On the picture bellow is what I'm trying to build:
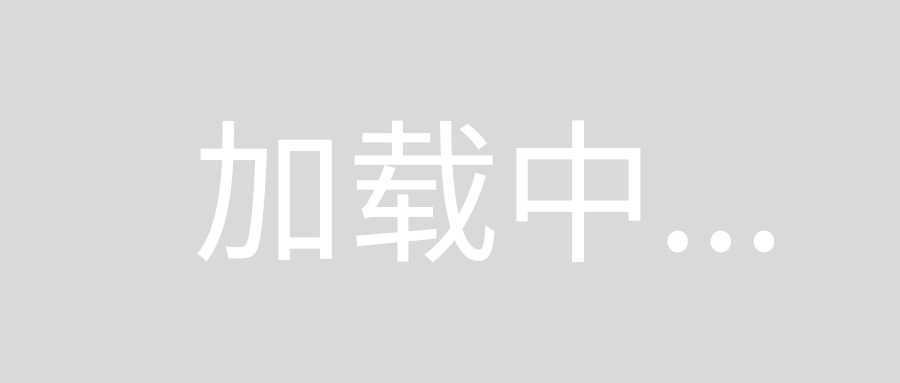
Idea is that all the boxes are hidden until whole page loads. Then they should have set random positions around browser view port and then they (boxes) needs to be animated to move to their proper positions.
Boxes have absolute positions in CSS, and my idea is when windows loads to put initial position in variable, then randomize position of the box, and then finally move it to the starting position. Hope I'm clear :)
Here is my current setup: http://jsfiddle.net/HgMB4/
You will notice that I'm missing code on window load, because I'm not sure how to animate boxes to their initial position.
$(window).load(function(){
//Huh! What to write here to animate boxes to their initial positions set by CSS?
});
Try this
$(document).ready(function(){
var h = $(window).height();
var w = $(window).width();
$('#intro .box').each(function(){
var originalOffset = $(this).position(),
$this = $(this),
tLeft = w-Math.floor(Math.random()*900),
tTop = h-Math.floor(Math.random()*900);
$(this).animate({"left": tLeft , "top": tTop},5000,function(){
$this.animate({
"left": originalOffset.left,
"top": originalOffset.top
},5000);
});
});
});
This will animate the boxes to random position and then will move them back to their original position.
Here is the working demo http://jsfiddle.net/HgMB4/18
If you want to animate boxes only when they move to initial position then try this
$(document).ready(function(){
var h = $(window).height();
var w = $(window).width();
$('#intro .box').each(function(){
var originalOffset = $(this).position(),
$this = $(this),
tLeft = w-Math.floor(Math.random()*900),
tTop = h-Math.floor(Math.random()*900);
$(this).css({
"left": tLeft,
"top": tTop
});
$this.animate({ "left": originalOffset.left,
"top": originalOffset.top
},5000);
});
});
Here is the working demo http://jsfiddle.net/HgMB4/22
Requirements
- boxes are hidden until whole page loads
- boxes are set to random positions around browser view port
- boxes need to animate to their proper positions
You can store the original position and after your random placement start animating them to go back to their starting position. I also cached any DOM reference which were repeated to speed up the code execution (most likely not noticeably but it's good practice)
DEMO - Boxes placed at random and then animated back to their proper positions.
I have separated the 2 actions, placing the boxes at random locations using your original code but now also storing the original position in associated data using jQuery's Data which is then used to animate them back to their proper positions.
You can now randomise and re-set the boxes on demand by calling either method:
$(document).ready(function() {
var h = $(window).height();
var w = $(window).width();
var $allBoxes = $('#intro .box');
placeBoxesRandomly($allBoxes, h, w);
placeBoxesToDataStorePositions($allBoxes);
});
placeBoxesRandomly
still does what it did before except it now also associates the original starting position data with the elements:
function placeBoxesRandomly($boxes, h, w) {
$boxes.each(function() {
var $box = $(this);
var position = $box.position();
tLeft = w - Math.floor(Math.random() * 900), tTop = h - Math.floor(Math.random() * 900);
$box.css({
"left": tLeft,
"top": tTop
});
$box.data("start-position-left", position.left);
$box.data("start-position-top", position.top);
});
}
placeBoxesToDataStorePositions
places the boxes to the positions stored in the associated data.
function placeBoxesToDataStorePositions($boxes) {
$boxes.each(function() {
var $box = $(this);
$box.animate({
"left": $box.data("start-position-left"),
"top": $box.data("start-position-top")
}, 3000);
});
}
I think you are looking for jQuery Isotope plugin. This Stack-exchange page his this implented with a variation of it