* I have rephrased the post since originally posted *
When I try to run a just-built release apk, I get an error "the apk for your currently selected variant ... is not signed." This is in the Edit Configuration popup. Here are my steps:
- In the Build Variants tab, select "release"
- In the menu, choose Build -> Generate Signed APK
- In the popup, fill in the fields for the key store and passwords.
- In the second panel, change the destination folder to ...\app\build\outputs\apk (see note * below)
- Observe notification in upper right of studio: APK(s) generated successfully.
- In the menu, click Run -> Run App.
- I get an "Edit configuration" popup with the error "The apk for your currently selected variant ... is not signed.
So, why this error? The APK generated appears to be valid. I have successfully posted it to the Android Store (alpha testing only) and verified that stack dumps are obfuscated.
What I can't do is download it (step 6 above) to my device. I guess that's ok since I can download the debug version just fine.
(*) Android Studio defaults the output for the release apk to a higher, presumably more convenient directory. However I find it harder to manage the consistency of generated files when they are scattered about so I prefer all the generated apks in one place.
Set signing config in project structure.
- File -> Project Structure...
- Select Modules/app (or other module)
- Click Signing tab and fill in.
Key Alias and Key Password comes first.
Not same order in "Generate Signed APK" dialog.
- Click Build Types tab and select release.
Select "config" in Signing config dropdown list.
- Click OK to close Project Structure.
- Run -> Run app
Run (or Debug) app seems to use apks built with "Buiild -> Build APK".
So, we should set signing config if build variants of app module is "release".
Go to File\Project Structure
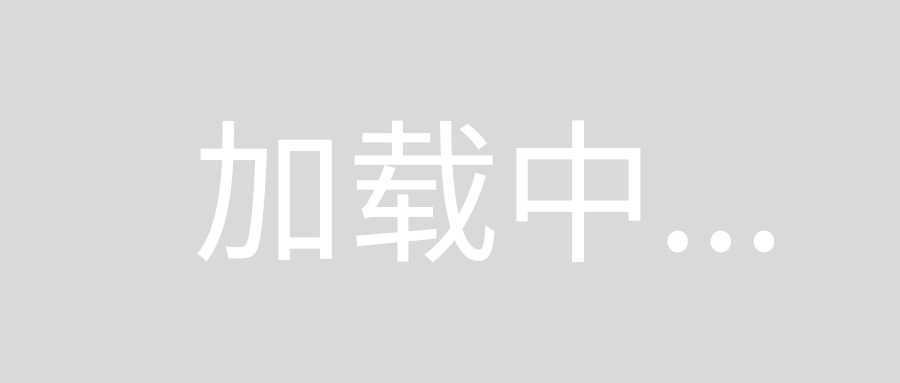
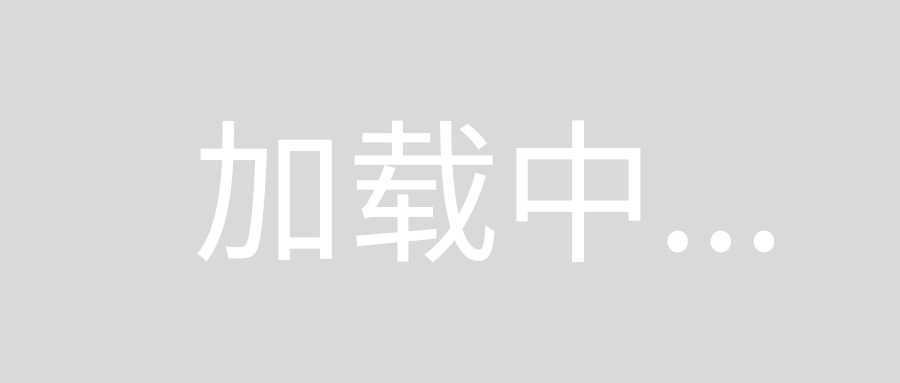
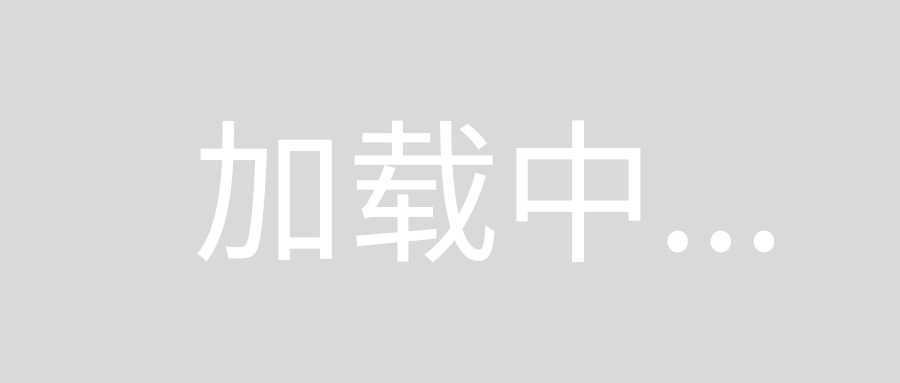
Done! ;)
Try add this in your build file:
buildTypes {
release {
signingConfig signingConfigs.release
minifyEnabled true
shrinkResources true
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
debug {
minifyEnabled false
}
}
First, Create a keystore file if there isn't one.
- Click Build from the dropdown menu
- Select Generate Signed APK
- Click Next
- Click Create New Keystore
- Fill out the form for keystore path, alias, password for keystore and alias, at least one field in the certificate area.
- Click OK
- A keystore file should be created in the specified keystore path.
Second update the app build gradle file to someting like this one to include the signing config.
android {
signingConfigs {
config {
keyAlias 'mykeyalias'
keyPassword 'android'
storeFile file('/Users/yourname/path/to/the/android/project/folder/android_project_folder_name/app/debug.keystore')
storePassword 'android'
}
}
buildTypes {
debug {
applicationIdSuffix = ".debug"
versionNameSuffix "-debug"
}
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
signingConfig signingConfigs.config
}
}
}
Third, build and run the app, done.
In https://developer.android.com/studio/publish/app-signing#secure-shared-keystore it is written that you shouldn't keep credentials information in build.gradle
and VCS. So create a signing config file (Build > Generate Signed APK...), then do so.
Create a file named keystore.properties
in the root directory of your
project. This file should contain your signing information, as
follows:
storePassword=myStorePassword
keyPassword=mykeyPassword
keyAlias=myKeyAlias
storeFile=myStoreFileLocation
In your module's build.gradle file, add code to load your
keystore.properties
file before the android {}
block.
// Create a variable called keystorePropertiesFile, and initialize it to your
// keystore.properties file, in the rootProject folder.
def keystorePropertiesFile = rootProject.file("keystore.properties")
// Initialize a new Properties() object called keystoreProperties.
def keystoreProperties = new Properties()
// Load your keystore.properties file into the keystoreProperties object.
keystoreProperties.load(new FileInputStream(keystorePropertiesFile))
android {
...
}
You can refer to properties stored in keystoreProperties
using the
syntax keystoreProperties['propertyName']
. Modify the signingConfigs
block of your module's build.gradle
file to reference the signing
information stored in keystoreProperties
using this syntax.
android {
signingConfigs {
config {
keyAlias keystoreProperties['keyAlias']
keyPassword keystoreProperties['keyPassword']
storeFile file(keystoreProperties['storeFile'])
storePassword keystoreProperties['storePassword']
}
}
...
}
Optionally in build.gradle
you can add:
buildTypes {
release {
...
signingConfig signingConfigs.config
}
}
Now you may make a signed apk. Don't forget to exclude keystore.properties
from VCS.
I had the same issue turned out I misconfigured the signingConfigs
property.
Specifically, I thought I didn't have a password for the key where I actually had set it. After adding the missing information, it worked.
signingConfigs {
config {
keyAlias 'key0'
storeFile file('C:/Users/xxx/xxx/keystore/xxx.jks')
storePassword '123'
keyPassword '123' // this was missing
}
}