可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I'm migrating from ActionBar
to Toolbar
in my application.
But I don't know how to display and set click event on Back Arrow on Toolbar
like I did on Actionbar
.
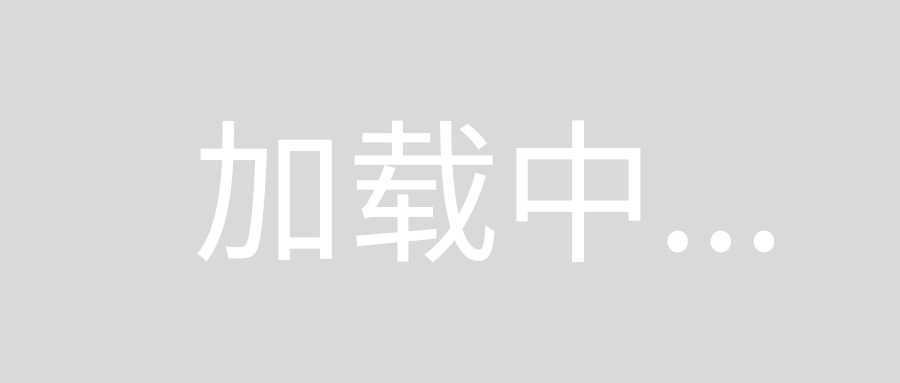
With ActionBar
, I call mActionbar.setDisplayHomeAsUpEnabled(true)
.
But there is no the similar method like this.
Has anyone ever faced this situation and somehow found a way to solve it?
回答1:
If you are using an ActionBarActivity
then you can tell Android to use the Toolbar
as the ActionBar
like so:
Toolbar toolbar = (Toolbar) findViewById(R.id.my_awesome_toolbar);
setSupportActionBar(toolbar);
And then calls to
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
will work. You can also use that in Fragments that are attached to ActionBarActivities
you can use it like this:
((ActionBarActivity) getActivity()).getSupportActionBar().setDisplayHomeAsUpEnabled(true);
((ActionBarActivity) getActivity()).getSupportActionBar().setDisplayShowHomeEnabled(true);
If you are not using ActionBarActivities
or if you want to get the back arrow on a Toolbar
that's not set as your SupportActionBar
then you can use the following:
mActionBar.setNavigationIcon(getResources().getDrawable(R.drawable.ic_action_back));
mActionBar.setNavigationOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//What to do on back clicked
}
});
If you are using android.support.v7.widget.Toolbar
, then you should add the following code to your AppCompatActivity
:
@Override
public boolean onSupportNavigateUp() {
onBackPressed();
return true;
}
回答2:
I see a lot of answers but here is mine which is not mentioned before. It works from API 8+.
public class DetailActivity extends AppCompatActivity
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_detail);
// toolbar
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
// add back arrow to toolbar
if (getSupportActionBar() != null){
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
}
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// handle arrow click here
if (item.getItemId() == android.R.id.home) {
finish(); // close this activity and return to preview activity (if there is any)
}
return super.onOptionsItemSelected(item);
}
回答3:
There are many ways to achieve that, here is my favorite:
Layout:
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:navigationIcon="?attr/homeAsUpIndicator" />
Activity:
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
toolbar.setNavigationOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// back button pressed
}
});
回答4:
you can use the tool bar setNavigationIcon method.
Android Doc
mToolBar.setNavigationIcon(R.drawable.abc_ic_ab_back_mtrl_am_alpha);
mToolBar.setNavigationOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
handleOnBackPress();
}
});
回答5:
I used this method from the Google Developer Documentation:
@Override
public void onCreate(Bundle savedInstanceState) {
...
getActionBar().setDisplayHomeAsUpEnabled(true);
}
If you get a null pointer exception it could depend on the theme. Try using a different theme in the manifest or use this alternatively:
@Override
public void onCreate(Bundle savedInstanceState) {
...
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
}
Then in the manifest, where I set the parent activity for current activity:
<activity
android:name="com.example.myapp.MyCurrentActivity"
android:label="@string/title_activity_display_message"
android:parentActivityName="com.example.myfirstapp.MainActivity" >
<!-- Parent activity meta-data to support 4.0 and lower -->
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value="com.example.myapp.MyMainActivity" />
</activity>
I hope this will help you!
回答6:
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
toolbar.setNavigationIcon(R.drawable.back_arrow); // your drawable
toolbar.setNavigationOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
onBackPressed(); // Implemented by activity
}
});
And for API 21+ android:navigationIcon
<android.support.v7.widget.Toolbar
android:navigationIcon="@drawable/back_arrow"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"/>
回答7:
If you don't want to create a custom Toolbar
, you can do like this
public class GalleryActivity extends AppCompatActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
...
getSupportActionBar().setTitle("Select Image");
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
if (item.getItemId() == android.R.id.home) {
finish();
}
return super.onOptionsItemSelected(item);
}
}
In you AndroidManifest.xml
<activity
android:name=".GalleryActivity"
android:theme="@style/Theme.AppCompat.Light"
>
</activity>
you can also put this android:theme="@style/Theme.AppCompat.Light"
to <aplication>
tag, for apply to all activities
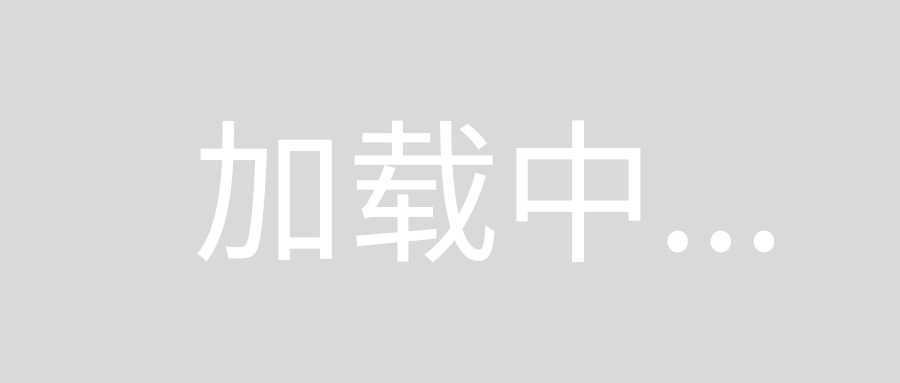
回答8:
If you were using AppCompatActivity
and have gone down the path of not using it, because you wanted to not get the automatic ActionBar
that it provides, because you want to separate out the Toolbar
, because of your Material Design needs and CoordinatorLayout
or AppBarLayout
, then, consider this:
You can still use the AppCompatActivity
, you don't need to stop using it just so that you can use a <android.support.v7.widget.Toolbar>
in your xml. Just turn off the action bar style as follows:
First, derive a style from one of the NoActionBar themes that you like in your styles.xml
, I used Theme.AppCompat.Light.NoActionBar
like so:
<style name="SuperCoolAppBarActivity" parent="Theme.AppCompat.Light.NoActionBar">
<item name="colorPrimary">@color/primary</item>
<!-- colorPrimaryDark is used for the status bar -->
<item name="colorPrimaryDark">@color/primary_dark</item>
...
...
</style>
In your App's manifest, choose the child style theme you just defined, like so:
<activity
android:name=".activity.YourSuperCoolActivity"
android:label="@string/super_cool"
android:theme="@style/SuperCoolAppBarActivity">
</activity>
In your Activity Xml, if the toolbar is defined like so:
...
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
/>
...
Then, and this is the important part, you set the support Action bar to the AppCompatActivity that you're extending, so that the toolbar in your xml, becomes the action bar. I feel that this is a better way, because you can simply do the many things that ActionBar allows, like menus, automatic activity title, item selection handling, etc. without resorting to adding custom click handlers, etc.
In your Activity's onCreate override, do the following:
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_super_cool);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
//Your toolbar is now an action bar and you can use it like you always do, for example:
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
}
回答9:
MyActivity extends AppCompatActivity {
private Toolbar toolbar;
@Override
protected void onCreate(Bundle savedInstanceState) {
...
toolbar = (Toolbar) findViewById(R.id.my_toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
toolbar.setNavigationOnClickListener(arrow -> onBackPressed());
}
回答10:
In the AppCompatActivity
for example you can do
public class GrandStatActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_grand_stat);
}
@Override
public void onResume() {
super.onResume();
// Display custom title
ActionBar actionBar = this.getSupportActionBar();
actionBar.setTitle(R.string.fragment_title_grandstats);
// Display the back arrow
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
}
// Back arrow click event to go to the parent Activity
@Override
public boolean onSupportNavigateUp() {
onBackPressed();
return true;
}
}
回答11:
This worked perfectly
public class BackButton extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.chat_box);
Toolbar chatbox_toolbar=(Toolbar)findViewById(R.id.chat_box_toolbar);
chatbox_toolbar.setTitle("Demo Back Button");
chatbox_toolbar.setTitleTextColor(getResources().getColor(R.color.white));
setSupportActionBar(chatbox_toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
chatbox_toolbar.setNavigationOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Define Back Button Function
}
});
}
}
回答12:
In your manifest file for the activity where you want to
add a back button, we will use the property android:parentActivityName
<activity
android:name=".WebActivity"
android:screenOrientation="portrait"
android:parentActivityName=".MainActivity"
/>
P.S. This attribute was introduced in API Level 16.
回答13:
Simple and easy way to show back button on toolbar
Paste this code in onCreate method
if (getSupportActionBar() != null){
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
}
Paste this override method outside the onCreate method
@Override
public boolean onOptionsItemSelected(MenuItem item) {
if(item.getItemId()== android.R.id.home) {
finish();
}
return super.onOptionsItemSelected(item);
}
回答14:
Easily you can do it.
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
@Override
public boolean onSupportNavigateUp() {
onBackPressed();
return true;
}
Credits:
https://freakycoder.com/android-notes-24-how-to-add-back-button-at-toolbar-941e6577418e
回答15:
In Kotlin it would be
private fun setupToolbar(){
toolbar.title = getString(R.string.YOUR_TITLE)
setSupportActionBar(toolbar)
supportActionBar?.setDisplayHomeAsUpEnabled(true)
supportActionBar?.setDisplayShowHomeEnabled(true)
}
// don't forget click listener for back button
override fun onSupportNavigateUp(): Boolean {
onBackPressed()
return true
}
回答16:
Add this to activity's xml in layout folder:
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.v7.widget.Toolbar
android:id="@+id/prod_toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:popupTheme="@style/AppTheme.PopupOverlay" />
</android.support.design.widget.AppBarLayout>
Make toolbar clickable, add these to onCreate method:
Toolbar toolbar = (Toolbar) findViewById(R.id.prod_toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
toolbar.setNavigationOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});