I have some simple entries in Firebase like:
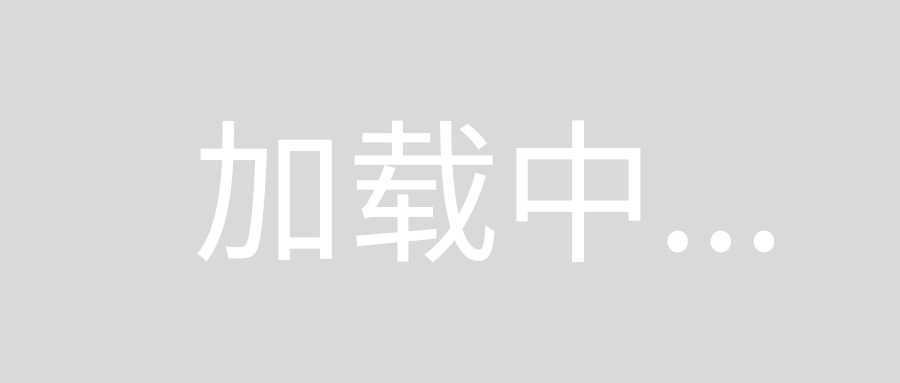
All I want is, to get these values (each percentage) and pass them into an array called labels. so that I can use that array in my code. That is my question.
Now, to me, it seemed easy but apparently it is not.
First of all what I faced is @angularfire version 5 differences in the examples(but easy to understand in their GitHub docs).
so secondly, I try to get data with:
this.items = db.list('/percentage').valueChanges();
so I do it and check it on the console.
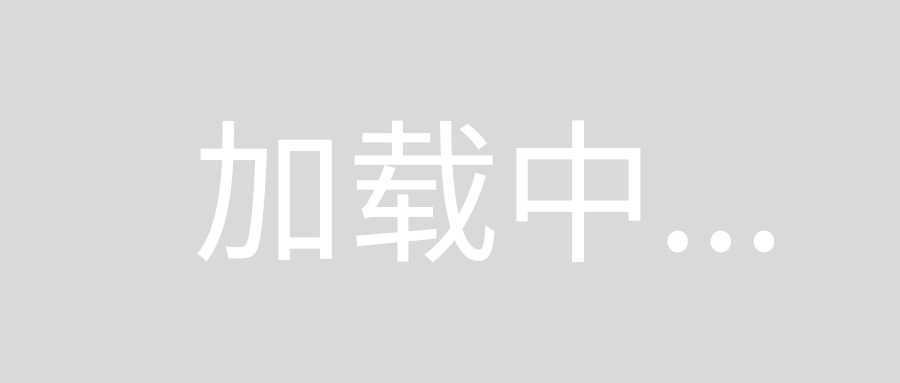
then I check out other questions on SO and thirdly, in examples, I am told 'its asynchronous data so you should subscribe to it' I do subscribe and again log it in the console to see how my array would look like.
and of course, after that I check out more examples and oh, turns out I should map() it. then I do it with
this.labels = db.list('/percentage').valueChanges().map(res => this.labels = res);
: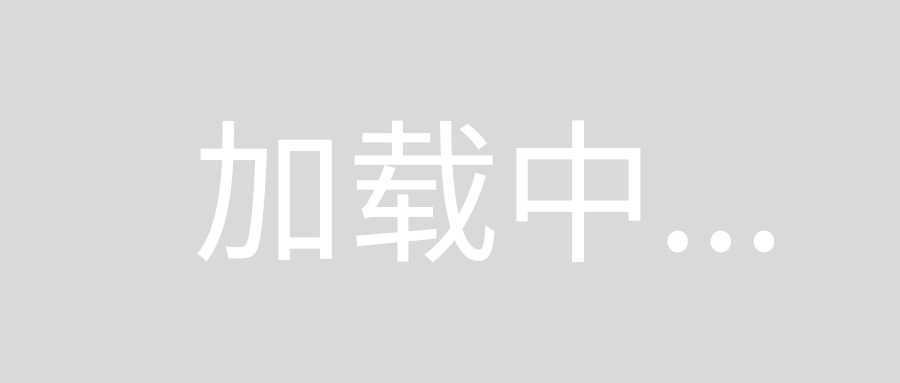
I don't get any errors but I can't figure out a way to get this data as an array which would work for me.
I would be really glad if someone explain a way to me.
Thank you
angularfire2
uses Observables
by default. It's beneficial if you read up a bit on rxjs library.
this.items = db.list('/percentage').valueChanges();
The above code will not work because db.list().valueChanges()
is technically an Observable
, as per your screenshot. Observable can be subscribed, but then again to assign the values you have to put your assignment statement in the callback function of the observables, not the observables itself. Here is how to do it:
db.list('/percentage')
.valueChanges()
.subscribe(res => {
console.log(res)//should give you the array of percentage.
this.labels = res;
})
Note that for the above, there is no this.lables = db.list()
.
On the other hand, .map()
is just another operators
in Observables. Operators are meant to use to manipulate the behaviour of the observables. How does each operator work is beyond the scope for this answer, I suggest reading up the docs.
Edit:
If you want to do some post processing on your data, that's where you will use .map()
.
db.list('/percentage')
.valueChanges()
.map(res=>{
// do some calculations here if you want to
return res.map(eachlLabel=> eachlLabel + " Hello World")
})
.subscribe(res => {
console.log(res)//should give you the array of percentage.
this.labels = res;
})
Alternatively you can do whatever post processing in your subscribe
callback:
db.list('/percentage')
.valueChanges()
.subscribe(res => {
console.log(res)//should give you the array of percentage.
//do your post processing to your res here.
res = res.map(x=> x+ " Hello World");
this.labels = res;
})
you can shown your array on html page.
<p *ngFor="let item of items | async;>
{{item}}
</p>