I'm using PHP guzzle
I have tried
public static function get($url) {
$client = new Client();
try {
$res = $client->request('GET',$url);
$result = (string) $res->getBody();
$result = json_decode($result, true);
return $result;
}
catch (GuzzleHttp\Exception\ClientException $e) {
$response = $e->getResponse();
$responseBodyAsString = $response->getBody()->getContents();
}
}
I kept getting
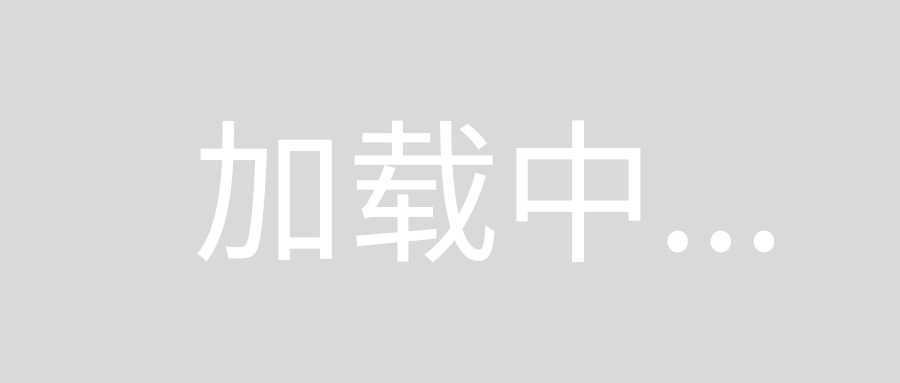
How to prevent crashing when Guzzle detecct 400 or 500 error ?
I just want my application to continue running and loading.
So, I'd bet your get()
function exists in a namespace like App\Http\Controllers
, which means this:
catch (GuzzleHttp\Exception\ClientException $e) {
is actually being interpreted as if you'd written:
catch (App\Http\Controllers\GuzzleHttp\Exception\ClientException $e) {
For obvious reasons, no exception of that kind is being thrown.
You can fix the namespacing issue by doing:
catch (\GuzzleHttp\Exception\ClientException $e) {
(note the leading \
) or putting:
use GuzzleHttp\Exception\ClientException;
at the top of the file after the namespace
declaration and catching just ClientException
.
See http://php.net/manual/en/language.namespaces.basics.php.
I would try something like this:
public static function get($url) {
try {
$client = new Client();
$res = $client->request('GET',$url);
$result = (string) $res->getBody();
$result = json_decode($result, true);
return $result;
}
catch (\Exception $e) {
if($e instanceof \GuzzleHttp\Exception\ClientException ){
$response = $e->getResponse();
$responseBodyAsString = $response->getBody()->getContents();
}
report($e);
return false;
}
}
The report()
helper function allows you to quickly report an exception using your exception handler's report method without rendering an error page.
Also take a look at http_errors
option to disable exceptions at all (if for your application it's an expected scenario, and you want to handle all responses specifically by yourself).
Instead of using just ClientException
You can try RequestException, which will help to handle bad requests.
try {
// Your code here.
} catch (GuzzleHttp\Exception\RequestException $e) {
if ($e->hasResponse()) {
// Get response body
// Modify message as proper response
$message = $e->getResponse()->getBody();
return (string) $exception;
}
else {
return $e->getMessage();
}
}
You can catch any exception with this :
catch (\Exception $e)