I want to read and edit(Write) the mp4 metadata. Particularly I want to read/write Tag metadata in android as shown in below picture.
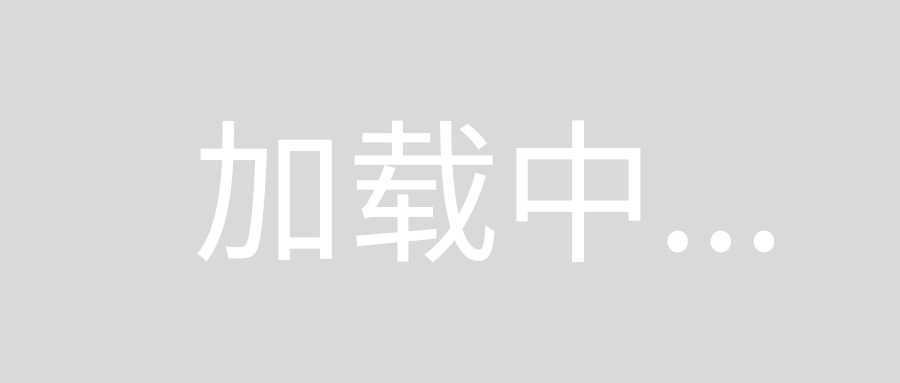
I searched for this on internet and found mp4Parser, but I think mp4Parser don't write Title Keyword.
For .jpg file we use XPKeyword metadata which represents title. So same way how to do same for .mp4 file.
The Android SDK contains the class MediaMetadataRetriever to retrieve all the metadata values inside your file, you can read more about MetaData values here.
public void readMetaData() {
File sdcard = Environment.getExternalStorageDirectory();
File file = new File(sdcard,"/Android/data/myfile.mp4");
if (file.exists()) {
Log.i(TAG, ".mp4 file Exist");
//Added in API level 10
MediaMetadataRetriever retriever = new MediaMetadataRetriever();
try {
retriever.setDataSource(file.getAbsolutePath());
for (int i = 0; i < 1000; i++){
//only Metadata != null is printed!
if(retriever.extractMetadata(i)!=null) {
Log.i(TAG, "Metadata :: " + retriever.extractMetadata(i));
}
}
} catch (Exception e) {
Log.e(TAG, "Exception : " + e.getMessage());
}
} else {
Log.e(TAG, ".mp4 file doesn´t exist.");
}
}
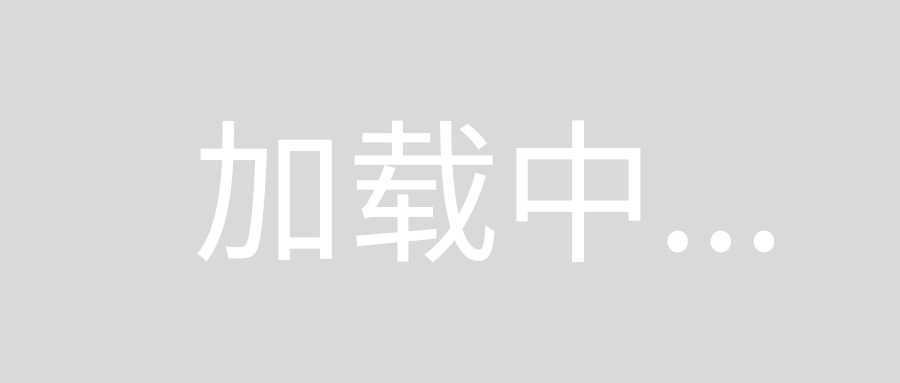
To edit/write metadata, Android SDK doesn´t have any method, probably by copyright issues, but you can use options like:
https://github.com/sannies/mp4parser
http://multimedia.cx/eggs/supplying-ffmpeg-with-metadata/
https://github.com/bytedeco/javacv/blob/master/src/main/java/org/bytedeco/javacv/FFmpegFrameRecorder.java
I hope it is not too late but if you want to add/edit/delete metadata fields on MP4 files you can use JCodec's metadata editing classes.
There's a CLI tool backed by a java API. The CLI is in org.jcodec.movtool.MetadataEditorMain and the API is in org.jcodec.movtool.MetadataEditor.
Read more about it: http://jcodec.org/docs/working_with_mp4_metadata.html
So basically when you want to add some metadata you need to know what key(s) it corresponds to. One way to find out is to inspect a sample file that already has the metadata you need. For this you can run the JCodec's CLI tool that will just print out all the existing metadata fields (keys with values):
./metaedit <file.mp4>
Then when you know the key you want to work with you can either use the same CLI tool:
# Changes the author of the movie
./metaedit -f -si ©ART=New\ value file.mov
or the same thing via the Java API:
MetadataEditor mediaMeta = MetadataEditor.createFrom(new
File("file.mp4"));
Map<Integer, MetaValue> meta = mediaMeta.getItunesMeta();
meta.put(0xa9415254, MetaValue.createString("New value")); // fourcc for '©ART'
mediaMeta.save(false); // fast mode is off
To delete a metadata field from a file:
MetadataEditor mediaMeta = MetadataEditor.createFrom(new
File("file.mp4"));
Map<Integer, MetaValue> meta = mediaMeta.getItunesMeta();
meta.remove(0xa9415254); // removes the '©ART'
mediaMeta.save(false); // fast mode is off
To convert string to integer fourcc you can use something like:
byte[] bytes = "©ART".getBytes("iso8859-1");
int fourcc =
ByteBuffer.wrap(bytes).order(ByteOrder.BIG_ENDIAN).getInt();
If you want to edit/delete the android metadata you'll need to use a different set of fucntion (because it's stored differently than iTunes metadata):
./metaedit -sk com.android.capture.fps,float=25.0 file.mp4
OR alternatively the same through the API:
MetadataEditor mediaMeta = MetadataEditor.createFrom(new
File("file.mp4"));
Map<String, MetaValue> meta = mediaMeta.getKeyedMeta();
meta.put("com.android.capture.fps", MetaValue.createFloat(25.));
mediaMeta.save(false); // fast mode is off