I'm developing my first iOS app which contains a UISlider
. I know how to get the value when the UISlider
is dragged. But for my app I need to get the slider's value in a single touch; i.e. if I touch somewhere in the UISlider
, a UILabel
should display its correct value.
Is it possible to this way. Any tutorial or code will very helpful.
Please Write Following Code may be helpful for you :)
Here tapCount is int
variable that declare in .h file
- (void)viewDidLoad
{
tapCount = 0; // Count tap on Slider
UITapGestureRecognizer *gr = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(sliderTapped:)];
[slider addGestureRecognizer:gr];
}
- (void)sliderTapped:(UIGestureRecognizer *)g
{
/////////////// For TapCount////////////
tapCount = tapCount + 1;
NSLog(@"Tap Count -- %d",tapCount);
/////////////// For TapCount////////////
UISlider* s = (UISlider*)g.view;
if (s.highlighted)
return; // tap on thumb, let slider deal with it
CGPoint pt = [g locationInView: s];
CGFloat percentage = pt.x / s.bounds.size.width;
CGFloat delta = percentage * (s.maximumValue - s.minimumValue);
CGFloat value = s.minimumValue + delta;
[s setValue:value animated:YES];
NSString *str=[NSString stringWithFormat:@"%.f",[self.slider value]];
self.lbl.text=str;
}
For Swift User
override func viewDidLoad()
{
super.viewDidLoad()
tapCount = 0
let gr = UITapGestureRecognizer(target: self, action: #selector(self.sliderTapped(_:)))
slider.addGestureRecognizer(gr)
}
func sliderTapped(_ g: UIGestureRecognizer) {
/////////////// For TapCount////////////
tapCount = tapCount + 1
print("Tap Count -- \(tapCount)")
/////////////// For TapCount////////////
let s: UISlider? = (g.view as? UISlider)
if (s?.isHighlighted)! {
return
}
// tap on thumb, let slider deal with it
let pt: CGPoint = g.location(in: s)
let percentage = pt.x / (s?.bounds.size.width)!
let delta = Float(percentage) * Float((s?.maximumValue)! - (s?.minimumValue)!)
let value = (s?.minimumValue)! + delta
s?.setValue(Float(value), animated: true)
let str = String(format: "%.f", slider.value)
lbl.text = str
}
Correction @ipatel: Math in previous answers is incomplete and causes jitter due to missing thumb width considerations.
Here is what it should look like if you choose to subclass UISlider
:
- (BOOL)beginTrackingWithTouch:(UITouch *)touch withEvent:(UIEvent *)event
{
[super beginTrackingWithTouch:touch withEvent:event];
CGPoint touchLocation = [touch locationInView:self];
CGFloat value = self.minimumValue + (self.maximumValue - self.minimumValue) *
((touchLocation.x - self.currentThumbImage.size.width/2) /
(self.frame.size.width-self.currentThumbImage.size.width));
[self setValue:value animated:YES];
return YES;
}
Swift version for accepted answer,
@IBAction func sliderTappedAction(sender: UITapGestureRecognizer)
{
if let slider = sender.view as? UISlider {
if slider.highlighted { return }
let point = sender.locationInView(slider)
let percentage = Float(point.x / CGRectGetWidth(slider.bounds))
let delta = percentage * (slider.maximumValue - slider.minimumValue)
let value = slider.minimumValue + delta
slider.setValue(value, animated: true)
}
}
You can display the current value of UISlider
with its action method like below.
- (IBAction) sliderValueChanged:(UISlider *)sender {
yourLable.text = [NSString stringWithFormat:@"%.1f", [sender value]];
}
See these tutorials and examples of UISlider
:
- UISlider-tutorial-example-how-to-use-slider-in-iphone-sdk-xcode.
- iphone-slider-control-uislider-control-tutorial.
This second tutorial with example which display the current (Latest) value of UISlider
in UILable
see the image below for example.
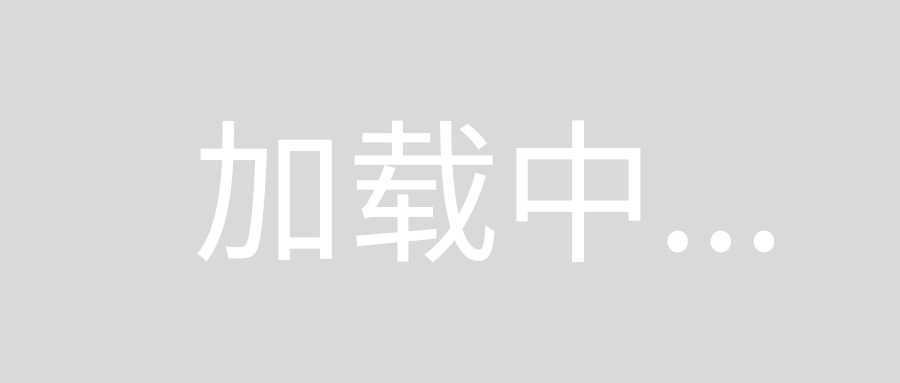