I used in Delphi QuickReport to create reports and print. What can I use to do this in .NET C#?
I added some reporting elements (Microsoft reports and Crystal reports) to my project (Winforms app), but what I saw, is that I can only insert data from a Database. What I want, is to use the values of objects created in runtime. This is because my reports actually consists of receipts and invoices.
Which is the best tool to use for my need?
You can use the built in reports to generate nice reports wihtout requiring a database.
Create a class for your data, in my case, I am going to create a person class:
class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string AddressLine1 { get; set; }
public string AddressLine2 { get; set; }
}
Next I am going to add a report using the report wizard (Add New Item -> Reporting -> Report Wizard).
For the datasource, I am going to select Object and then point it to my Person class.
Select the columns you want for your details, I am just dragging all of them into the values for simplicity.
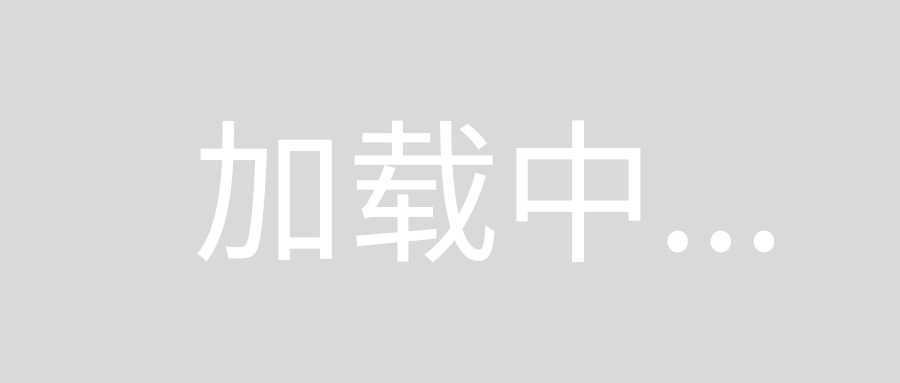
Walk through the rest of the wizard just selecting the defaults and you should then see your report.
Now you can add a ReportViewer control to a form and set the report to the report you just created. This should create a PersonBindingSource on your form as well.
Set the PersonBindingSource's data to a list in memory:
BindingList<Person> myPeople = new BindingList<Person>();
myPeople.Add(new Person() { FirstName = "John" , LastName = "Doe"});
myPeople.Add(new Person() { FirstName = "Jane" , LastName = "Doe"});
myPeople.Add(new Person() { FirstName = "Jerry" , LastName = "Smithers" });
PersonBindingSource.DataSource = myPeople;
reportViewer1.RefreshReport();
this.reportViewer1.RefreshReport();
With the final report looking like this:
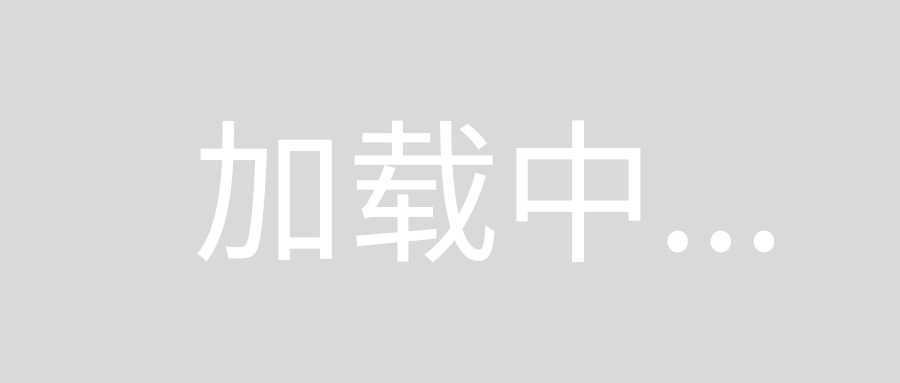
Crystal Reports will work perfectly for you. Actually you can generate reports without a database. Check out this project and it should get you started and is just like what you are trying to do.
You this helps you!