可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
Is there any simple way to turn Crashlytics Android SDK off while developing ?
I don't want it to send a crash every time I do something stupid
On the other hand I don't want to comment out Crashlytics.start()
and possibly risk forgetting to uncomment it and commit
回答1:
Marc from Crashlytics here. Here's a couple of ways to disable Crashlytics while you are doing your debug builds!
Use a different android:versionString for debug and release builds and then disable crash reporting from the Crashlytics web dashboard for the debug version.
Wrap the call to Crashlytics.start() in an if statement that checks a debug flag. You could use either a custom flag or an approach like the ones proposed here: How to check if APK is signed or "debug build"?
回答2:
I found the solution from Crashlytics (with Fabric integration)
Put following code inside your Application class onCreate()
Crashlytics crashlytics = new Crashlytics.Builder().disabled(BuildConfig.DEBUG).build();
Fabric.with(this, crashlytics);
EDIT:
In Crashalitics 2.3 and above, this is deprecated. The correct code is:
CrashlyticsCore core = new CrashlyticsCore.Builder().disabled(BuildConfig.DEBUG).build();
Fabric.with(this, new Crashlytics.Builder().core(core).build());
or
Fabric.with(this, new Crashlytics.Builder().core(new CrashlyticsCore.Builder().disabled(BuildConfig.DEBUG).build()).build());
(copied from Crashlytics deprecated method disabled())
EDIT2:
You can also optionally add this to your buildType
in gradle. This command disables sending the crashlytics mapping file and generating an ID for each build, which speeds up gradle builds of those flavors. (It doesn't disable Crashlytics at run time.) See Mike B's answer here.
buildTypes {
release {
....
}
debug {
ext.enableCrashlytics = false
}
}
回答3:
If you use Gradle just add this to a flavor:
ext.enableCrashlytics = false
回答4:
Check out the latest doc.
https://docs.fabric.io/android/crashlytics/build-tools.html#gradle-advanced-setup.
Apart from adding ext.enableCrashlytics = false
in build.grade you need to do,
Crashlytics crashlyticsKit = new Crashlytics.Builder()
.core(new CrashlyticsCore.Builder().disabled(BuildConfig.DEBUG).build())
.build();
// Initialize Fabric with the debug-disabled crashlytics.
Fabric.with(this, crashlyticsKit);
回答5:
The chosen answer is not correct any more. Google changed the integration of Crashlytics. My current version is 2.9.1
and the only thing I had to do is, to add implementation 'com.crashlytics.sdk.android:crashlytics:2.9.1'
to my Gradle file. No further things required, nice but this means that Crashlytics is always running.
Solution 1
Only compile Crashlytics in release version:
dependencies {
...
releaseImplementation 'com.crashlytics.sdk.android:crashlytics:2.9.1' // update version
}
Solution 2
If you want to additionally configure Crashlytics then Solution 1 is not working, since the Crashlytics classes will not be found in Debug Builds. So change the Gradle implementation back to:
implementation 'com.crashlytics.sdk.android:crashlytics:2.9.1' // update version
Then go to your Manifest and add the following meta-data
tag inside the application
tag:
<application
android:name="...>
<meta-data
android:name="firebase_crashlytics_collection_enabled"
android:value="false" />
...
</application>
Add to your Launch-Activity (only one-time required, not every Activity)
if (!BuildConfig.DEBUG) { // only enable bug tracking in release version
Fabric.with(this, new Crashlytics());
}
This will only enable Crashlytics in release versions. Be careful, also check for BuildConfig.DEBUG when you then configure Crashlytics, E.g:
if (!BuildConfig.DEBUG) {
Crashlytics.setUserIdentifier("HASH_ID");
}
Best,
Paul
回答6:
I found this to be the the easiest solution:
release {
...
buildConfigField 'Boolean', 'enableCrashlytics', 'true'
}
debug {
buildConfigField 'Boolean', 'enableCrashlytics', 'false'
}
The above lines will create a static boolean field called enableCrashlytics
in the BuildConfig
file which you can use to decide whether to initiate Fabric
or not:
if (BuildConfig.enableCrashlytics)
Fabric.with(this, new Crashlytics());
NOTE: With this method Fabrics is initialised only in release builds (as indicative in the above code). This means you need to put calls to statics methods in the Crashlytics
class in an if
block which checks whether Fabrics has been initialised as shown below.
if (Fabric.isInitialized())
Crashlytics.logException(e);
Otherwise the app will crash with Must Initialize Fabric before using singleton()
error when testing on the emulator.
回答7:
Use this in MyApplication#onCreate()
if (!BuildConfig.DEBUG) Crashlytics.start(this);
EDIT
If you upgraded to Fabric, use this answer instead.
回答8:
If you want to capture all crashes (for debug and release builds) but want to separate them in the Crashlytics Dashboard, you can add this line of code to build.gradle:
debug {
versionNameSuffix "-DEBUG"
}
For example, if your app's versionName is 1.0.0, your release builds will be tagged as 1.0.0 while debug builds will be 1.0.0-DEBUG
回答9:
There are plenty of good answers here, but for my testing I use debug builds for in-house betas and out-of-the-lab testing where crash logs are still very useful and I would still like to report them. Like the OP, all I wanted was to disable them during active development where I am often causing and quickly resolving crashes.
Rather than remove ALL debug crashes you can choose to only disable the reports while a device is connected to your development machine with the following code.
if (!Debug.isDebuggerConnected()) {
Fabric.with(this, new Crashlytics());
}
回答10:
Notice that you can also disable the annoying uploading of symbols in debug build:
def crashlyticsUploadStoredDeobsDebug = "crashlyticsUploadStoredDeobsDebug"
def crashlyticsUploadDeobsDebug = "crashlyticsUploadDeobsDebug"
tasks.whenTaskAdded { task ->
if (crashlyticsUploadStoredDeobsDebug.equals(task.name) ||
crashlyticsUploadDeobsDebug.equals(task.name)) {
println "Disabling $task.name."
task.enabled = false
}
}
Just put it into the build.gradle
of your application module.
回答11:
The problem is that none of the solutions does work for the latest crashlytics sdk. (I'm using 2.9.0)
You can't disable it by code since it compiles into your project and runs before even a call onCreate of your application. So other solution is simple - don't compile crashlytics when not needed.
Replace the 'compile' call with 'releaseCompile' within build.gradle file.
releaseCompile('com.crashlytics.sdk.android:crashlytics:2.9.0@aar') {
transitive = true
}
回答12:
Up to date easiest version when using Gradle to build:
if (!BuildConfig.DEBUG) {
Fabric.with(this, new Crashlytics());
}
It uses the new Built-In Syntax from Fabric for Crashlytics and works automatically with the a Gradle build.
回答13:
If you're worried about BuildConfig.DEBUG
not being set up correctly, use ApplicationInfo
instead:
boolean isDebug = ( mAppContext.getApplicationInfo().flags & ApplicationInfo.FLAG_DEBUGGABLE ) != 0;
Crashlytics crashlytics = new Crashlytics.Builder().disabled( isDebug ).build();
Fabric.with( uIContext, crashlytics );
回答14:
Use flavors or build configs. Use a separate build identifier for dev build and all your crashes will keep going to a separate app. Can come handy in case of sharing the build with peers or using it without a debugger. Something like this -
productFlavors {
dev {
applicationId "io.yourapp.developement"
}
staging {
applicationId "io.yourapp.staging"
}
production {
applicationId "io.yourapp.app"
}
回答15:
A strange problem that I encountered: I followed xialin's answer (which also appears on the official website) and it didn't work. Turned out that I was referencing BuildConfig
in Fabric's package which also contains a static DEBUG variable that was set to false even in debug mode.
So, if you follow the aforementioned solution and you still get debug reports, make sure that you're referencing this:
import com.yourpackagename.BuildConfig;
And not this:
import io.fabric.sdk.android.BuildConfig;
回答16:
If you want a debuggable release build, here's the way:
buildTypes {
release {
signingConfig signingConfigs.config
debuggable true //-> debuggable release build
minifyEnabled true
multiDexEnabled false
ext.enableCrashlytics = true
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
buildConfigField 'boolean', 'BUILD_TYPE_DEBUG', 'false'
}
debug {
minifyEnabled false
multiDexEnabled true
ext.enableCrashlytics = false
ext.alwaysUpdateBuildId = false
// Disable fabric build ID generation for debug builds
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
buildConfigField 'boolean', 'BUILD_TYPE_DEBUG', 'true'
}
}
When you set debuggable true
your BuildConfig.DEBUG will initialized with true, that's why I added that variable in BuildConfig class.
Init Fabric:
Crashlytics crashlytics = new Crashlytics.Builder()
// disable crash reporting in debug build types with custom build type variable
.core(new CrashlyticsCore.Builder().disabled(BuildConfig.BUILD_TYPE_DEBUG).build())
.build();
final Fabric fabric = new Fabric.Builder(this)
.kits(crashlytics)
//enable debugging with debuggable flag in build type
.debuggable(BuildConfig.DEBUG)
.build();
// Initialize Fabric with the debug-disabled crashlytics.
Fabric.with(fabric);
回答17:
As per google use this code for disable Crashlytics and it will also improve build process.
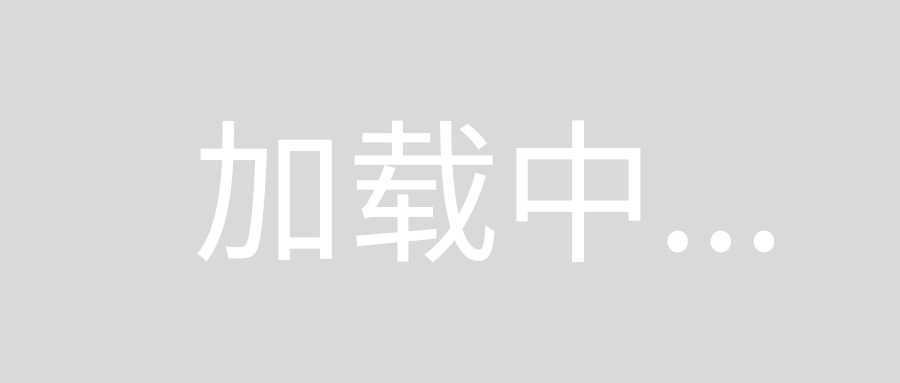
reference-https://developer.android.com/studio/build/optimize-your-build
回答18:
Another simple solution that I like, because it doesn't require different manifest files:
Step 1 - define manifest placeholders in build.gradle
android {
...
buildTypes {
release {
manifestPlaceholders = [crashlytics:"true"]
}
debug {
manifestPlaceholders = [crashlytics:"false"]
}
}
...
}
Step 2 - use them in your AndroidManifest.xml
<meta-data
android:name="firebase_crashlytics_collection_enabled"
android:value="${crashlytics}" />
回答19:
Another way if you only want to do it on your IDE is to logout of the plugin. Apparently it will stop sending reports while you're generating builds without logging in again.
回答20:
We can use fabric's isDebuggable() method.
import static io.fabric.sdk.android.Fabric.isDebuggable;
if(! isDebuggable()){
// set Crashlytics ...
}
Happy coding :)
回答21:
1. Add this to your app’s build.gradle:
android {
buildTypes {
debug {
// Disable fabric build ID generation for debug builds
ext.enableCrashlytics = false
...
2. Disable the Crashlytics kit at runtime. Otherwise, the Crashlytics kit will throw the error:
// Set up Crashlytics, disabled for debug builds
// Add These lines in your app Application class onCreate method
Crashlytics crashlyticsKit = new Crashlytics.Builder()
.core(new CrashlyticsCore.Builder().disabled(BuildConfig.DEBUG).build())
.build();
// Initialize Fabric with the debug-disabled crashlytics.
Fabric.with(this, crashlyticsKit);
3. In AndroidManifest.xml, add
<meta-data android:name="firebase_crashlytics_collection_enabled" android:value="false" />
回答22:
You can use a dedicated manifest file for debug mode (works for me with Crashlytics 2.9.7):
Create the file app/src/debug/AndroidManifest.xml
and add the following:
<application>
<meta-data
android:name="firebase_crashlytics_collection_enabled"
android:value="false"/>
</application>
Note that this meta-data element must be put into debug/AndroidManifest.xml only, and not into the regular AndroidManifest.xml
The solution which uses CrashlyticsCore.Builder().disabled(BuildConfig.DEBUG).build()
did not work for me, and I found out that crashlytics is initialized by the CrashlyticsInitProvider before Application.onCreate() is called or an any activity is started, which means that manually initializing fabric in the application or an activity has no effect because fabric is already initialized.
回答23:
This is silly answer, I know
Just comment out Fabric.with(this, new Crashlytics());
, work on that and uncomment it when you want to release it.