可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I am using Xcode 6 and implementing UIPageViewController
for my app. I followed appcoda's tutorial, and everything works except the page indicators. This is because I cannot set the transition style of UIPageViewController
to scroll.
Graphically, when I click on the PageViewController
, the tab shows View Controller instead of Page View Controller like appcoda
(See its image below)
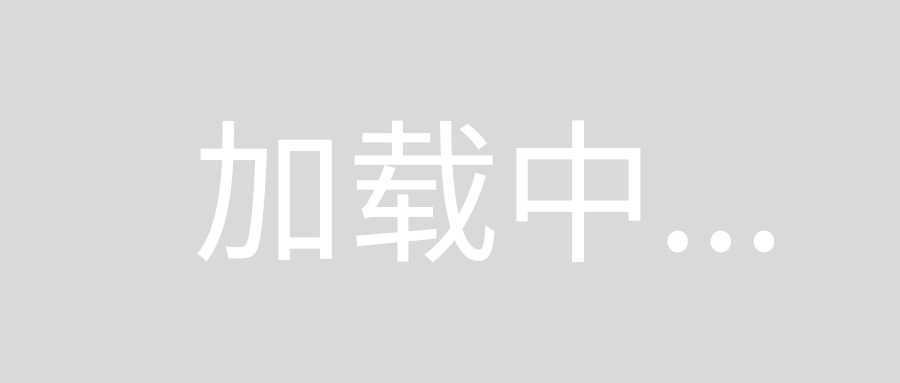
This is what mine looks like:
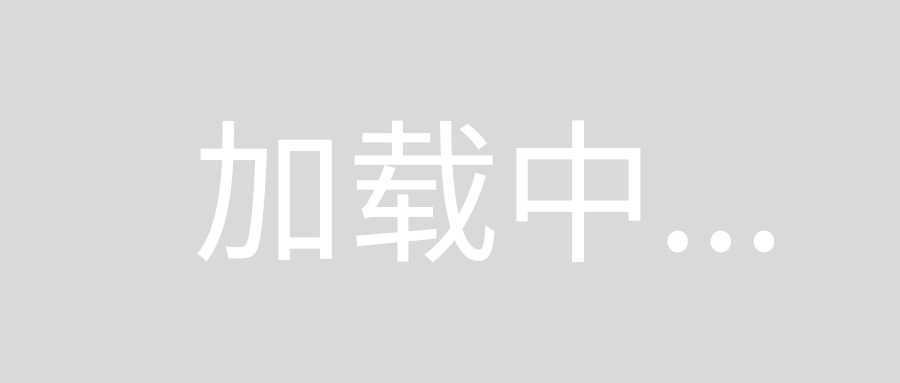
And yes, my custom class is set to: UIPageViewController
as it is in the tutorial.
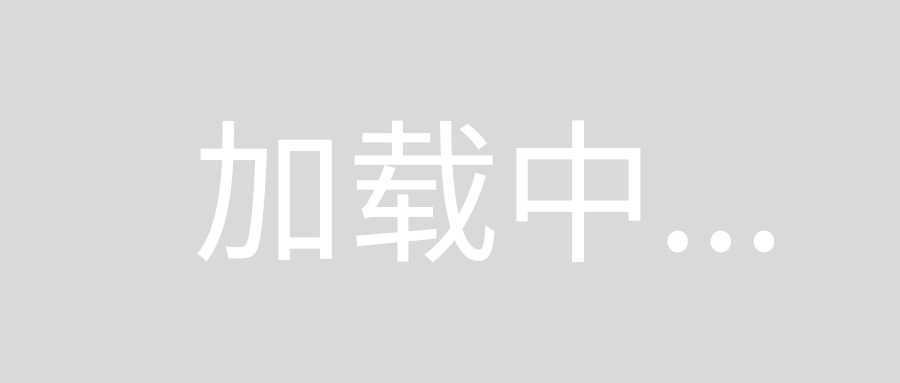
Programmatically, I try to set the transition style with:
self.pageViewController.transitionStyle = UIPageViewControllerTransitionStyle.Scroll
but it fails to build, telling me that it Could not find member transition style.
One last weird thing I would like to point out is that if I just write self.pageViewController.transitionStyle
, it builds successfully, but it still uses the Page Curl
Transition.
Could anyone help me? I am using Swift, Xcode 6, and developing on the iOS 8 SDK.
回答1:
You're getting an error because transitionStyle
is a readonly property. If you want to set the transition style of the page controller programmatically, it can only be done during initialization with the method:
init(transitionStyle style: UIPageViewControllerTransitionStyle, navigationOrientation navigationOrientation: UIPageViewControllerNavigationOrientation, options options: [NSObject : AnyObject]!) { ... }
More info in the documentation.
回答2:
Since nobody has answered the question. The issue is that when you create a UIPageViewController inside a Container, the container is first created with a UIViewController, and that s the controller you see your options for. In order to fix it you have to delete UIViewController where you set your custom UIPageViewController. Then drag UIPageViewController from the controls picker and hook up the embed segue from the container to that controller, then you can set your custom pager controller as well as set transition to scroll.
回答3:
Swift 3.0
required init?(coder aDecoder: NSCoder) {
super.init(transitionStyle: .scroll, navigationOrientation: .horizontal, options: nil)
}
回答4:
in ios 8 swift3
override init(transitionStyle style: UIPageViewControllerTransitionStyle, navigationOrientation: UIPageViewControllerNavigationOrientation, options: [String : Any]? = nil) {
super.init(transitionStyle: .scroll, navigationOrientation: .horizontal, options: options)
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
回答5:
Xcode 7.1 & Swift 2
All other solutions did not fully work for me at the latest Swift, especially since my class also inherits from UIPageViewControllerDataSource and UIPageViewControllerDelegate.
To set the PageViewController transition to .Scroll just add these 2 methods to your class:
override init(transitionStyle style: UIPageViewControllerTransitionStyle, navigationOrientation: UIPageViewControllerNavigationOrientation, options: [String : AnyObject]?) {
super.init(transitionStyle: .Scroll, navigationOrientation: .Horizontal, options: options)
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
回答6:
This is how i solve this problem in Swift 2.0
1. Created a class which is subclass of UIPageViewController
import UIKit
class OPageViewController: UIPageViewController {
override init(transitionStyle style: UIPageViewControllerTransitionStyle, navigationOrientation: UIPageViewControllerNavigationOrientation, options: [String : AnyObject]?) {
// Here i changed the transition style: UIPageViewControllerTransitionStyle.Scroll
super.init(transitionStyle: UIPageViewControllerTransitionStyle.Scroll, navigationOrientation: UIPageViewControllerNavigationOrientation.Horizontal, options: options)
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func viewDidLoad() {
super.viewDidLoad()
}
}
2.Than i just used it :D
pageController = OPageViewController()
pageController.dataSource = self
pageController.setViewControllers(NSArray(object: vc) as? [UIViewController], direction: .Forward, animated: true, completion: nil)
pageController.view.frame = CGRectMake(0, container.frame.origin.y, view.frame.size.width, container.frame.size.height);
回答7:
I know this a little bit late, but I just had the same problem and realized that it was originated because I was using a simple UIViewController, even when my custom view controller class inherits from an UIPageViewController, aparently the graphic menu of xcode doesn't recognize it.
The solution was to delete in story board the view controller and drag a PageViewController to replace it. This will have the graphic menu with the scroll option.
回答8:
Easiest way to do this programmatically in Swift 2.0 (without subclassing):
class PageViewController: UIPageViewController {
override init(transitionStyle style: UIPageViewControllerTransitionStyle, navigationOrientation navigationOrientation: UIPageViewControllerNavigationOrientation, options options: [NSObject : AnyObject]!) {
super.init(transitionStyle: .Scroll, navigationOrientation: .Horizontal, options: options)
}
}
回答9:
Swift 4 programmatically set the transition style of UIPageViewController
This is straight from 'UIPageViewController.h' line 61-97
Just put this before viewDidLoad() in your UIPageViewController() class
override init(transitionStyle style:
UIPageViewControllerTransitionStyle, navigationOrientation: UIPageViewControllerNavigationOrientation, options: [String : Any]? = nil) {
super.init(transitionStyle: .scroll, navigationOrientation: .horizontal, options: nil)
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
回答10:
This is a known bug with UIPageViewController
You can read an explanation of the cause in this SO question and more comprehensively in this one.
I used the AppCoda Tutorial as well to get a handle on PageViewControllers. Another SO user actually wrote a gist that solved the problem for me in a way that I didn't have to change a line of code in my own project. Just drop AVC in to your project and call that instead of UIPageViewController in your code.
Paul de Lange's AlzheimerViewController.
回答11:
swift 3 xcode 8 in
class ...: UIPageViewController {
override var transitionStyle: UIPageViewControllerTransitionStyle {
return .scroll
}
override var navigationOrientation: UIPageViewControllerNavigationOrientation {
return .horizontal
}
}