I have an app with a Navigation View for my DrawerLayout. I add programmatically items in the menu because I receive the information over the network. But sometimes, an item name can be very long and is just cut off with even having the ellipsize icon "..."
Does someone have an idea on how to have multiline for my menu items?
Thanks
Override design_navigation_menu_item.xml from the Android Support Design Library and modify the things you need (set android:ellipsize="end"
and android:maxLines="2"
)
Your res/layout/design_navigation_menu_item.xml should look like this:
<?xml version="1.0" encoding="utf-8"?>
<merge xmlns:android="http://schemas.android.com/apk/res/android">
<CheckedTextView
android:id="@+id/design_menu_item_text"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:drawablePadding="@dimen/design_navigation_icon_padding"
android:gravity="center_vertical|start"
android:textAppearance="@style/TextAppearance.AppCompat.Body2"
android:ellipsize="end"
android:maxLines="2" />
<ViewStub
android:id="@+id/design_menu_item_action_area_stub"
android:inflatedId="@+id/design_menu_item_action_area"
android:layout="@layout/design_menu_item_action_area"
android:layout_width="wrap_content"
android:layout_height="match_parent" />
</merge>
However, you should just ellipsize the text to properly follow the Material Design guidelines.
You have not to override anything to just ellipsize the text:
- Add a new style to the styles.xml
- Set the new style to the
NavigationView
res / values / styles.xml
<style name="TextAppearance">
<item name="android:ellipsize">end</item>
</style>
res / layout / activity_main.xml
<android.support.design.widget.NavigationView
android:id="@+id/nav_view"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
android:fitsSystemWindows="true"
app:headerLayout="@layout/nav_header_main"
app:menu="@menu/activity_main_drawer"
app:theme="@style/TextAppearance" />
Check out this answer. Default android slide menu is not very flexible, most likely you will have to create your custom view.
I really this tutorial from AndroidHive.
I had the same problem and I tried and tried, There was no progress with Menu
, I don't say it was impossible but I couldn't find a way to break MenuItem
lines.
But if you don't insist on using Menu
I suggest you to use a ListView
or RecyclerView
in your layout like this:
<android.support.design.widget.NavigationView
android:id="@+id/nav_view"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
app:headerLayout="@layout/nav_draw_header">
<android.support.v7.widget.RecyclerView
android:id="@+id/rv"
android:layout_marginTop="160dp"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</android.support.design.widget.NavigationView>
As you can see there is no special thing to explain, no menu, just a RecyclerView
, layout_marginTop
value is same as header height, I'm sure you know the rest of the story (How to fire up RecyclerView
), This approach gives more flexibility to NavigationView
menu items (actually RecyclerView
items).
If my answer is not clear enough to you let me know.
Here is the result:
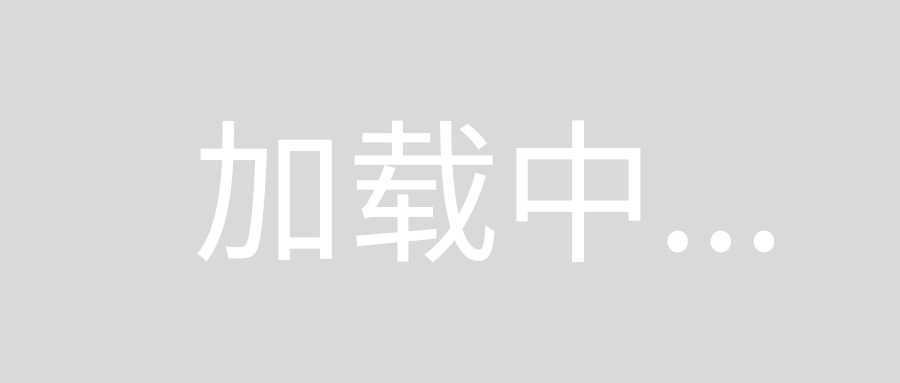
You shouldn't change that.
Check out the material design guideline: https://material.io/design/components/navigation-drawer.html#anatomy