
.center(position1)
.fillColor(Color.RED)
.strokeColor(Color.WHITE)
.radius(1.2)
.strokeWidth(1 / 4));
Needs:
I need the circle to be independant of the tilt and independant of the zoom, ie its size adapts to the zoom level and it behaves just like the blue circle.
Having the bearing displayed is essential.
Having the precision indication (the wide circle is optional).
EDIT 1:
I think that this blue dot may be in the com.google.android.gms.maps.R.drawable
directory but I can't find a way to get the info, or to get access to the list of drawables !
EDIT 2:
Using Apps Ressources (available on the PlayStore, I came to find that a blue_dot exists in the drawable folder of Maps. So I tried :
.icon(BitmapDescriptorFactory.fromResource(com.google.android.gms.maps.R.drawable.blue_dot)
But I got a Cannot Resolve Symbol... Any idea ?
EDIT 3... :
To complete what I was saying before, here is a screenshot showing that the ressources is in the Maps drawables:
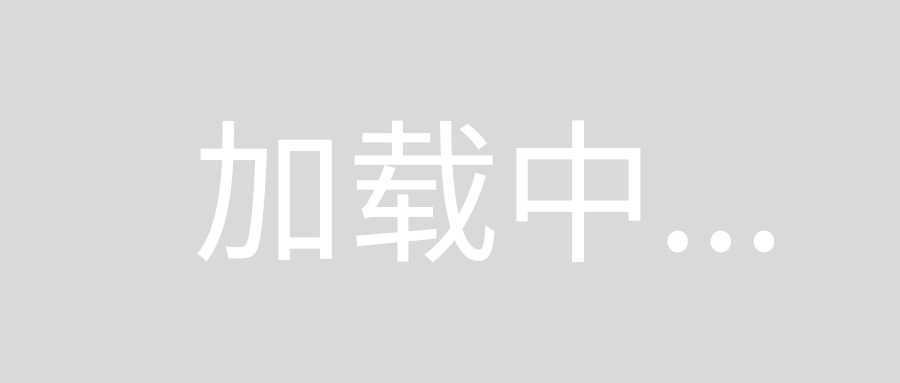
And this is the list of drawables that seem available in my AndroidStudio :
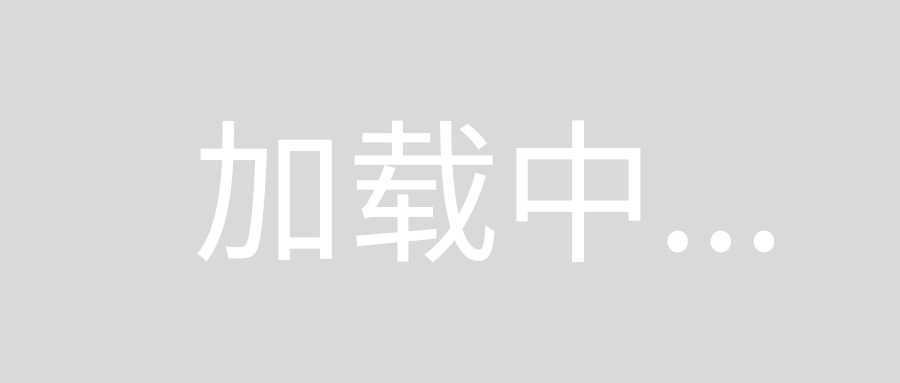
You can't access the blue dot resource because it is not public. Anyway it is a blue dot with the bearing part added as part of the resource.
We can't know how to create it (there is no explanation or source code), but you can try to replicate it by:
Create a resource which is a dot (blue or red as you want) and that have the "bearing" indicator on top of it (like the standard one), and put the 'arrow' to point the up center part of the resource.
Se this as a marker resource, put it as flat ( setFlat(boolean flat) ) and change the rotation according to the bearing you want; from the documentation:
Rotation
The rotation of the marker in degrees clockwise about the
marker's anchor point. The axis of rotation is perpendicular to the
marker. A rotation of 0 corresponds to the default position of the
marker. When the marker is flat on the map, the default position is
North aligned and the rotation is such that the marker always remains
flat on the map. When the marker is a billboard, the default position
is pointing up and the rotation is such that the marker is always
facing the camera. The default value is 0.
You have to pay attention to the "anchor" of the marker, which must be set to the center of the dot, not the center of the resource (otherwise it will not work correctly). In order to do it, place the dot in the center of the resource (according to the bearing arrow dimension) or change the anchor when adding the marker:
setAnchor (float anchorU, float anchorV)
documentation is https://developers.google.com/android/reference/com/google/android/gms/maps/model/Marker#setAnchor(float, float)
It is easier to center the dot in the resource, but the resource will be bigger.
About the glow
This is "worse" to do since you can't draw it in the same way they do (again, I'm supposing they are not using standard API, I can't know).
You can add a circle shape with center the same as the marker and play a bit with colors and circle dimensions:
// Instantiates a new CircleOptions object and defines the center and radius
CircleOptions circleOptions = new CircleOptions()
.center(new LatLng(POS_LAT, POS_LNG))
.radius(30) // radius in meters
.fillColor(0x8800CCFF) //this is a half transparent blue, change "88" for the transparency
.strokeColor(Color.BLUE) //The stroke (border) is blue
.strokeWidth(2)); // The width is in pixel, so try it!
// Get back the mutable Circle
Circle circle = myMap.addCircle(circleOptions);
The only thing i can't help is on the dimension of the dot when zooming out. Unfortunately gmaps automatically resize it, so in order to keep it small, you have to work with the GoogleMap.OnCameraChangeListener and resize the marker when zooming (this is a mess to do in a good way!)
I partially agree with N.Dorigatti, you're in the right way.
There is no such thing as "can't do" or "hidden API". Blue dot is a bitmap resource resized to an acceptable size and given an anchor at (0.5f,0.5f) of a plain old Marker. It remains at constant size throughout zoom unlike circle.
BitmapDescriptor blueDot = BitmapDescriptorFactory.fromResource(R.drawable.your_blue_dot);
MarkerOptions mo = new MarkerOptions()
.position(new LatLng(yourLatitude, yourLongitude))
.title("dot example")
.snippet("seriously")
.icon(blueDot)
.anchor(0.5f, 0.5f);
yourMap.addMarker(mo);
Feel free to correct me if I'm wrong.
It works great for my app though.
This way you can even display a Mickey-Mouse if needed.
Use .png with transparent surround.
The image must be BitmapDescriptor type. It can easily converted from Bitmap if there is a need for Bitmap-type working which I had.