I want to read a .class
file that is located inside of a .jar
package. How can I read a readable .class
file from a .jar
package?
My environment is:
- Language version:
Java
- Platform version:
Java 1.8.0_73
- Runtime:
Java(TM) SE Runtime Environment (build 1.8.0_73-b02)
- VM Server:
Java HotSpot(TM) 64-Bit Server VM (build 25.73-b02, mixed mode)
- Operating system:
Windows 10 Home (64-bit) [build 10586]
EDIT:
My extracted .class
file that contains binary & compiled bytecode:
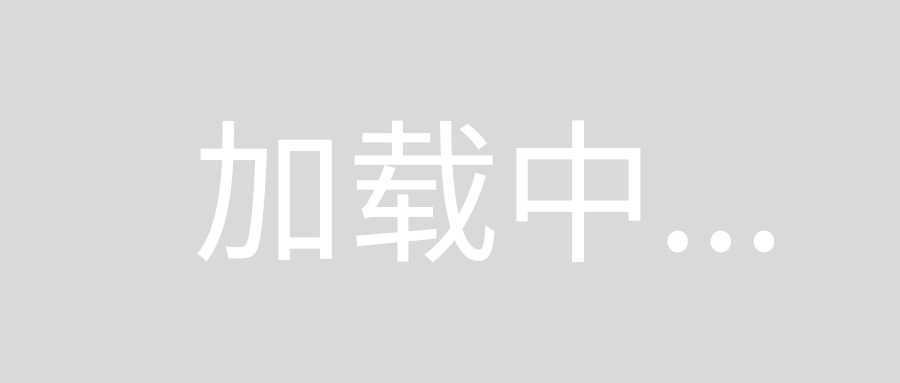
The output I want:
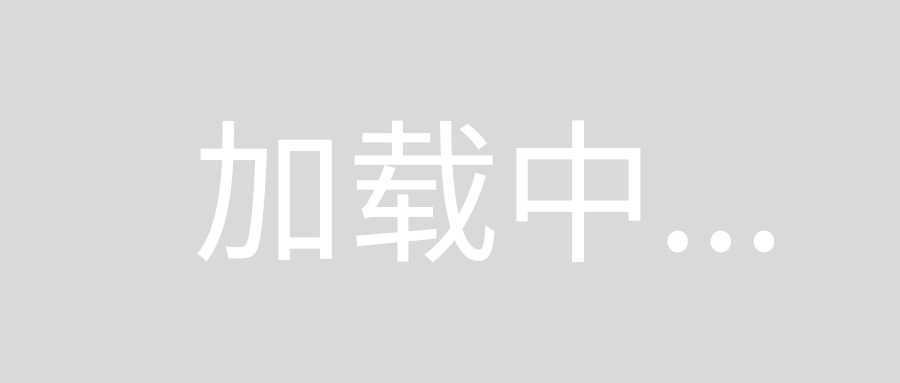
Use a decompiler. I prefer using Fernflower, or if you use IntelliJ IDEA, simply open .class files from there, because it has Fernflower pre-installed.
Or, go to javadecompilers.com, upload your .jar file, use CFR and download the decompiled .zip file.
However, in some cases, decompiling code is quite illegal, so, prefer to learn instead of decompiling.
Extract the Contents of .zip
/.jar
files programmatically
Suppose .jar
file is the .jar
/.zip
file to be extracted. destDir
is the path where it will be extracted:
java.util.jar.JarFile jar = new java.util.jar.JarFile(jarFile);
java.util.Enumeration enum = jar.entries();
while (enum.hasMoreElements()) {
java.util.jar.JarEntry file = (java.util.jar.JarEntry) enum.nextElement();
java.io.File f = new java.io.File(destDir + java.io.File.separator + file.getName());
if (file.isDirectory()) { // if its a directory, create it
f.mkdir();
continue;
}
java.io.InputStream is = jar.getInputStream(file); // get the input stream
java.io.FileOutputStream fos = new java.io.FileOutputStream(f);
while (is.available() > 0) { // write contents of 'is' to 'fos'
fos.write(is.read());
}
fos.close();
is.close();
}