I'm using Swift language for ADMOB whenever new advertisement comes up, my Memory is increasing. I think there is a Leaking. Without ADMOB everything else is fine.
var inter: GADInterstitial
override func viewWillAppear(animated: Bool) {
inter = GADInterstitial()
inter.delegate = self
inter.adUnitID = "****"
var request:GADRequest = GADRequest()
request.testDevices = [ "***" ]
inter.loadRequest(request)
}
And I'm using UIActionAlert for displaying the Interstitial
self.inter.presentFromRootViewController(self)
Memory Report Link:
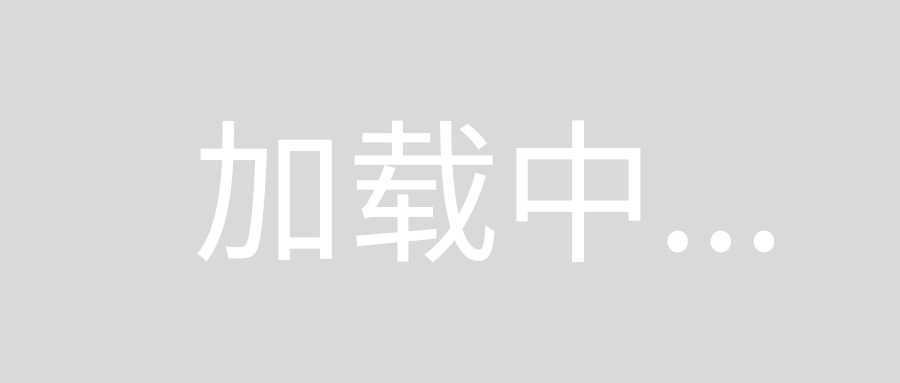
Am I doing something wrong? I'm using ARC. Can I force to release this Interstitials
by myself.
EDİT:
I tried GADBanner
too. I'm just opening the app; I'm not doing anything else and memory is increasing
override func viewWillAppear(animated: Bool) {
banner = GADBannerView()
banner.delegate = self
banner.adSize = kGADAdSizeSmartBannerPortrait
banner.adUnitID = "****"
var request:GADRequest = GADRequest()
banner.rootViewController = self
request.testDevices = [ "****" ]
self.view.addSubview(banner)
}
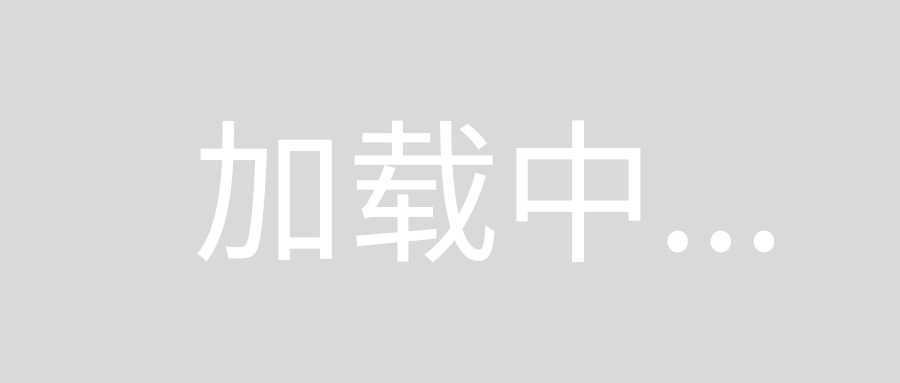
You need to call destroy()
to avoid memory leaks on both banner and interstitial ads. Interstitial ads are one-time-use objects, so you would have to destroy them. Banner ads can be reused but once done using them, call destroy()
.
See this for reference.
I think you need to release the banner by setting delegate to nil.
From AdMob documentation (see https://developers.google.com/mobile-ads-sdk/docs/admob/ios/banner?hl=es):
Note that if you implement your delegate as a distinct object rather
than a GADBannerView subclass you should be sure to set the
GADBannerView's' delegate property to nil before releasing the view.
- (void)dealloc {
bannerView_.delegate = nil;
// Don't release the bannerView_ if you are using ARC in your project
[bannerView_ release];
[super dealloc];
}
In your case I think you only need to implement the bannerView_.delegate = nil
call.
I hope this helps.
I found that the best practice is to allocate the GADBannerView only once, otherwise it will pile up in your memory.
My solution is to store my GADBannerView in a singleton class, and allocate it when the app's rootViewController loads (didLoad). Then you can use it anywhere without requesting again.
I had the same issue, although with GADInterstitial AdMob ads. HUGE CPU churn from the memory leak. The problem is that you have to go to your actual root view controller. I'm in Objective C, but basically, if you're in, say, a UITabBarController view hierarchy, then try this:
banner.rootViewController = (UITabBarController *)self.view.window.rootViewController
That one thing solved all of my issues. Hope it works!