I want to a draw transparent image on Delphi form, but it is not working.
Here is original PNG:
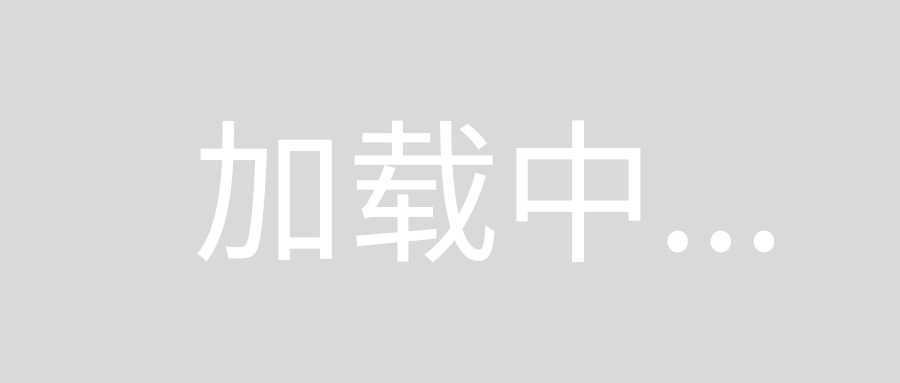
I have loaded image in a TImage::
Image1.Transparent := True;
Form1.Color := clWhite;
Form1.TransparentColor := True;
Form1.TransparentColorValue := clWhite;
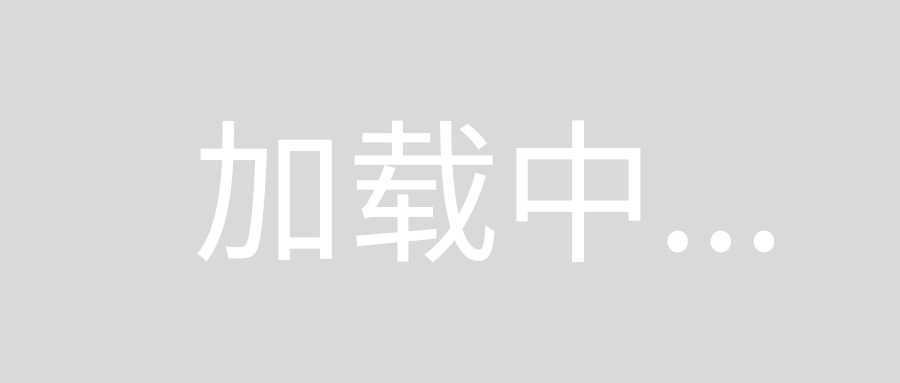
The application:
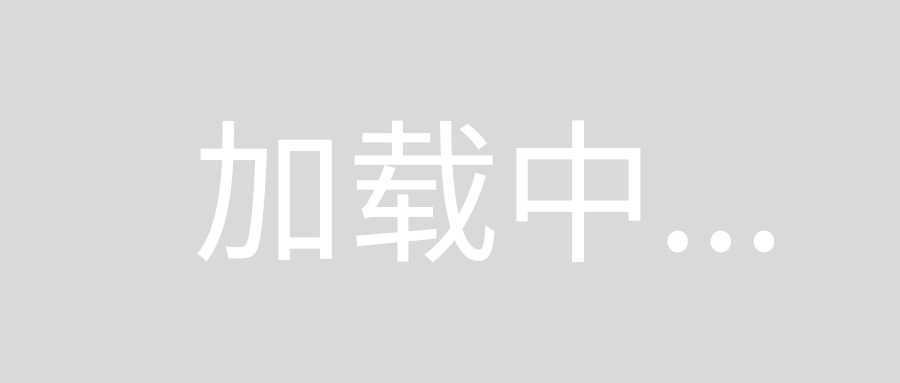
The image isnt fully transparent.
The image can be drawed by any control or just by canvas.
I would like to use BMP images.
Maybe I am doing something wrong?
Please help!
I found a solution that will let you draw a BMP image with an alpha channel onto a form using only the Windows API:
const
AC_SRC_OVER = 0;
AC_SRC_ALPHA = 1;
type
BLENDFUNCTION = packed record
BlendOp,
BlendFlags,
SourceConstantAlpha,
AlphaFormat: byte;
end;
function WinAlphaBlend(hdcDest: HDC; xoriginDest, yoriginDest, wDest, hDest: integer;
hdcSrc: HDC; xoriginSrc, yoriginSrc, wSrc, hSrc: integer; ftn: BLENDFUNCTION): LongBool;
stdcall; external 'Msimg32.dll' name 'AlphaBlend';
procedure TForm4.FormClick(Sender: TObject);
var
hbm: HBITMAP;
bm: BITMAP;
bf: BLENDFUNCTION;
dc: HDC;
begin
hbm := LoadImage(0,
'C:\Users\Andreas Rejbrand\Skrivbord\RatingCtrl.bmp',
IMAGE_BITMAP,
0,
0,
LR_LOADFROMFILE);
if hbm = 0 then
RaiseLastOSError;
try
if GetObject(hbm, sizeof(bm), @bm) = 0 then RaiseLastOSError;
dc := CreateCompatibleDC(0);
if dc = 0 then RaiseLastOSError;
try
if SelectObject(dc, hbm) = 0 then RaiseLastOSError;
bf.BlendOp := AC_SRC_OVER;
bf.BlendFlags := 0;
bf.SourceConstantAlpha := 255;
bf.AlphaFormat := AC_SRC_ALPHA;
if not WinAlphaBlend(Canvas.Handle,
10,
10,
bm.bmWidth,
bm.bmHeight,
dc,
0,
0,
bm.bmWidth,
bm.bmHeight,
bf) then RaiseLastOSError;
finally
DeleteDC(dc);
end;
finally
DeleteObject(hbm);
end;
end;
Using The GIMP, I converted the PNG image
http://privat.rejbrand.se/RatingCtrl.png
found here to a 32-bit RGBA bitmap, found here, and the result is very good:
http://privat.rejbrand.se/gdiblend1.png
http://privat.rejbrand.se/gdiblend2.png
http://privat.rejbrand.se/gdiblend3.png
The TransparentColorValue
approach cannot possibly work, because this only works with images in which a single colour represents full transparency. [In addition, you are toying with the form's transparent colour instead of image's transparent colour!] The above PNG image is supposed to have an alpha channel, so it's not like every pixel is either shown or transparent -- instead, each pixel has an opacity between 0 and 1 (0.37, for instance). That is, in addition to the R, G, and B components of each pixel, there is an 'alpha' component A.
The above image appears to be corrupt, however. A 'correct' PNG is shown below:
http://privat.rejbrand.se/RatingCtrl.png
You can try to blend the above one onto different backgrounds, and you will find that the shadow blends nicely.
So, if one has a 'correct' PNG, how to draw it onto a form? Well, that is going to be very difficult in your case, since Delphi 7 does not support PNG images. It only supports BMP images, and these normally do not have alpha channels.
Why not try do draw your png onto new image with regular bmp. Draw what you want onto image 2 and redraw /or assign/ all to your image 1 when finish. Must works...