I can't add few values to the same field. I can select only one value, and after I input ,
, ;
or other delimiter character, I can't select another one. I want it to work similar to autocomplete.
I have a textbox with jQuery bound:
<div class="editor-field">
@Html.EditorFor(model => model.Name) @Html.ValidationMessageFor(model => model.Name)
</div>
<script type="text/javascript">
$(document).ready(function () {
$("#Name").autocomplete('@Url.Action("TagName", "Tag")', {
minChars: 1,
delimiter: /(,|;)\s*/,
onSelect: function(value, data){
alert('You selected: ' + value + ', ' + data);
}
});
});
</script>
It uses data from my controller:
public ActionResult TagName(string q)
{
var tags = new List<TagModel>
{
new TagModel {Name = "aaaa", NumberOfUse = "0"},
new TagModel {Name = "mkoh", NumberOfUse = "1"},
new TagModel {Name = "asdf", NumberOfUse = "2"},
new TagModel {Name = "zxcv", NumberOfUse = "3"},
new TagModel {Name = "qwer", NumberOfUse = "4"},
new TagModel {Name = "tyui", NumberOfUse = "5"},
new TagModel {Name = "asdf[", NumberOfUse = "6"},
new TagModel {Name = "mnbv", NumberOfUse = "7"}
};
var tagNames = (from p in tags where p.Name.Contains(q) select p.Name).Distinct().Take(3);
string content = string.Join<string>("\n", tagNames);
return Content(content);
}
I'm using these scripts:
<link href="@Url.Content("~/Content/Site.css")" rel="stylesheet" type="text/css" />
<script src="@Url.Content("~/Scripts/jquery-1.5.1.min.js")" type="text/javascript"></script>
<script src="@Url.Content("~/Scripts/jquery.autocomplete.js")" type="text/javascript"></script>
<link href="@Url.Content("~/Scripts/jquery.autocomplete.css")" rel="stylesheet" type="text/css" />
There is no error in firebug. What is wrong with my code?
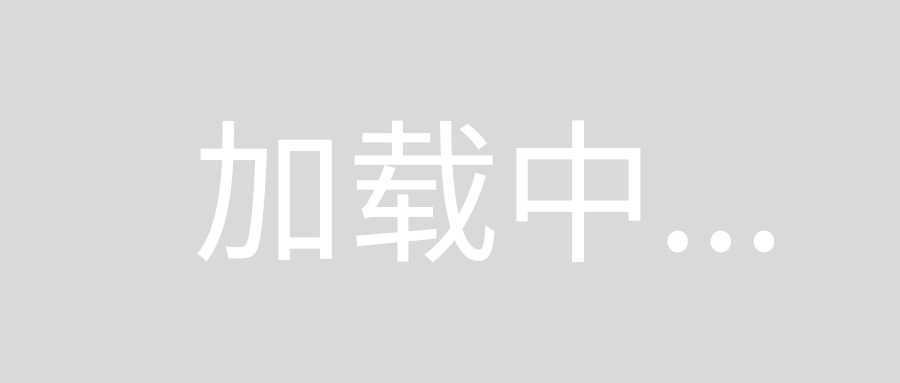
Experienced this kind of problems with firebug.
I suggest to not trust Firebug console
until it contains error messages
If your code is not working as expected, and firebug is not showing you any error messages then it's time to check your web page in chrome and see where exactly the exception is not handled within Console tab, specially when you're using ajax.
I would recommend you using the more recent jQuery UI autocomplete plugin. The jQuery ui 1.8 is even distributed as part of new ASP.NET MVC 3 projects.
As far as your code is concerned try to fix it like this:
var url = '@Url.Action("TagName", "Tag")';
$('#Name').autocomplete(url, {
minChars: 1,
multiple: true,
formatResult: function(row) {
return row[0].replace(/(<.+?>)/gi, '');
}
}).result(function (event, data, formatted) {
alert(!data ? "No match!" : "Selected: " + formatted);
});
The autocomplete function that is included with jQuery UI has a different code format than the stand-alone jQuery plugin. Details on this can be found at the jQuery UI website at the link below.
http://jqueryui.com/demos/autocomplete/
Here is a simple example of the jQuery UI Autocomplete function
$( "#Name" ).autocomplete({
source: url,
minLength: 1,
select: function( event, ui ) {
log( ui.item ?
"Selected: " + ui.item.value + " aka " + ui.item.id :
"Nothing selected, input was " + this.value );
}
});
What version of autocomplete are you using, the jquery ui one does not support this. See http://jqueryui.com/demos/autocomplete/#multiple for details.
This is a snippit from the page to set up multi selections
.autocomplete({
minLength: 0,
source: function( request, response ) {
// delegate back to autocomplete, but extract the last term
response( $.ui.autocomplete.filter(
availableTags, extractLast( request.term ) ) );
},
focus: function() {
// prevent value inserted on focus
return false;
},
select: function( event, ui ) {
var terms = split( this.value );
// remove the current input
terms.pop();
// add the selected item
terms.push( ui.item.value );
// add placeholder to get the comma-and-space at the end
terms.push( "" );
this.value = terms.join( ", " );
return false;
}
});