I'm using ActionBarCompat in my app, an I want to show one or two items in the action bar
I follwed the guide of google developers, but when I test it, the items are showed in the "Overflow" option (in Nexus 4) and if I tap on the menu button if there exist (ex. Galaxy S3)
What I've doing wrong?
SOLUTION FOUND
You can find it in a answer.
I had the same problem, and found two solutions:
In the menu xml (Login.xml), use your app name for the showAsAction tag:
instead of:
<item
android:id="@+id/action_register"
android:showAsAction="always"
android:icon="@drawable/some_icon"
android:title="@string/login_menu_register" />
use:
<item
android:id="@+id/action_register"
yourappname:showAsAction="always"
android:icon="@drawable/some_icon"
android:title="@string/login_menu_register" />
I suppose your application's name is shady.
the second solution for me, on the activity class, at the onCreateOptionsMenu()
public boolean onCreateOptionsMenu(Menu menu) {
super.onCreateOptionsMenu(
getMenuInflater().inflate(R.menu.main, menu);
MenuItem registerMenuItem = menu.findItem(R.id.action_register);
registerMenuItem.setShowAsAction(MenuItem.SHOW_AS_ACTION_ALWAYS); // change this in backcompat
return true;
}
if you are using backCompatibility, change last line:
MenuItemCompat.setShowAsAction(registerMenuItem,MenuItemCompat.SHOW_AS_ACTION_ALWAYS);
I think you need to provide the icon for these actions so they can appear in the action bar.
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:shudy="http://schemas.android.com/apk/res-auto" >
<item
android:id="@+id/action_register"
android:showAsAction="always"
android:icon="@drawable/some_icon"
android:title="@string/login_menu_register" />
<item
android:id="@+id/action_register2"
android:showAsAction="always"
android:icon="@drawable/some_icon2"
android:title="miau" />
</menu>
The question has been updated to include the answer, but for anyone who is interested in the official documentation, see http://developer.android.com/guide/topics/ui/actionbar.html#ActionItems and pay particular attention to the note about using a custom namespace for the showAsAction
attribute.
<item
android:id="@+id/ok"
android:icon="@drawable/ic_ok"
android:orderInCategory="0"
android:showAsAction="ifRoom"
android:title="OK"/>
We have two ways to solute this problem.
- You need add libs "ActionBarSherlock".This is usage of ActionbarSherlock.
OR
2. You need add libs "android-Support-v7". If you choice this method ,then your menu.xml need like this:
<?xml version="1.0" encoding="UTF-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:v7="http://schemas.android.com/apk/res-auto" >
<item
android:id="..."
android:icon="..."
android:showAsAction="always"
android:title="..."
v7:showAsAction="always"/>
</menu>
This is also true for the Android Toolbar in new Material Design concepts in the AppCompat-v21 and API levels 21 and above.
I found the solution so I posted as Answer:
SOLUTION FOUND
In the xml of the menu, you have to put the new namespace, to make actionbarcompat work. So there are some options that need this spacename instead of "android". So the solutions is This:
Old Login menu:
<item
android:id="@+id/action_register"
android:showAsAction="always"
android:title="@string/login_menu_register"/>
New Login Menu (solution) (Look at how is caled "showAsAction")
<item
android:id="@+id/action_register"
shudy:showAsAction="always"
android:title="@string/login_menu_register"/>
Login.xml (MENU)
<item
android:id="@+id/action_register"
android:showAsAction="always"
android:title="@string/login_menu_register"/>
<item
android:id="@+id/action_register2"
android:showAsAction="always"
android:title="miau"/>
LoginActivity.java
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
SLog.d(CLASS_NAME + " onCreate()");
setContentView(R.layout.activity_login);
actionBar = getSupportActionBar();
actionBar.setDisplayHomeAsUpEnabled(true);
actionBar.setHomeButtonEnabled(true);
findViews();
buttons();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.login, menu);
return true;
}
Manifest
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/Theme.AppCompat.Light.DarkActionBar" >
<activity
android:name="com.shudy.myworld.LoginActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
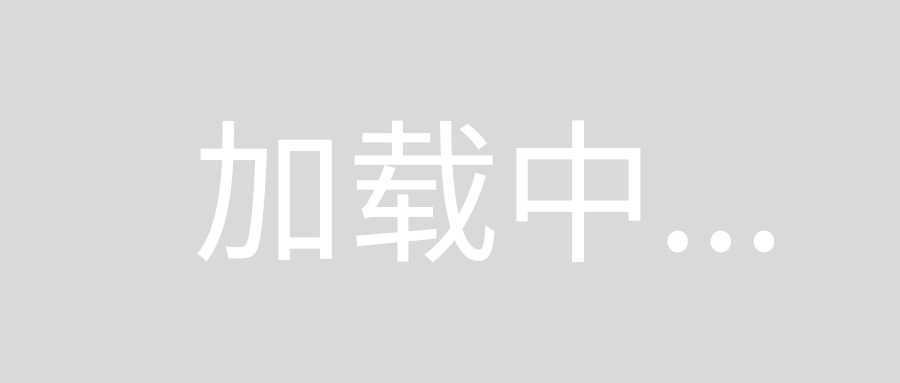