The code for FAB:
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|right|end"
android:layout_margin="16dp"
android:src="@drawable/add_chat"
android:tint="@android:color/white" />
</android.support.design.widget.CoordinatorLayout>
The code for gradient background:
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="oval">
<gradient
android:angle="90"
android:endColor="#e4849f"
android:startColor="#d96e30" />
</shape>
android:backgroundTint="" or app:backgroundTint="" both uses color resources only.Can anyone suggest how to do this?
Thanks !!
Step 1. Create a new drawable.xml and add this code to it
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="oval">
<gradient
android:type="linear"
android:angle="0"
android:startColor="#f6ee19"
android:endColor="#115ede" />
</shape>
</item>
<item android:gravity="center"
>
<bitmap android:src="@drawable/your_icon"
android:gravity="fill_horizontal" />
</item>
</layer-list>
Step 2.) Now in your dimens.xml add this line of code,
<dimen name="design_fab_image_size" tools:override="true">56dp</dimen>
Step 3.) Finally set this drawable.xml in FAB using *android:src="@drawable/drawable.xml"*
P.S. - Make sure your_icon is a PNG, JPEG.
No, it is not possible to give a custom drawable or gradient or the FAB icon without breaking the UI guidelines. The icon only accepts backgroundTint
to the FAB icon. The UI guidelines clearly states that
Don’t give the floating action button extra dimension.
Read this:Material Guidelines FAB icon
If you still want to implement the gradient on FAB, you could replicate the FAB icon using a simple button and then give gradient color to it.
This method works also.
Create a shape called fab_background.xml in drawable where you can do all the customization you want
<?xml version="1.0" encoding="utf-8"?>
<shape android:shape="oval" xmlns:android="http://schemas.android.com/apk/res/android">
<size android:width="150dp" android:height="150dp"/>
<gradient android:startColor="@color/colorAccentDark" android:endColor="@color/colorAccent"/>
</shape>
Create a layout called custom_fab.xml in layout then set the backround and fab icon using the image view
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="50dp"
android:layout_height="50dp"
android:gravity="center"
android:background="@drawable/fab_background"
>
<android.appcompat.widget.AppCompatImageView
android:layout_width="24dp"
android:layout_height="24dp"
android:src="@drawable/right" />
</LinearLayout>
You can then reference it using include
Example
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/background"
android:orientation="vertical">
<include layout="@layout/custom_fab"
android:layout_width="45dp"
android:layout_height="45dp"
android:layout_alignParentEnd="true"
android:layout_alignParentBottom="true"
android:layout_margin="32dp"/>
</RelativeLayout>
The accepted answer is working fine but after adding the below line, it stretches the icon added on FAB
<dimen name="design_fab_image_size" tools:override="true">56dp</dimen>
I am sharing the screenshots:
Previous FAB button
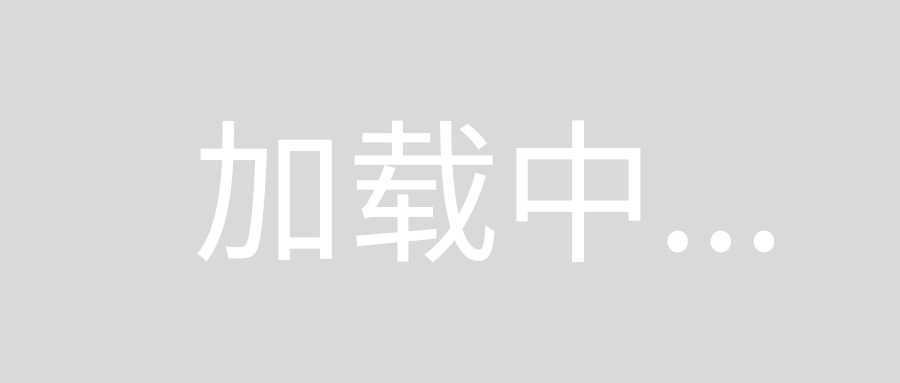
After adding design_fab_image_size in dimen.xml and adding android:src="@drawable/gradient"
, the icon get stretched:
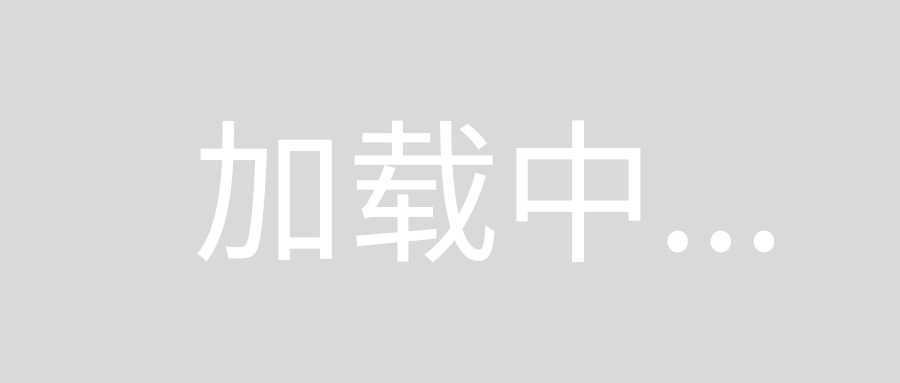
To deal with this I replaced my gradient.xml with the following code:
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="oval">
<gradient
android:angle="90"
android:startColor="#00FF00"
android:endColor="#000000" />
<size
android:width="56dp"
android:height="56dp" />
</shape>
</item>
<item
android:left="10dp"
android:right="10dp">
<shape android:shape="line">
<stroke
android:width="2dp"
android:color="#ffffff" />
</shape>
</item>
<item
android:left="10dp"
android:right="10dp">
<rotate
android:fromDegrees="90">
<shape android:shape="line">
<stroke
android:width="2dp"
android:color="#ffffff" />
</shape>
</rotate>
</item>
Output:
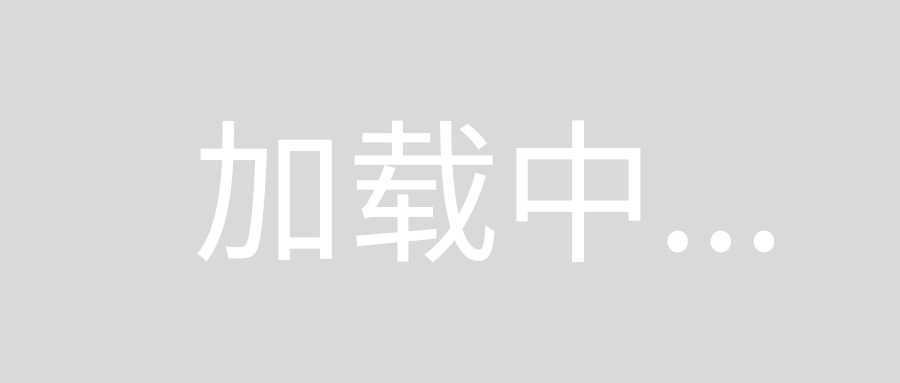