可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
How to set status bar color when AppBar not present.
I have tried this but not working.
Widget build(BuildContext context) {
SystemChrome.setSystemUIOverlayStyle(SystemUiOverlayStyle.dark);
return new Scaffold(
body: new Container(
color: UniQueryColors.colorBackground,
child: new ListView.builder(
itemCount: 7,
itemBuilder: (BuildContext context, int index){
if (index == 0){
return addTopInfoSection();
}
},
),
),
);
}
Output look like this:
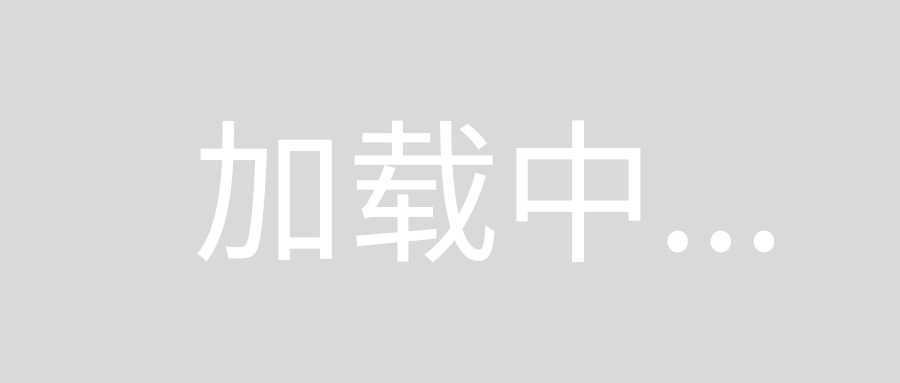
回答1:
Simply put this in the build function of your App:
SystemChrome.setSystemUIOverlayStyle(SystemUiOverlayStyle.dark.copyWith(
statusBarColor: Colors.blue, //or set color with: Color(0xFF0000FF)
));
Additionally you can set useful variables like: statusBarIconBrightness
, systemNavigationBarColor
or systemNavigationBarDividerColor
回答2:
On Android, add the following to onCreate in MainActivity.java, after the call to super.onCreate(savedInstanceState);
getWindow().setStatusBarColor(0x00000000);
or you can use the the flutter_statusbarcolor plugin
changeStatusColor(Color color) async {
try {
await FlutterStatusbarcolor.setStatusBarColor(color);
} on PlatformException catch (e) {
print(e);
}
}
Sample project
回答3:
While searching for SystemChrome I found this: https://docs.flutter.io/flutter/services/SystemChrome/setSystemUIOverlayStyle.html
Right above the sample code is a paragraph about AppBar.brightness
.
You should should be able to add an AppBar like this:
Widget build(BuildContext context) {
SystemChrome.setSystemUIOverlayStyle(SystemUiOverlayStyle.dark);
return new Scaffold(
appBar: new AppBar(
title: new Text('Nice title!'),
brightness: Brightness.light,
),
body: new Container(
color: UniQueryColors.colorBackground,
child: new ListView.builder(
itemCount: 7,
itemBuilder: (BuildContext context, int index){
if (index == 0){
return addTopInfoSection();
}
},
),
),
);
}
Heres info about the Brightness
回答4:
If you take a loot at the source code of AppBar, you can see they use a AnnotatedRegion widget.
From my understanding, this widget automatically sets the statusbar/navigationbar color when the widget wrapped in it gets overlaid by the statusbar/navigationbar.
You can wrap your widget like this:
import 'package:flutter/services.dart';
...
Widget build(BuildContext context) {
return Scaffold(
body: AnnotatedRegion<SystemUiOverlayStyle>(
value: SystemUiOverlayStyle.light,
child: ...,
),
);
}
回答5:
Widget build(BuildContext context) {
return new Scaffold(
body: new Container(
color: UniQueryColors.colorBackground,
/* Wrapping ListView.builder with MediaQuery.removePadding() removes the default padding of the ListView.builder() and the status bar takes the color of the app background */
child: MediaQuery.removePadding(
removeTop: true,
context: context,
child: ListView.builder(
itemCount: 7,
itemBuilder: (BuildContext context, int index){
if (index == 0){
return addTopInfoSection();
}
},
),
),
),
);
}
回答6:
The status bar colour is rendered by the Android system. Whether that can be set from Flutter or not is up for debate: How to make Android status bar light in Flutter
What you can do however, is change the status bar colour in the Android specific code by editing the theme: How to change the status bar color in android
For iOS you'll have to see their documentation - I'm not familiar with the platform.
There are in fact two Dart libraries, one for setting the light/dark theme of the statusbar and the other for setting the colour. I haven't used either, but clearly someone else has had the same issue you're facing and ended up developing their own package.
回答7:
return MaterialApp(
title: 'I Am A Student',
theme: ThemeData(
primarySwatch: AppColor.appColor,
primaryColorDark: AppColor.appColor,
primaryColorBrightness: Brightness.dark,
cursorColor: AppColor.appColor,
primaryColor: Color(0xFFF1F2F4),
buttonTheme: ButtonThemeData(buttonColor: AppColor.appColor,textTheme: ButtonTextTheme.primary),
fontFamily: 'Rubik',
),
// home: NewAddressScreen(),
home: SplashScreen(),
);
add cursorColor in theme data after that you'll see statusbar color