I've created a custom tag that I want to use, but Django can't seem to find it. My templatetags
directory is set up like this:
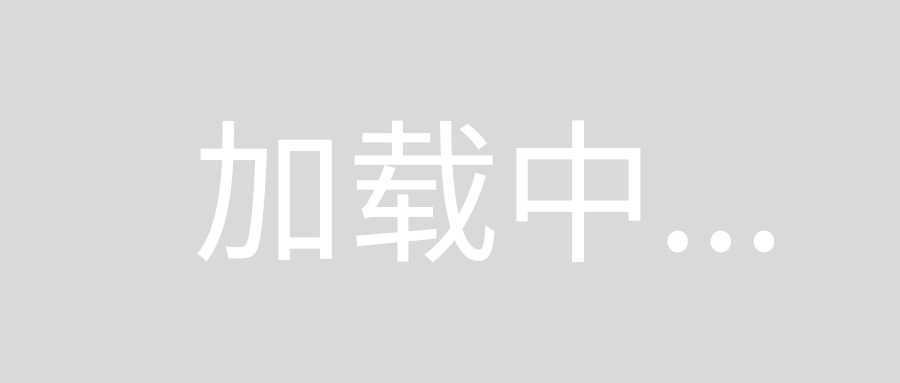
pygmentize.py
from pygments import highlight
from pygments.lexers import get_lexer_by_name
from django import template
from pygments.formatters.other import NullFormatter
register = template.Library()
@register.tag(name='code')
def do_code(parser,token):
code = token.split_contents()[-1]
nodelist = parser.parse(('endcode',))
parser.delete_first_token()
return CodeNode(code,nodelist)
class CodeNode(template.Node):
def __init__(self,lang,code):
self.lang = lang
self.nodelist = code
def render(self,context):
code = self.nodelist.render(context)
lexer = get_lexer_by_name('python')
return highlight(code,lexer,NullFormatter())
I am trying to use this tag to render code in gameprofile.html
.
gameprofile.html
(% load pygmentize %}
{% block content %}
<title>{% block title %} | {{ game.title }}{% endblock %}</title>
<div id="gamecodecontainer">
{% code %}
{{game.code}}
{% endcode %}
</div>
{% endblock content %}
When I navigate to gameprofile.html
, I get an error:
Invalid block tag on line 23: 'code', expected 'endblock'. Did you forget to register or load this tag?
did you try this
{% load games_tags %}
at the top instead of pygmentize?
The error is in this line: (% load pygmentize %}
, an invalid tag.
Change it to {% load pygmentize %}
I had the same problem, here's how I solved it. Following the first section of this very excellent Django tutorial, I did the following:
- Create a new Django app by executing:
python manage.py startapp new_app
- Edit the
settings.py
file, adding the following to the list of INSTALLED_APPS
: 'new_app',
- Add a new module to the
new_app
package named new_app_tags
.
- In a Django HTML template, add the following to the top of the file, but after
{% extends 'base_template_name.html' %}
: {% load new_app_tags %}
- In the
new_app_tags
module file, create a custom template tag (see below).
- In the same Django HTML template, from step 4 above, use your shiney new custom tag like so:
{% multiply_by_two | "5.0" %}
- Celebrate!
Example from step 5 above:
from django import template
register = template.Library()
@register.simple_tag
def multiply_by_two(value):
return float(value) * 2.0
The app that contains the custom tags must be in INSTALLED_APPS
. So Are you sure that your directory is in INSTALLED_APPS
?
From the documentation:
The app that contains the custom tags must be in INSTALLED_APPS
in order for the {% load %}
tag to work. This is a security feature: It allows you to host Python code for many template libraries on a single host machine without enabling access to all of them for every Django installation.
In gameprofile.html
please change the tag {% endblock content %}
to {% endblock %}
then it works otherwise django will not load the endblock and give error.