i'm currently using epson ePOS SDK for android.
i need to print the receipt that menu name align to the left and its price align to the right in the same line but it doesn't work properly,
my temporary solution is add some feed line to make its price align right, is it possible to have both text align left and right in the same line ?
(Attachments below and please ignore question mark symbols)
mPrinter.addTextAlign(Printer.ALIGN_LEFT);
mPrinter.addFeedLine(0);
textData.append(menuName);
mPrinter.addText(textData.toString());
textData.delete(0, textData.length());
mPrinter.addFeedLine(0);
//print price
mPrinter.addTextAlign(Printer.ALIGN_RIGHT);
textData.append(price + "Y" + "\n");
mPrinter.addText(textData.toString());
textData.delete(0, textData.length());
mPrinter.addFeedLine(0);
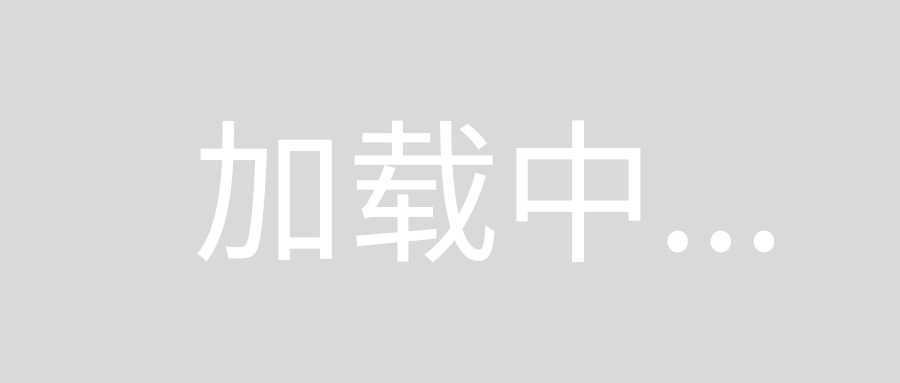
80mm is like 42 columns per line ...which can be easily padded:
mPrinter.addText(padLine(menuName, price + "¥", 42) + "\n");
the required String
manipulation methods look alike:
/** utility: pads two strings to columns per line */
protected String padLine(@Nullable String partOne, @Nullable String partTwo, int columnsPerLine){
if(partOne == null) {partOne = "";}
if(partTwo == null) {partTwo = "";}
String concat;
if((partOne.length() + partTwo.length()) > columnsPerLine) {
concat = partOne + " " + partTwo;
} else {
int padding = columnsPerLine - (partOne.length() + partTwo.length());
concat = partOne + repeat(" ", padding) + partTwo;
}
return concat;
}
/** utility: string repeat */
protected String repeat(String str, int i){
return new String(new char[i]).replace("\0", str);
}
one should format the price to currency, before padding it.
to make it truly "flawless" ... when String concat
exceeds length 42
, then String partOne
should be truncated by the excess length - and be concatenated, once again. exceeding int columnsPerLine
will most likely mess up the output.
Inserting the RIGHT string in the LEFT string at an appropriate position. This is because I'm not using a feedLine provided by EPSON. Instead I'm manually adding a new line(\n) after 42(this varies according to the printer) characters.
// gap -> Number of spaces between the left and the right string
public void print(Printer epsonPrinter, String left, String right, int gap) throws Epos2Exception {
int total = 42;
// This is to give a gap between the 2 columns
for (int i = 0; i < gap; i++) {
right = " " + right;
}
// This will make sure that the right string is pushed to the right.
// If the total sum of both the strings is less than the total length(42),
// we are prepending extra spaces to the RIGHT string so that it is pushed to the right.
if ((left.length() + right.length()) < total) {
int extraSpace = total - left.length() - right.length();
for (int i = 0; i < extraSpace; i++) {
left = left + " ";
}
}
int printableSpace = total - right.length(); // printable space left for the LEFT string
//stringBuilder -> is the modified string which has RIGHT inserted in the LEFT at the appropriate position. And also contains the new line. This is the string which needs to be printed
StringBuilder stringBuilder = new StringBuilder();
// Below 2 statements make the first line of the print
stringBuilder.append(left.substring(0, Math.min(printableSpace, left.length())));
stringBuilder.append(right);
// stringBuilder now contains the first line of the print
// For appropriately printing the remaining lines of the LEFT string
for (int index = printableSpace; index < left.length(); index = index + (printableSpace)) {
stringBuilder.append(left.substring(index, Math.min(index + printableSpace, left.length())) + "\n");
}
epsonPrinter.addText(stringBuilder.toString());
}