Here's a print screen of my software:
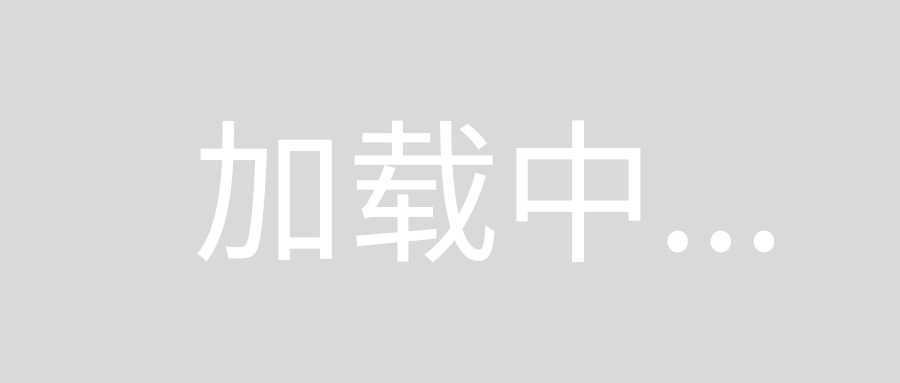
As you can see, the first QTableVIew
headers do not take 100% of the width. In fact, there is a small vertical white space on the right of the field size
.
How can I get the headers to take 100% of the width of the QTableView
?
If you are using Qt 5, QHeaderView::setResizeMode()
is no longer available. Instead, you can use QHeaderView::setSectionResizeMode()
. Just call it for every column:
for (int c = 0; c < ui->tableView->horizontalHeader()->count(); ++c)
{
ui->tableView->horizontalHeader()->setSectionResizeMode(
c, QHeaderView::Stretch);
}
Use view->horizontalHeader()->setStretchLastSection(true)
to make the last column expand to free space.
Additionally, use view->horizontalHeader()->setResizeMode(QHeaderView::Stretch)
to give columns the same width.
Here works using only with:
ui->tableView->horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch);
I'm using Qt 5.2!
I had a hard time distributing column widths among all cells of a table. In my case, in headerData function of the model, I did the following (requires calling resizeColumnsToContents() somewhere):
QVariant headerData(int section, Qt::Orientation orientation, int role) const override {
if (orientation == Qt::Vertical) {
return QVariant();
}
if (role == Qt::SizeHintRole) {
auto* p = qobject_cast<QTableView*>(QObject::parent());
if (p == nullptr) return QVariant();
// Parent total width.
const int w = p->viewport()->size().width() -
p->verticalScrollBar()->sizeHint().width();
QSize qs;
// Default height.
qs.setHeight(p->verticalHeader()->defaultSectionSize());
// Width per column.
switch (section) {
case 0:
qs.setWidth(w * 0.45);
return QVariant(qs);
case 1:
qs.setWidth(w * 0.45);
return QVariant(qs);
// ... others
default: ;
}
return QVariant();
}
if (role == Qt::DisplayRole) {
// header titles.
}
}