I was wondering how to draw a circle around a point taken from a control's positioning.
This is not working as hoped
CGContextRef contextRef = UIGraphicsGetCurrentContext();
CGContextSetLineWidth(contextRef, 2.0);
CGContextSetRGBFillColor(contextRef, 0, 0, 1.0, 1.0);
CGContextSetRGBStrokeColor(contextRef, 0, 0, 1.0, 1.0);
CGRect circlePoint = (CGRectMake(self.frame.size.width / 2, self.frame.size.height / 2, 10.0, 10.0));
CGContextFillEllipseInRect(contextRef, circlePoint);
I think its pretty easy to draw a circle using UIBezierPath. All you need to do is subclass UIView and create UIBezierPath. I have created a example using UIBezierPath :
Create a subclass of UIView called CirecleView. Add following code :
#import "CircleView.h"
@implementation CircleView
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
// Initialization code
}
return self;
}
- (void)drawRect:(CGRect)rect
{
CGFloat rectX = self.frame.size.width / 2;
CGFloat rectY = self.frame.size.height / 2;
CGFloat width = 100;
CGFloat height = 100;
CGFloat centerX = rectX - width/2;
CGFloat centerY = rectY - height/2;
UIBezierPath *bezierPath = [UIBezierPath bezierPathWithOvalInRect:CGRectMake(centerX, centerY, width, height)];
[[UIColor blackColor] set];
[bezierPath stroke];
}
@end
Change your controller's view class to CircleView. Please see below picture.
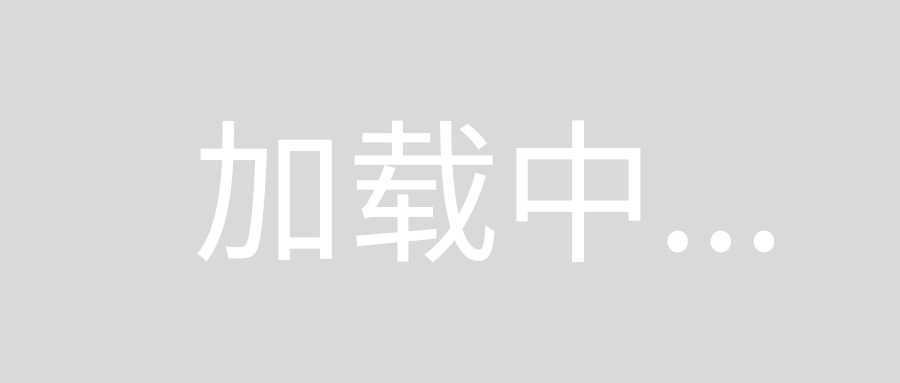
Thats it. Run your code to present your controller's view. Following is the output :
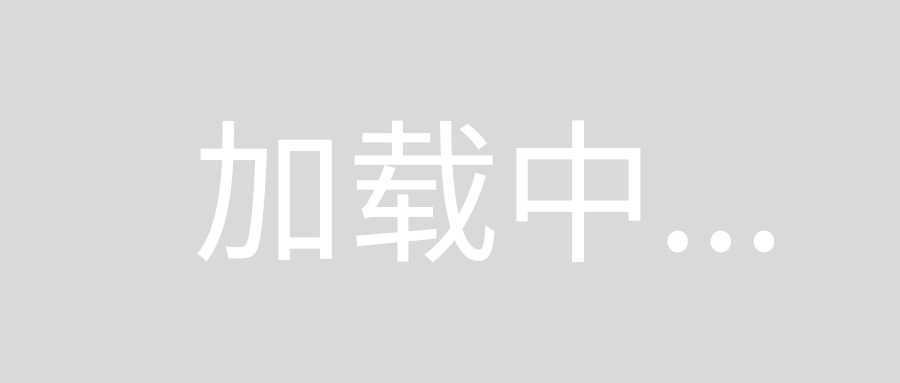
You can down code example from here
You just need to translate to the point first
cgContextRef.translateBy(x:circlePoint.x, y:circlePoint.y)
Note this is Swift 3, it may be different in other languages, just search "translate cgcontext"