可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I've been really banging my head against the table trying to get the MediaPlayer class to try to play h.264-encoded videos on Android 2.1. My code is rather simple:
AssetFileDescriptor fileDescriptor = getResources().openRawResourceFd(R.raw.my_movie);
introMoviePlayer = new MediaPlayer();
introMoviePlayer.setDataSource(fileDescriptor.getFileDescriptor(), fileDescriptor.getStartOffset(), fileDescriptor.getDeclaredLength());
introMoviePlayer.prepare();
This always throws an exception at prepare()
, with the text java.io.IOException: Prepare failed.: status=0x1
. I got a bit more info by using MediaPlayer.create()
with a URI, which also throws at prepare()
, which is actually called by MediaPlayer.create()
, with the message Command PLAYER_PREPARE completed with an error or info PVMFErrResourceConfiguration
.
The same code works perfectly in Froyo (2.2). The videos themselves play fine in the video player app. Does anyone have perhaps a helpful hint that might help to solve this problem?
Edit: Still no solution -- very frustrating problem indeed. However, I have found that by creating a VideoView
and setting the URI for the raw video works. This is very puzzling, as sending the exact same URI through a MediaPlayer class will throw.
回答1:
I do not know if this is your issue, but I just found a solution to the problem the described by Tuszy above. I could read the file I was creating from external storage but not from Cache.
The solution is that the read write permissions when writing the file are different.
Please see this excellent explanation in this blog I found.
http://blog.weston-fl.com/android-mediaplayer-prepare-throws-status0x1-error1-2147483648/
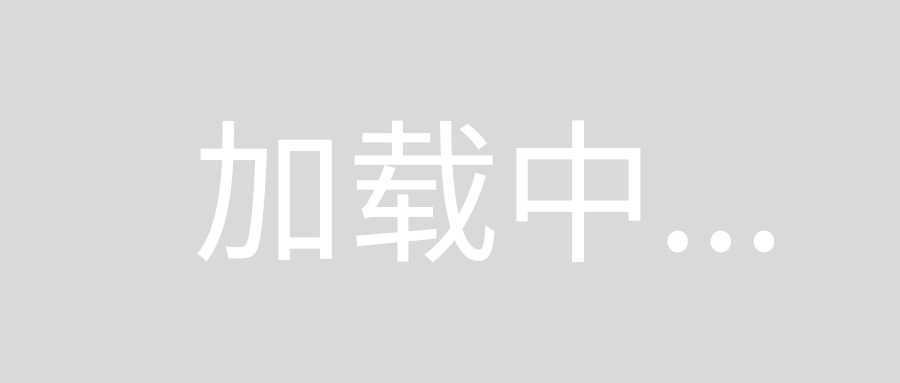
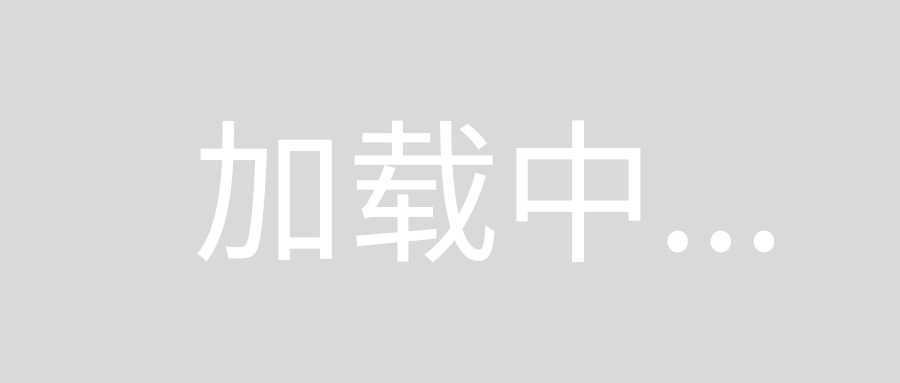
回答2:
This is my solution:
MediaPlayer mediaPlayer = new MediaPlayer();
FileInputStream fis = null;
try {
fis = new FileInputStream(mp3File);
mediaPlayer.setDataSource(fis.getFD());
mediaPlayer.setAudioStreamType(AudioManager.STREAM_MUSIC);
mediaPlayer.prepare();
} finally {
if (fis != null) {
try {
fis.close();
} catch (IOException ignore) {
}
}
}
回答3:
I know that I am late here but hopefully this helps somebody else. I worked around this issue by setting up a setOnCompletionListener
callback that explicitly releases the MediaPlayer object after the media is done playing.
I can't take credit for this solution, as it was originally posted by Ronny here:
How do you detect when a sound file has finished?
But since this is the first answer when I search for Android+Failed Status 0x1 here is my code that solved this problem for me:
public void playSound(String soundPath){
MediaPlayer m = new MediaPlayer();
m.setOnCompletionListener(new OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mp) {
mp.release();
}
});
try {
AssetFileDescriptor descriptor = mContext.getAssets().openFd(soundPath);
m.setDataSource(descriptor.getFileDescriptor(), descriptor.getStartOffset(),
descriptor.getLength());
descriptor.close();
m.prepare();
m.setVolume(100f, 100f);
m.setLooping(false);
m.start();
} catch (Exception e) {
//Your catch code here.
}
}
回答4:
If you are using multiple instances of MediaPlayer
, make sure that you've executed release()
on a resource before attempting to use it in a different instance.
回答5:
I had a similar problem. I had a zip file which contained sound files which were compressed. In order to pass them on to MediaPlayer
they needed to be uncompressed. When I placed them inside application's cache directory (context.getCacheDir()
), MediaPlayer
returned the same error which you described.
But when I placed them on external storage (Environment.getExternalStorageDirectory()
), everything started working.
回答6:
On my opinion i will ask you to check back your location of your media files<< SONG PATH or MOVIE PATH >>. I faced with this exception, i have overcome by correct "SONG PATH"
回答7:
first thanks for your code, the open raw ressource helped me with my problem.
I'm sorry I don't have a definite answer to your problem but based on the api media example in the SDK and my media player in my project I would suggest checking that the file opens correctly.
otherwise maybe you need to set the display before preparing as such:
mMediaPlayer = new MediaPlayer();
mMediaPlayer.setDataSource(fileDescriptor.getFileDescriptor(), fileDescriptor.getStartOffset(), fileDescriptor.getDeclaredLength());
mMediaPlayer.setDisplay(holder);
mMediaPlayer.prepare();
those are the only ideas I have
hope it helps
Jason
回答8:
I know this may be too late. I managed to use video from resources as this:
MediaPlayer mp = ...;
Uri uri = Uri.parse("android.resource://com.example.myapp/" + R.raw.my_movie");
mp.setDataSourceUri(this, uri);
回答9:
initilize the fileName, mediaPlayer instance:
private MediaPlayer mp;
private final static String fileName = "ring";
for playing/starting audio file from res/raw directory:
try
{
mp = new MediaPlayer();
mp.setAudioStreamType(AudioManager.STREAM_RING); //set streaming according to ur needs
mp.setDataSource(Context, Uri.parse("android.resource://yourAppPackageName/raw/"+fileName));
mp.setLooping(true);
mp.prepare();
mp.start();
} catch (Exception e)
{
System.out.println("Unable to TunePlay: startRingtone(): "+e.toString());
}
finally stopping the audioFile:
try
{
if (!(mp == null) && mp.isPlaying())
{
mp.stop();
mp.release(); //its a very good practice
}
}
catch (Exception e)
{
System.out.println("Unable to TunePlay: stopRingtone():"+e.toString());
}
回答10:
If you are dealing with remote URL, use below code to get encoded url compatible with MediaPlayer.
public String getEncodedURL(String urlStr) throws Exception
{
URL url = new URL(urlStr);
URI uri = new URI(url.getProtocol(), url.getUserInfo(), url.getHost(), url.getPort(), url.getPath(), url.getQuery(), url.getRef());
urlStr = uri.toASCIIString();
return urlStr;
}
回答11:
In my case it was just a filename that it didn't like:
/storage/emulated/0/appname/2018-03-12T11:52:08Z.wav
Removed the hyphens and colons, and the exception went away.
回答12:
I found Best and clear solution for this error : java.io.IOException: Prepare failed.: status=0x1
Note
if you want read from your sd card and got this error , your are not set
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
in your Manifast .
Note 2
if your are want play from url and got that error maybe your are set setDataSource
to like this :https://www.android.com
just remove S from http
like this http://www.android.com
回答13:
To playback a video or audio file located in the raw folder use:
mMediaPlayer = MediaPlayer.create(this, R.raw.a_video_or_audio_file);
mMediaPlayer.start();
no need to call prepare() when using MediaPlayer.create(Context,R.raw.some_resource_file)
回答14:
playing mp3 files instead of wmva fixed it. Apparently that isn't an issue on API 19