Apologies for such a basic question but I can't figure it out. I know you can initialize a class like this:
QFile file("C:\\example");
But how would you initialize it from a global variable? For example:
QFile file; //QFile class
int main()
{
file = ?? //need to initialize 'file' with the QFile class
}
1. Straightforward answer
If the class is assignable/copy constructible you can just write
QFile file; //QFile class
int main()
{
file = QFile("C:\\example");
}
2. Use indirection
If not, you'll have to resort to other options:
QFile* file = 0;
int main()
{
file = new QFile("C:\\example");
//
delete file;
}
Or use boost::optional<QFile>
, std::shared_ptr<QFile>
, boost::scoped_ptr<QFile>
etc.
3. Use singleton-related patterns:
Due to the Static Initialization Fiasco you could want to write such a function:
static QFile& getFile()
{
static QFile s_file("C:\\example"); // initialized once due to being static
return s_file;
}
C++11 made such a function-local static initialization thread safe as well (quoting the C++0x draft n3242, §6.7:)
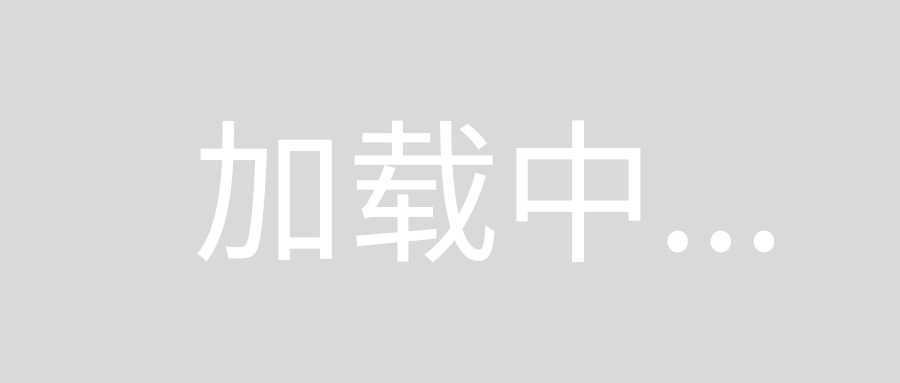
The same way:
// file.cpp
QFile file("C:\\example");
int main()
{
// use `file`
}
The constructors of all global objects execute before main()
is invoked (and inversely for destructors).
#include <iostream>
struct QFile
{
char const* name;
QFile(char const* name) : name(name) {}
};
QFile file("test.dat"); // global object
int main()
{
std::cout << file.name << std::endl;
}
By the way, you wrote:
I know you can initialize a class
You can not initialize a class. You create and initialize object, not a class.
It is totally up to you to decide whether you use a global variable or some thing else.
For a global variable you can define it in globally in the file and then initialize it in function as below, make sure that you write copy constructor properly.
QFile file; //class object
int main()
{
file = QFile("C:\\example"); //create a temporary object and copy it to `file`
}
But, it is better if you use global pointer,
QFile *file;
int main()
{
file = new QFile("C:\\example");
}
Note, if you are using this global variable only within the file, you can make it a static
variable. Then it is limited to file itself. So you can minimize the bad of global variables.