I'm using CAGradientLayer
on my image. I use this code to do this:
UIImage *image = [UIImage imageNamed:@"perfect.jpg"];
[testImage setImage:image];
CAGradientLayer *myLayer = [CAGradientLayer layer];
myLayer.anchorPoint = CGPointZero;
//starts in bottom left
myLayer.startPoint = CGPointMake(1.0f,1.0f);
//ends in top right
myLayer.endPoint = CGPointMake(1.0f, 0.0f);
UIColor *outerColor = [UIColor colorWithWhite:1.0 alpha:0.0];
UIColor *innerColor = [UIColor colorWithWhite:1.0 alpha:1.0];
//an array of colors that dictatates the gradient(s)
myLayer.colors = @[(id)outerColor.CGColor, (id)outerColor.CGColor, (id)innerColor.CGColor, (id)innerColor.CGColor];
//these are percentage points along the line defined by our startPoint and endPoint and correspond to our colors array. The gradient will shift between the colors between these percentage points.
myLayer.locations = @[@0.0, @0.15, @0.5, @1.0f];
myLayer.bounds = CGRectMake(0, 0, CGRectGetWidth(testImage.bounds), CGRectGetHeight(testImage.bounds));
testImage.layer.mask = myLayer;
[self.view addSubview:testImage];
But what I want is a Radial gradient or circle gradient effect. I'm only getting a gradient on one side. How can I achieve a circular gradient layer? Thanks.
If you want a circular gradient layer you will have to subclass and override the - (void)drawInContext:(CGContextRef)ctx
method. In that the code is pretty straight forward and should looks like to something like this for a radial gradient:
CGColorSpaceRef colorSpace = CGColorSpaceCreateDeviceRGB();
NSArray* colors = @[(id)[UIColor whiteColor].CGColor, (id)[UIColor blackColor].CGColor];
CGFloat locations[] = {.25, .75};
CGGradientRef gradient = CGGradientCreateWithColors(colorSpace, (CFArrayRef)colors, locations);
CGPoint center = (CGPoint){self.bounds.size.width / 2, self.bounds.size.height / 2};
CGContextDrawRadialGradient(ctx, gradient, center, 20, center, 40, kCGGradientDrawsAfterEndLocation);
CGGradientRelease(gradient);
CGColorSpaceRelease(colorSpace);
Mind that I cannot test the code right now, but it should be fine. If instead of a radial gradient you want an angle gradient like this one:
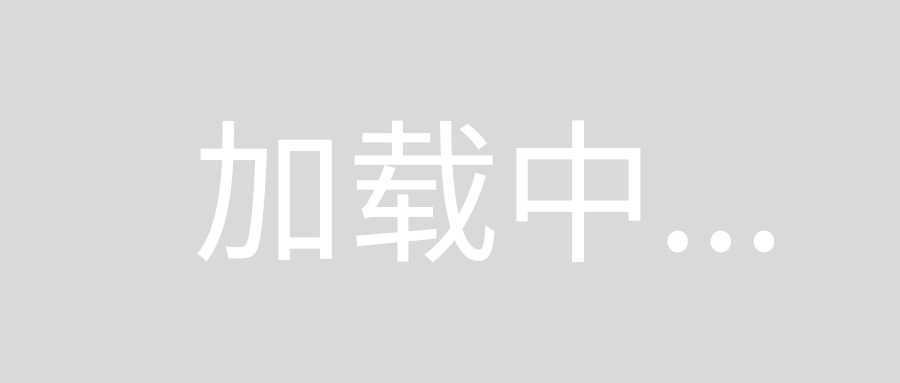
You are unlucky since Objective c has no way to make it directly. To make it you can either loop through a linear interpolation between the colors you want and directly draw them (not so hard to implement but has poor performance) or use an image as a mask.
Here's the code that produces the output shown by the attached image:
CAGradientLayer *gradientLayer = [CAGradientLayer layer];
gradientLayer.frame = self.clockView.bounds;
[self.clockView.layer addSublayer:gradientLayer];
// TO-DO:
// add durations
gradientLayer.colors = @[(__bridge id)[UIColor colorWithRed:1.0/255.0 green:025.0/255.0 blue:147.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:0.0 green:118.0/255.0 blue:186.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:153.0/255.0 green:25.0/255.0 blue:94.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:181.0/255.0 green:23.0/255.0 blue:0.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:255.0/255.0 green:147.0/255.0 blue:0.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:250.0/255.0 green:226.0/255.0 blue:50.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:255.0/255.0 green:255.0/255.0 blue:255.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:255.0/255.0 green:255.0/255.0 blue:255.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:250.0/255.0 green:226.0/255.0 blue:50.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:255.0/255.0 green:147.0/255.0 blue:0.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:181.0/255.0 green:23.0/255.0 blue:0.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:153.0/255.0 green:25.0/255.0 blue:94.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:0.0 green:118.0/255.0 blue:186.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:1.0/255.0 green:025.0/255.0 blue:147.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:1.0/255.0 green:025.0/255.0 blue:147.0/255.0 alpha:1.0].CGColor,
(__bridge id)[UIColor colorWithRed:0.0 green:0.0 blue:0.0 alpha:1.0].CGColor] ;
gradientLayer.type = kCAGradientLayerConic;
gradientLayer.startPoint = CGPointMake(0.5, 0.5);
gradientLayer.endPoint = CGPointMake(1, 1.0);
// Circular mask layer
CAShapeLayer *maskLayer = [CAShapeLayer layer];
maskLayer.frame = gradientLayer.bounds;
CGMutablePathRef circlePath = CGPathCreateMutable();
CGPathAddEllipseInRect(circlePath, NULL, CGRectInset(self.clockView.layer.bounds, 10.0 , 10.0));
maskLayer.path = circlePath;
CGPathRelease(circlePath);
gradientLayer.mask = maskLayer;
// second, smaller circle
CALayer *gradientLayer2 = [CALayer layer];
gradientLayer2.frame = gradientLayer.bounds;// CGRectMake(0.0, 0.0, self.clockView.bounds.size.width / 2.0, self.clockView.bounds.size.height / 2.0);
[gradientLayer2 setBackgroundColor:[UIColor darkGrayColor].CGColor];
[self.clockView.layer insertSublayer:gradientLayer2 atIndex:1];
// Circular mask layer
CAShapeLayer *maskLayer2 = [CAShapeLayer layer];
maskLayer2.frame = gradientLayer2.bounds;
CGMutablePathRef circlePath2 = CGPathCreateMutable();
CGPathAddEllipseInRect(circlePath2, NULL, CGRectInset(self.clockView.layer.bounds, 20.0 , 20.0));
maskLayer2.path = circlePath2;
CGPathRelease(circlePath2);
gradientLayer2.mask = maskLayer2;
[
]1