This question already has an answer here:
-
VBA Excel Getting File Path (ends with folder)
6 answers
I have a small Access VBA application that requires users to select a folder. I was wondering if there is a way to tell VBA the path at which to start the folder picker. i.e. start the folder picker at C:\data\forms
. Currently it seems to be starting from the directory that was previously used. Also is there a way to limit what the folder picker can access. So it can access anything within C:\data
but not anything else in C:
I have been using the following code (Not My Code) successfully for many years.
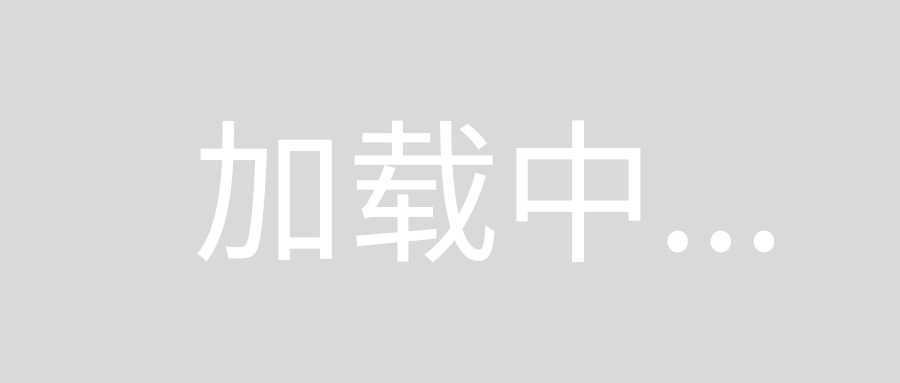
Sub Sample()
Dim Ret
'~~> Specify your start folder here
Ret = BrowseForFolder("C:\")
End Sub
Function BrowseForFolder(Optional OpenAt As Variant) As Variant
'Function purpose: To Browser for a user selected folder.
'If the "OpenAt" path is provided, open the browser at that directory
'NOTE: If invalid, it will open at the Desktop level
Dim ShellApp As Object
'Create a file browser window at the default folder
Set ShellApp = CreateObject("Shell.Application"). _
BrowseForFolder(0, "Please choose a folder", 0, OpenAt)
'Set the folder to that selected. (On error in case cancelled)
On Error Resume Next
BrowseForFolder = ShellApp.self.Path
On Error GoTo 0
'Destroy the Shell Application
Set ShellApp = Nothing
'Check for invalid or non-entries and send to the Invalid error
'handler if found
'Valid selections can begin L: (where L is a letter) or
'\\ (as in \\servername\sharename. All others are invalid
Select Case Mid(BrowseForFolder, 2, 1)
Case Is = ":"
If Left(BrowseForFolder, 1) = ":" Then GoTo Invalid
Case Is = "\"
If Not Left(BrowseForFolder, 1) = "\" Then GoTo Invalid
Case Else
GoTo Invalid
End Select
Exit Function
Invalid:
'If it was determined that the selection was invalid, set to False
BrowseForFolder = False
End Function
Here is a quick and dirty method I use all the time. The function below will only get the user to select the folder they want to start at - I think the simplest way to limit access to a given path is to perhaps check GetFolderName
below against the path(s) you want to restrict e.g.
If GetFolderName = "C:\" then
MsgBox("This folder is not for you buddy")
Exit Sub
end if
Also not my code :)
Public Function GetFolderName(Optional OpenAt As String) As String
Dim lCount As Long
GetFolderName = vbNullString
With Application.FileDialog(msoFileDialogFolderPicker)
.InitialFileName = OpenAt
.Show
For lCount = 1 To .SelectedItems.Count
GetFolderName = .SelectedItems(lCount)
Next lCount
End With
End Function
If you do not need to restrict the folder-view to your user, then I would suggest using the FileDialog method (the interface is more intuitive then what invoking the shell gives you). For additional details, you can read more on CPearson's site. He has a lengthy article on browsing for folders using VBA (mulitple ways; the FileDialog option is at the very end):
Function BrowseFolder(Title As String, _
Optional InitialFolder As String = vbNullString, _
Optional InitialView As Office.MsoFileDialogView = _
msoFileDialogViewList) As String
Dim V As Variant
Dim InitFolder As String
With Application.FileDialog(msoFileDialogFolderPicker)
.Title = Title
.InitialView = InitialView
If Len(InitialFolder) > 0 Then
If Dir(InitialFolder, vbDirectory) <> vbNullString Then
InitFolder = InitialFolder
If Right(InitFolder, 1) <> "\" Then
InitFolder = InitFolder & "\"
End If
.InitialFileName = InitFolder
End If
End If
.Show
On Error Resume Next
Err.Clear
V = .SelectedItems(1)
If Err.Number <> 0 Then
V = vbNullString
End If
End With
BrowseFolder = CStr(V)
End Function
This function takes two parameters. The first, Title is a string specifying the title to be displayed with the file dialog. The second InitialFolder, which is optional, specifies the initial folder to which the dialog should open. The third parameter, also optional, InitialView specifies the view type. See MsoFileDialogView in the Object Browser for the valid values of this parameter. The function returns the fully-qualified folder name selected by the user or an empty string if the user cancelled the dialog.
Here is a much simpler way. This code snippet lets user pick a folder and then prints that folder address to the screen:
Sub PrintSelectedFolder()
Dim selectedFolder
With Application.FileDialog(msoFileDialogFolderPicker)
.Show
selectedFolder = .SelectedItems(1)
End With
'print to screen the address of folder selected
MsgBox (selectedFolder)
End Sub
For mac users:
Sub Select_Folder_On_Mac()
Dim folderPath As String
Dim RootFolder As String
On Error Resume Next
RootFolder = MacScript("return (path to desktop folder) as String")
'Or use RootFolder = "Macintosh HD:Users:YourUserName:Desktop:TestMap:"
folderPath = MacScript("(choose folder with prompt ""Select the folder""" & _
"default location alias """ & RootFolder & """) as string")
On Error GoTo 0
If folderPath <> "" Then
MsgBox folderPath
End If
End Sub
Stolen from http://www.rondebruin.nl/mac/mac017.htm ;)