So I want to do a very simple task using webpack.
I have a few static HTML templates like e.g.
test.html
<div><span>template content</span></div>
and all I want to do is return the string inside the template
e.g
require("raw!./test.html")
with should return a string like:
"<div><span>template content</span></div>"
but instead, it returns the following string
"modules.exports = <div><span>template content</span></div>"
I have tried several modules, like the raw-loader and html-loader.
and they both behave the same way.So I took a look at the source code, just to find out that its SUPPOSED to behave this way.
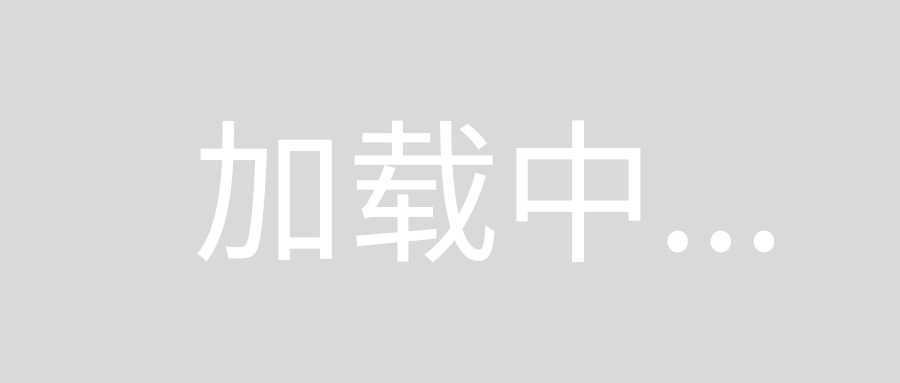
so what exactly am I expected to do with this, if I just want the raw
HTML? is it a bad practice just to remove the prepended
"module.exports =" string? from the bundle
edit: removing the 'modules.export =' part results in the bundle returning nothing :/
my config
module.exports =
{
module:
{
loaders:
[
{ test: /\.html$/, loader: "raw-loader" }
]
}
};
The solution is to require your file without specifying any additional loader, as this is already specified in the webpack config
const test = require('./test.html')
Explanation: With your current code, you are applying the raw
loader twice to your file. When you specify a loader chain in your configuration:
loaders:
[
{ test: /\.html$/, loader: "raw-loader" }
]
... you are already telling webpack to add this loader to the loader chain every time you require a file matching the test
condition (here, every html file)
Therefore, when you write this
const test = require('raw!./test.html')
... it is actually equivalent to this
const test = require('raw!raw!./test.html')
I finally figured it out I think. You need to resolve the path name using require.resolve(./test.html)
https://nodejs.org/dist/latest-v7.x/docs/api/globals.html#globals_require
When you write require('./test.html')
it means that you simply run the code returned by the loaders chain. The result is exported in this code as module.exports
. To use this result you need to assign your require statement to variable:
var htmlString = require('raw!./test.html');
//htmlString === "<div><span>template content</span></div>"
Remember that any loader in Webpack returns JS code - not HTML, not CSS. You can use this code to get HTML, CSS and whatever.