I want to make an edittext which aligns in its parent's left and when users click it, edittext's width'll increase to right side. Here is the code that i used. But when animation ended, edittext width come to first size.
Can any one help me.?
And is there any solution to set "fillparent" to width's final size in animation?
Animation scaleAnimation = new ScaleAnimation(0, -500, 1, 1);
scaleAnimation.setDuration(750);
editText.startAnimation(scaleAnimation);
I added scaleAnimation.setFillAfter(true);
before animation starts but i get this;
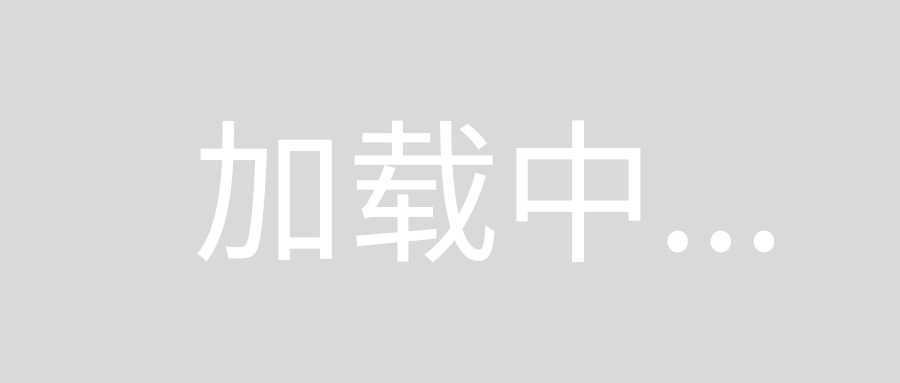
After 750ms, edittext is going to its first width.
This will definitely work for you.
Dynamic way of doing this:
ScaleAnimation animate = new ScaleAnimation(1, 2, 1, 1, Animation.RELATIVE_TO_SELF, 0, Animation.RELATIVE_TO_SELF, 0);
animate.setDuration(700);
animate.setFillAfter(true);
target.startAnimation(animate);
Way of Using XML
scale.xml (Put this in res/anim folder)
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true">
<scale
android:duration="400"
android:fillAfter="true"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:pivotX="0%"
android:pivotY="0%"
android:toXScale="2.0"
android:toYScale="1.0" >
</scale>
</set>
Java Code:
Animation anim = AnimationUtils.loadAnimation(Anims.this, R.anim.scale);
mEdittext.startAnimation(anim);
Do this
Animation scaleAnimation = new ScaleAnimation(0, -500, 1, 1);
scaleAnimation.setDuration(750);
scaleAnimation.setFillAfter(true);
editText.startAnimation(scaleAnimation);
Give a try to this one.
Animation scaleAnimation = new ScaleAnimation(0, -500, 1, 1);
scaleAnimation.setDuration(750);
scaleAnimation.setAnimationListener(new AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {}
@Override
public void onAnimationRepeat(Animation animation) {}
@Override
public void onAnimationEnd(Animation animation) {
//Set your editext width to match parent using layout params
}
});
editText.startAnimation(scaleAnimation);
Use
scaleAnimation.setFillAfter(true);
Or use ObjectAnimator:
ObjectAnimator animWidth = ObjectAnimator.ofInt(your_view, "width", startWidth,endWith);
animWidth.setDuration(yourDuration);
animWidth.start();