I am trying to generate 10 pairs of plots with a few pairs per page of plots, and am using a for
loop to construct the pairs. However, the plots are sent to the device as separate plots instead of pages.
The MWE below has identical constructions for base graphics and ggplot
versions, but the base graphics works and ggplot
does not. What do I need to do to get the pagination correct in the second version?
library(ggplot2)
attach(mtcars)
# correct configuration
par(mfrow=c(2,2))
for (ii in 1:3){
vars <- c("wt", "disp", "wt")
plot(get(vars[ii]), mpg)
hist(get(vars[ii]))
}
# places each on separate plot
par(mfrow=c(2,2))
for (ii in 1:3){
vars <- c("wt", "disp", "wt")
p <- ggplot(mtcars, aes(get(vars[ii]), mpg)) + geom_point(size=4)
plot(p)
p <- ggplot(mtcars, aes(get(vars[ii]))) + geom_histogram()
plot(p)
}
detach(mtcars)
Here is one way to do it with cowplot::plot_grid
. The plot_duo
function uses tidyeval
approach in ggplot2 v3.0.0
# install.packages("ggplot2", dependencies = TRUE)
library(rlang)
library(dplyr)
library(ggplot2)
library(cowplot)
plot_duo <- function(df, plot_var_x, plot_var_y) {
if (is.character(plot_var_x)) {
print('character column names supplied, use rlang::sym()')
plot_var_x <- rlang::sym(plot_var_x)
} else {
print('bare column names supplied, use dplyr::enquo()')
plot_var_x <- enquo(plot_var_x)
}
if (is.character(plot_var_y)) {
plot_var_y <- rlang::sym(plot_var_y)
} else {
plot_var_y <- enquo(plot_var_y)
}
pts_plt <- ggplot(df, aes(x = !! plot_var_x, y = !! plot_var_y)) + geom_point(size = 4)
his_plt <- ggplot(df, aes(x = !! plot_var_x)) + geom_histogram()
duo_plot <- plot_grid(pts_plt, his_plt, ncol = 2)
}
### use character column names
plot_vars1 <- c("wt", "disp", "wt")
plt1 <- plot_vars1 %>% purrr::map(., ~ plot_duo(mtcars, .x, "mpg"))
#> [1] "character column names supplied, use rlang::sym()"
#> `stat_bin()` using `bins = 30`. Pick better value with `binwidth`.
#> [1] "character column names supplied, use rlang::sym()"
#> `stat_bin()` using `bins = 30`. Pick better value with `binwidth`.
#> [1] "character column names supplied, use rlang::sym()"
#> `stat_bin()` using `bins = 30`. Pick better value with `binwidth`.
plot_grid(plotlist = plt1, nrow = 3)
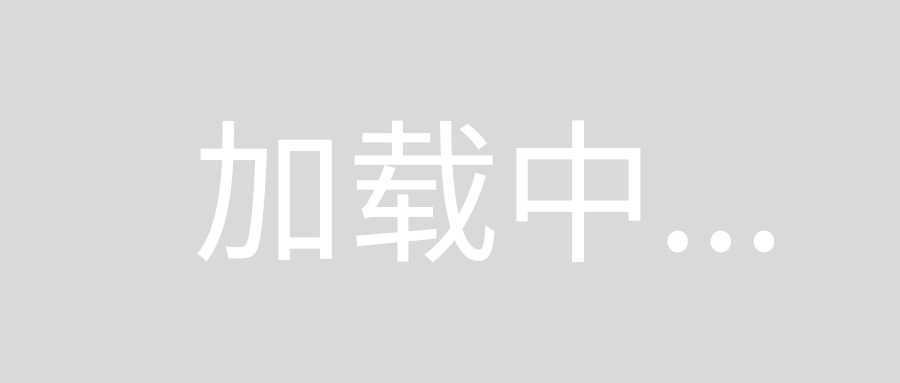
### use bare column names
plot_vars2 <- alist(wt, disp, wt)
plt2 <- plot_vars2 %>% purrr::map(., ~ plot_duo(mtcars, .x, "mpg"))
#> [1] "bare column names supplied, use dplyr::enquo()"
#> `stat_bin()` using `bins = 30`. Pick better value with `binwidth`.
#> [1] "bare column names supplied, use dplyr::enquo()"
#> `stat_bin()` using `bins = 30`. Pick better value with `binwidth`.
#> [1] "bare column names supplied, use dplyr::enquo()"
#> `stat_bin()` using `bins = 30`. Pick better value with `binwidth`.
plot_grid(plotlist = plt2, nrow = 3)
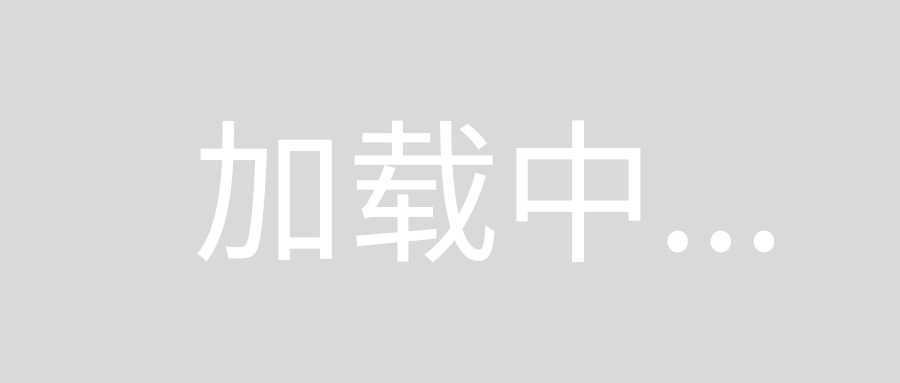
To separate plots into multiple pages, we can use gridExtra::marrangeGrob
ml1 <- marrangeGrob(plt, nrow = 2, ncol = 1)
# Interactive use
ml1
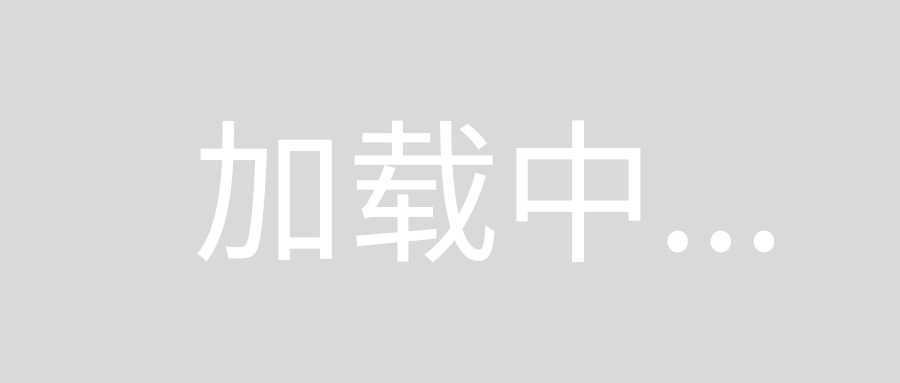
# Non-interactive use, multipage pdf
ggsave("multipage.pdf", ml1)