I'm trying to create a grid which will have multiple row and columns. I'd like them all to have the same height using flexbox, but the only thing I can get is columns of same size on one row.
Here is an example of what I'm trying to do: http://jsbin.com/maxavahesa/1/edit?html,css,output
In this example I'd like all my <li>
's to have the same height, which means the height of the biggest item (in my example, this would be the last <li>
). Is it possible to achieve with flexbox ?
No, this is not possible with pure CSS/flexbox.
I'll cite the W3C spec:
When a flex container has multiple lines, the cross size of each line is the minimum size necessary to contain the flex items on the line (after aligment due to align-self), and the lines are aligned within the flex container with the align-content property. [...]
(From http://www.w3.org/TR/css3-flexbox/#flex-lines)
So, one item is only expanded to the maximum height of that line it's currently on.
Terminology of the above quote:
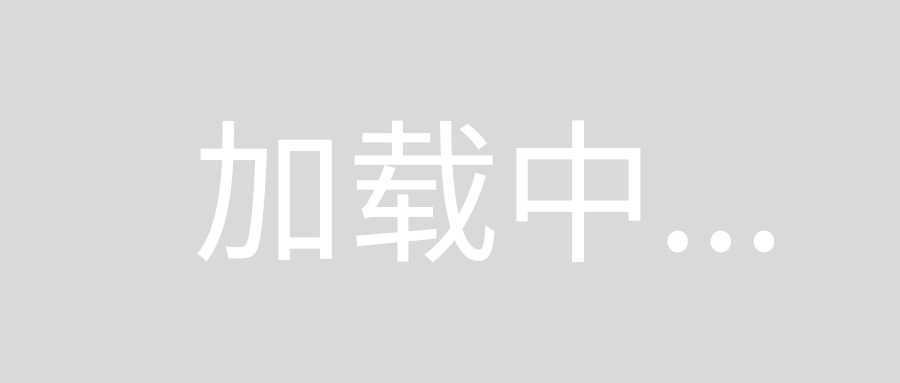
(From http://www.w3.org/TR/css3-flexbox/#box-model)
Although this topic is old, for anyone seeing this topic now, you can't achieve that with flexbox, like it's said in the accepted answer; however, you can achive that with Grid Layout:
* {
box-sizing: border-box;
}
ul {
margin: 0;
padding: 0;
font-size: 0;
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-auto-rows: 1fr;
grid-column-gap: 1px;
grid-row-gap: 1px;
}
li {
display: inline-block;
font-size: 16px;
background: grey;
border: 1px solid white;
}
<ul>
<li>a</li>
<li>b</li>
<li>c</li>
<li>d</li>
<li>e</li>
<li>f</li>
<li>g</li>
<li>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Eum et rerum labore repellat voluptatibus ut sit deserunt facilis dolorum exercitationem earum quibusdam quo itaque distinctio magnam, accusantium soluta voluptates aut.</li>
</ul>
Although this is not flexbox, it behaves in a very similar way and it's very easy to use.
This doesn't force the list items to have a specific display, so the items could be block
, flex
, inline-block
(like in the example above).
Just define the list (container) with:
display: grid
(will use the grid layout);
grid-template-columns: repeat(4, 1fr)
(assuming you want 4 columns per row);
grid-auto-rows: 1fr
(fr
is the flex factor, the value of 1 meaning the max size of all items in the list; more information in https://developer.mozilla.org/en-US/docs/Web/CSS/grid-auto-rows).