Are there any tools or Visual Studio 2010 extensions which allow me to view the output of a configuration file transformation short of having to publish the entire project? Is the process which performs the transformation directly invokable?
Edit
After a little more Googling I came across this:
Step 4: Generating a new transformed web.config file for “Staging” environment from command line
Open Visual Studio Command prompt by
going to Start --> Program Files –>
Visual Studio v10.0 –> Visual Studio
tools –> Visual Studio 10.0 Command
Prompt
Type “MSBuild “Path to Application
project file (.csproj/.vbproj) ”
/t:TransformWebConfig
/p:Configuration=Staging" and hit
enter as shown below:
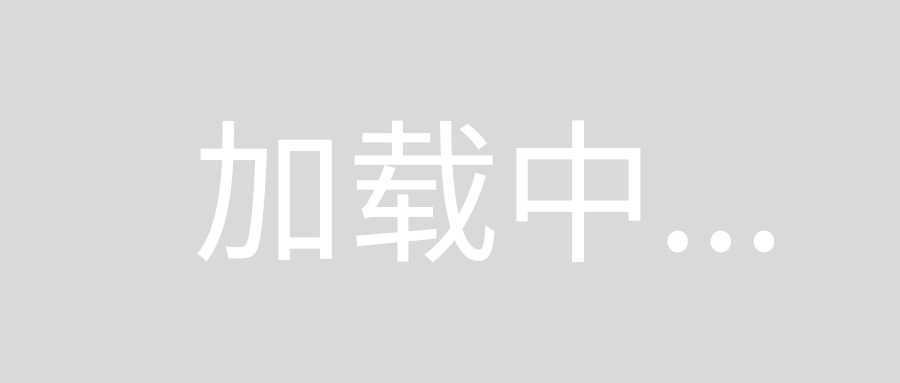
Once the transformation is successful
the web.config for the “Staging”
configuration will be stored under obj
-->Staging folder under your project root (In solution explorer you can
access this folder by first un-hiding
the hidden files) :
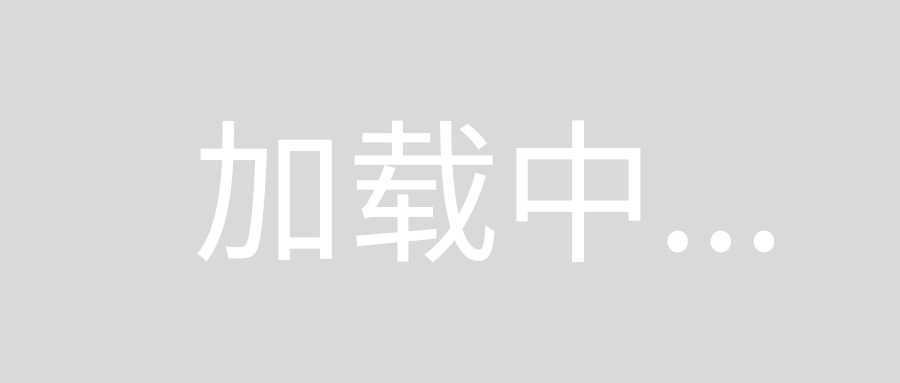
- In the solution explorer click the button to show hidden files
- Open the Obj folder
- Navigate to your Active configuration (in our current case it is “Staging”)
- You can find the transformed web.config there
You can now verify that the new
staging web.config file generated has
the changed connection string section.
Source: Web Deployment: Web.Config Transformation
This isn't really a perfect solution for me as it still requires building the entire project- at least with the command he posted. If anyone knows of way to skip the build step with the MSBuild command that would be helpful (although that sounds somewhat unlikely).
Edit 2
I also found this Config Transformation Tool on CodePlex, which offers some nice functionality to extend the transformation process. This is tool is the closest thing I've seen for the functionality I'm seeking and would be a great starting point for developing an extension which creates previews. It uses the Microsoft.Web.Publishing.Tasks library to perform the transformation and does not depend on building an actual project.
The SlowCheetah VS add-in on the visualstudiogallery allows you to preview the transform results
You can transform a config file by using the same objects the MSBuild task uses, bypassing MSBuild altogether. Web config transform logic is contained in the Microsoft.Web.Publishing.Tasks library.
The following code snippet comes from a simple class library, referencing the Microsoft.Web.Publishing.Tasks library (which is installed on my machine at C:\Program Files (x86)\MSBuild\Microsoft\VisualStudio\v10.0\Web).
The sample loads a source document and transform, applies the transform, and writes the results to a new file.
using System;
using Microsoft.Web.Publishing.Tasks;
// ...
var xmlTarget = new XmlTransformableDocument();
xmlTarget.PreserveWhitespace = true;
xmlTarget.Load("Web.config");
var xmlTransform = new XmlTransformation("Web.Release.config");
if (xmlTransform.Apply(xmlTarget))
xmlTarget.Save("Web.Transformed.config");
else
Console.WriteLine("Unable to apply transform.");
With a little creativity, this simple solution could be integrated into a Visual Studio plugin, perhaps as a context menu item on the web.config file. At the very least, you can make a console utility or script out of it to generate previews.
Good luck!
Old post, but thought I would share what I had found with a quick google (for those that may not have found it or tried here first):
Web.config Transformation Tester - By AppHarbor
Simply paste your original XML along with the transformation XML and see the result instantaneously.
Also, it's open source for anyone who's interested.
Just to extend on this a little.
I needed exactly what is discussed above. To be able to run the transform only.
Then hook that into my build process which happens to be TeamCity in my case.
You will need using Microsoft.Web.Publishing.Tasks, which you can just smash down with Nuget. Well, I was in VS2013 so I could. I'm sure you could acquire the dll otherwise.
Wrote a simple Console App. You may find it useful.
Program.cs
using System;
namespace WebConfigTransform
{
class Program
{
static void Main(string[] args)
{
if (args.Length != 3)
{
Console.WriteLine("Config Gen ... usage -source -transform -destination");
Environment.Exit(-1);
}
Transform t = new Transform(args[0], args[1], args[2]);
t.Run();
}
}
}
Transform.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Security;
using System.Security.Permissions;
using Microsoft.Web.XmlTransform;
namespace WebConfigTransform
{
class Transform
{
private readonly string m_source;
private readonly string m_transform;
private readonly string m_destination;
public Transform(string source, string transform, string destination)
{
m_source = source;
m_transform = transform;
m_destination = destination;
}
private void TransformFiles()
{
var xmlTarget = new XmlTransformableDocument();
xmlTarget.PreserveWhitespace = true;
xmlTarget.Load(m_source);
var xmlTransform = new XmlTransformation(m_transform);
if (xmlTransform.Apply(xmlTarget))
xmlTarget.Save(m_destination);
else
{
Console.WriteLine("Unable to apply transform.");
Environment.Exit(-1);
}
}
private void CheckPermissions()
{
string directoryName = m_destination;
PermissionSet permissionSet = new PermissionSet(PermissionState.None);
FileIOPermission writePermission = new FileIOPermission(FileIOPermissionAccess.Write, directoryName);
permissionSet.AddPermission(writePermission);
if (!(permissionSet.IsSubsetOf(AppDomain.CurrentDomain.PermissionSet)))
{
Console.WriteLine("Cannot write to file : " + m_destination);
Environment.Exit(-1);
}
}
private void CheckFileExistance()
{
List<string> ls = new List<string>();
ls.Add(m_source);
ls.Add(m_transform);
foreach (string item in ls)
{
if (!File.Exists(item))
{
Console.WriteLine("Cannot locate file : " + item);
Environment.Exit(-1);
}
}
}
public void Run()
{
CheckFileExistance();
CheckPermissions();
TransformFiles();
}
}
}