What is 'Greedy token parsing' in PHP ?
I found this in Codeigniter guideline:
"Always use single quoted strings unless you need variables parsed, and in cases where you do need variables parsed, use braces to prevent greedy token parsing."
"My string {$foo}"
An answer with good explanation will help.
Thanks !!
Greedy token parsing refers to something like this:
$fruit = "apple";
$amount = 3;
$string = "I have $amount $fruits";
Possible expected output: "I have 3 apples"
Actual output: "I have 3 "
Of course, this is a beginner mistake, but even experts make mistakes sometimes!
Personally, I don't like to interpolate variables at all, braces or not. I find my code much more readable like this:
$string = "I have ".$amount." ".$fruit."s";
Note that code editors have an easier job colour-coding this line, as shown here in Notepad++:
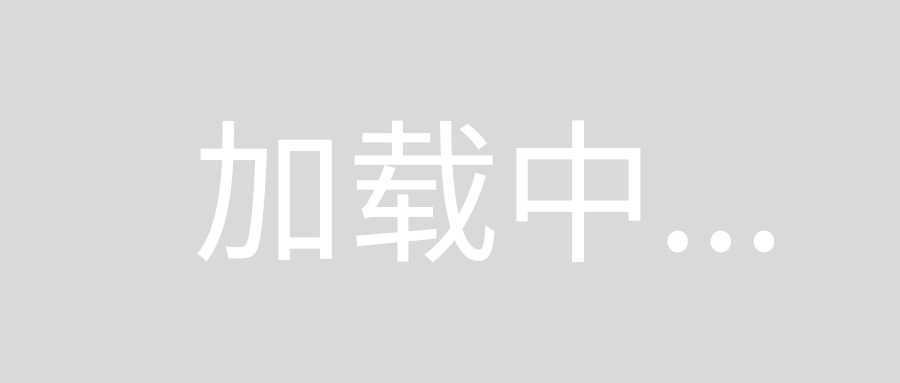
Then again, some people might prefer letting the engine do the interpolation:
$string = sprintf("I have %d %ss",$amount,$fruit);
It's all up to personal preference really, but the point made in the guideline you quoted is to be careful of what you are writing.
"Greedy" is a general term in parsing referring to "getting as much as you can". The opposite would be "ungreedy" or "get only as much as you need".
The difference in variable interpolation is, for example:
$foo = 'bar';
echo "$foos";
The parser here will greedily parse as much as makes sense and try to interpolate the variable "$foos
", instead of the actually existing variable "$foo
".
Another example in regular expressions:
preg_match('/.+\s/', 'foo bar baz')
This greedily grabs "foo bar
", because it's the longest string that fits the pattern .+\s
. On the other hand:
preg_match('/.+?\s/', 'foo bar baz')
This ungreedy +?
only grabs "foo
", which is the minimum needed to match the pattern.