可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I'm trying to setup a basic swagger API doc in a new asp .net CORE / MVC 6 project and receiving a 500 error from the swagger UI:
500 : http://localhost:4405/swagger/v1/swagger.json
My startup class has the following code in it:
using Swashbuckle.SwaggerGen;
using Swashbuckle.SwaggerGen.XmlComments;
using Swashbuckle.Application;
....
public void ConfigureServices(IServiceCollection services)
{
...
services.AddSwaggerGen();
services.ConfigureSwaggerDocument(options =>
{
options.SingleApiVersion(new Info
{
Version = "v1",
Title = "Blog Test Api",
Description = "A test API for this blogpost"
});
});
}
and then under Configure:
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory)
{
....
app.UseSwaggerGen();
app.UseSwaggerUi();
....
}
When i build and run the project, the UI will come up when i go to swagger/UI/index.html, but the 500 error above is displayed. When i go to the swagger/v1/swagger.json link, console gives the following 500 error:
Failed to load resource: the server responded with a status of 500 (Internal Server Error)
Is there any way i can figure out the root cause of the 500 or enable any additional debug in swagger to figure out why it's throwing this error? Based on some of the tutorials i've looked at, only what i have in startup is required for a base implementation. Please let me know if i can provide any additional information.
EDIT: this is for rc1, and may not be relevant to the new netcore 1.0 currently out (6/29/2016)
回答1:
Initially I got a 500 error too. Deep down in the stacktrace it said:
System.NotSupportedException: Unbounded HTTP verbs for path 'api/hotels'. Are you missing an HttpMethodAttribute?
It turned out I was missing a HttpGet attribute for one of my api methods:
[Microsoft.AspNetCore.Mvc.HttpGet]
回答2:
If someone want to know the exact error is in the Swagger's stack trace, request the URL:
<your-app-url>/swagger/v1/swagger.json
Or, click on the swagger.json
link from the browser dev tools console:
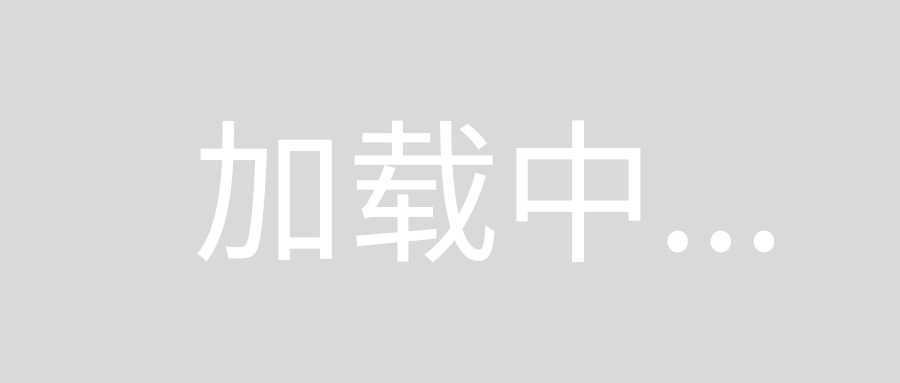
回答3:
Firstly you can enable de developer exception page by adding app.UseDeveloperExceptionPage();
on your Configure() in order to see better which is the root cause. Take a look here
In my case the problem was that I have to install also Microsoft.AspNetCore.StaticFiles
nuget in order to make Swagger work.
Try also to uninstall/reinstall Swashbuckle.AspNetCore
nuget.
回答4:
I got this error when one of my functions was marked as public
, but wasn't meant to be a web service which could be called directly.
Changing the function to private
made the error go away.
Alternatively, immediately before your public
function, you can put the [NonAction]
command, to tell Swagger to ignore it.
[NonAction]
public async Task<IActionResult> SomeEvent(string id)
{
...
}
(I wish Swagger would actually report the name of the function which caused this problem though, rather than just complaining that it could no longer find the "../swagger/v1/swagger.json" file... that's not particularly useful.)
回答5:
Might be obvious but, besides missing the HttpGet
or HttpPost
attributes, don't forget to differentiate the post methods.
You may have 2 different methods (with different names) marked with HttpPost
, and that would also cause this kind of issue. Remember to specify the method name in the attribute: [HttpPost("update")]
.
回答6:
Also if I may add, the swagger set up does not like it when you route at the root level of your controllers. For example:
Do not do this:
[Produces("application/json")]
[Route("/v1/myController")]
[Authorize]
public class myController
{
[SwaggerResponse((int)System.Net.HttpStatusCode.OK, Type = typeof(RestOkResponse<Response>))]
[SwaggerResponse((int)System.Net.HttpStatusCode.InternalServerError, Type = typeof(RestErrorResponse))]
[SwaggerResponse((int)System.Net.HttpStatusCode.BadRequest, Type = typeof(RestErrorResponse))]
[SwaggerResponse((int)System.Net.HttpStatusCode.Forbidden, Type = typeof(RestErrorResponse))]
[SwaggerResponse((int)System.Net.HttpStatusCode.NotFound)]
[HttpPost]
[Authorize()]
public async Task<IActionResult> Create([FromBody] MyObject myObject)
{
return Ok();
}
}
Do this:
[Produces("application/json")]
[Authorize]
public class myController
{
[SwaggerResponse((int)System.Net.HttpStatusCode.OK, Type = typeof(RestOkResponse<Response>))]
[SwaggerResponse((int)System.Net.HttpStatusCode.InternalServerError, Type = typeof(RestErrorResponse))]
[SwaggerResponse((int)System.Net.HttpStatusCode.BadRequest, Type = typeof(RestErrorResponse))]
[SwaggerResponse((int)System.Net.HttpStatusCode.Forbidden, Type = typeof(RestErrorResponse))]
[SwaggerResponse((int)System.Net.HttpStatusCode.NotFound)]
[HttpPost("/v1/myController")]
[Authorize()]
public async Task<IActionResult> Create([FromBody] MyObject myObject)
{
return Ok();
}
}
It took me a while to figure that the reason why I was getting internal server error was because of this routing issue. Hope this helps someone!
回答7:
When I Add the parameter Version , it works
services.AddSwaggerGen(options =>
{
options.SwaggerDoc("v1", new Info { Title = "My API", Version = "v1" });
});
回答8:
To see the source of exception
- open chrome browser
- open developer tools
- see exceptions in console tab
- fix it.
回答9:
Also had this problem. In my case, it was caused by two endpoints in the same controller with the same route and method name (but different parameter types). Of course, it then became apparent that that was probably poor practice anyway so I changed the endpoint names and all was well.
回答10:
in some cases, the router of controller is duplicated. Review the last controller modified.
回答11:
I was getting this error because in STARTUP.CS I not put the version's name in SwaggerDoc parameters:
Error => c.SwaggerDoc("", blablabla
WORK => c.SwaggerDoc("v1",blablabla
then, now are ok fine!
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new Swashbuckle.AspNetCore.Swagger.Info {Title = "PME SERVICES", Version = "v1"});
});
回答12:
I ran into this issue today configuring Swagger in a .Net Core 2.2 Web Api project. I started down the path that @Popa Andrei mentions above by including the Microsoft.AspNetCore.StaticFiles
dependency in my project as I figured that was most likely the culprit. That turned into a rabbit hole of chaining dependencies although it did ultimately work for me.
I then realized that in my ConfigureServices
method in Startup
I had services.AddMvcCore(...)
which just gives you bare bones and you add dependencies as you need them. When I changed that to services.AddMvc(...)
it started working without having to manually add all the dependencies required by Microsoft.AspNetCore.StaticFiles
.
That doesn't mean you can't take the route of staying with services.AddMvcCore(...)
and then adding all the necessary dependencies. You can, and it will work.
It is just much easier to take the services.AddMvc(...)
approach and be done.
Hope that helps someone.
回答13:
Making sure my swagger versions lined up with each other fixed my issue. Since I was starting a new project I set my api version to be v0.1
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v0.1", new Info { Title = "Tinroll API", Version = "v0.1" });
});
But had left my swagger url to be v1.
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "Tinroll API v0.1");
c.RoutePrefix = string.Empty;
});
I updated my versioning to be /swagger/v0.1/swagger.json
instead of v1
and Swagger worked as expected.
回答14:
Since I don't see the solution which worked for me posted here, I will contribute one to the ongoing thread. In my case, it was the Route attribute was set separately with the HttpPost/HttpGet at the function level (not controller level).
INCORRECT:
[HttpPost]
[Route("RequestItem/{itemId}")]
CORRECT:
[HttpPost("RequestItem/{itemId}")]
Also, the Swagger seems to expect Ok(object) result instead of StatusCode(object) result for a success request to return.
回答15:
Give a look at this project.
https://github.com/domaindrivendev/Ahoy/tree/master/test/WebSites/Basic
This repo is from Swashbuckle´s owner, is a basic ASP.NET 5 Sample app, this is help you to correct configure yours middlewares (and take care about the orders of them, it´s matter, e.g., use "app.UseSwaggerGen();app.UseSwaggerUi(); after app.UseMvc();)
To enable logging in your applcation give a look at:
https://docs.asp.net/en/latest/fundamentals/logging.html?highlight=logging
(the log will be generated inside "wwwroot" folder
回答16:
The setup for Swagger is varying greatly from version to version. This answer is for Swashbuckle 6.0.0-beta9 and Asp.Net Core 1.0. Inside of the ConfigureServices method of Startup.cs, you need to add -
services.AddSwaggerGen(c =>
{
c.SingleApiVersion(new Info
{
Version = "v1",
Title = "My Awesome Api",
Description = "A sample API for prototyping.",
TermsOfService = "Some terms ..."
});
});
Then in the Configure method you must add -
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory)
{
loggerFactory.AddConsole(Configuration.GetSection("Logging"));
loggerFactory.AddDebug();
app.UseMvc();
app.UseSwaggerGen();
app.UseSwaggerUi();
}
Be sure you are referencing in Startup.cs -
using Swashbuckle.SwaggerGen.Generator;
My project.json file looks like -
"dependencies": {
"Microsoft.AspNetCore.Mvc": "1.0.0-rc2-final",
"Microsoft.AspNetCore.Server.IISIntegration": "1.0.0-rc2-final",
"Microsoft.AspNetCore.Server.Kestrel": "1.0.0-rc2-final",
"Microsoft.EntityFrameworkCore.SqlServer": "1.0.0-rc2-final",
"Microsoft.EntityFrameworkCore.SqlServer.Design": "1.0.0-rc2-final",
"Microsoft.EntityFrameworkCore.Tools": "1.0.0-*",
"Microsoft.Extensions.Configuration.EnvironmentVariables": "1.0.0-rc2-final",
"Microsoft.Extensions.Configuration.FileExtensions": "1.0.0-rc2-final",
"Microsoft.Extensions.Configuration.Json": "1.0.0-rc2-final",
"Microsoft.Extensions.Logging": "1.0.0-rc2-final",
"Microsoft.Extensions.Logging.Console": "1.0.0-rc2-final",
"Microsoft.Extensions.Logging.Debug": "1.0.0-rc2-final",
"Swashbuckle": "6.0.0-beta9"
},
"tools": {
"Microsoft.AspNetCore.Server.IISIntegration.Tools": {
"version": "1.0.0-preview1-final",
"imports": "portable-net45+win8+dnxcore50"
},
"Microsoft.EntityFrameworkCore.Tools": {
"version": "1.0.0-preview1-final",
"imports": [
"portable-net45+win8+dnxcore50",
"portable-net45+win8"
]
}
},
"frameworks": {
"net452": { }
},
"buildOptions": {
"emitEntryPoint": true,
"preserveCompilationContext": true,
"xmlDoc": false
},
"publishOptions": {
"include": [
"wwwroot",
"Views",
"appsettings.json",
"web.config"
]
},
"scripts": {
"postpublish": [ "dotnet publish-iis --publish-folder %publish:OutputPath% --framework %publish:FullTargetFramework%" ]
}
}