可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I am trying to set up a hello world example with AWS lambda and serving it through api gateway. I clicked the "Create a Lambda Function", which set up the api gatway and selected the Blank Function option. I added the lambda function found on AWS gateway getting started guide:
exports.handler = function(event, context, callback) {
callback(null, {"Hello":"World"}); // SUCCESS with message
};
The issue is that when I make a GET request to it, it's returning back a 502 response { "message": "Internal server error" }
. And the logs say "Execution failed due to configuration error: Malformed Lambda proxy response".
回答1:
Usually, when you see Malformed Lambda proxy response
, it means your response from your Lambda function doesn't match the format API Gateway is expecting, like this
{
"isBase64Encoded": true|false,
"statusCode": httpStatusCode,
"headers": { "headerName": "headerValue", ... },
"body": "..."
}
If you are not using Lambda proxy integration, you can login to API Gateway console and uncheck the Lambda proxy integration checkbox.
Also, if you are seeing intermittent Malformed Lambda proxy response
, it might mean the request to your Lambda function has been throttled by Lambda, and you need to request a concurrent execution limit increase on the Lambda function.
回答2:
If lambda is used as a proxy then the response format should be
{
"isBase64Encoded": true|false,
"statusCode": httpStatusCode,
"headers": { "headerName": "headerValue", ... },
"body": "..."
}
Note : The body should be stringified
回答3:
Yeah so I think this is because you're not actually returning a proper http response there which is why you're getting the error.
personally I use a set of functions like so:
module.exports = {
success: (result) => {
return {
statusCode: 200,
headers: {
"Access-Control-Allow-Origin" : "*", // Required for CORS support to work
"Access-Control-Allow-Credentials" : true // Required for cookies, authorization headers with HTTPS
},
body: JSON.stringify(result),
}
},
internalServerError: (msg) => {
return {
statusCode: 500,
headers: {
"Access-Control-Allow-Origin" : "*", // Required for CORS support to work
"Access-Control-Allow-Credentials" : true // Required for cookies, authorization headers with HTTPS
},
body: JSON.stringify({
statusCode: 500,
error: 'Internal Server Error',
internalError: JSON.stringify(msg),
}),
}
}
} // add more responses here.
Then you simply do:
var responder = require('responder')
// some code
callback(null, responder.success({ message: 'hello world'}))
回答4:
From the AWS docs
In a Lambda function in Node.js, To return a successful response, call
callback(null, {"statusCode": 200, "body": "results"}). To throw an
exception, call callback(new Error('internal server error')). For a
client-side error, e.g., a required parameter is missing, you can call
callback(null, {"statusCode": 400, "body": "Missing parameters of
..."}) to return the error without throwing an exception.
回答5:
A very very special case, if you pass the headers directly there is a chance you have this header:
"set-cookie": [ "........" ]
But Amazon needs this:
"set-cookie": "[ \\"........\\" ]"
回答6:
For anyone else who struggles when the response appears valid. This does not work:
callback(null,JSON.stringify( {
isBase64Encoded: false,
statusCode: 200,
headers: { 'headerName': 'headerValue' },
body: 'hello world'
})
but this does:
callback(null,JSON.stringify( {
'isBase64Encoded': false,
'statusCode': 200,
'headers': { 'headerName': 'headerValue' },
'body': 'hello world'
})
Also, it appears that no extra keys are allowed to be present on the response object.
回答7:
I had this error because I accidentally removed the variable ServerlessExpressLambdaFunctionName from the CloudFormation AWS::Serverless::Api resource. The context here is https://github.com/awslabs/aws-serverless-express "Run serverless applications and REST APIs using your existing Node.js application framework, on top of AWS Lambda and Amazon API Gateway"
回答8:
If you're using Go with https://github.com/aws/aws-lambda-go, you have to use events.APIGatewayProxyResponse
.
func hello(ctx context.Context, event ImageEditorEvent) (events.APIGatewayProxyResponse, error) {
return events.APIGatewayProxyResponse{
IsBase64Encoded: false,
StatusCode: 200,
Headers: headers,
Body: body,
}, nil
}
回答9:
In case the above doesn't work for anyone, I ran into this error despite setting the response variable correctly.
I was making a call to an RDS database in my function. It turned out that what was causing the problem was the security group rules (inbound) on that database.
You'll probably want to restrict the IP addresses that can access the API, but if you want to get it working quick / dirty to test out if that change fixes it you can set it to accept all like so (you can also set the range on the ports to accept all ports too, but I didn't do that in this example):
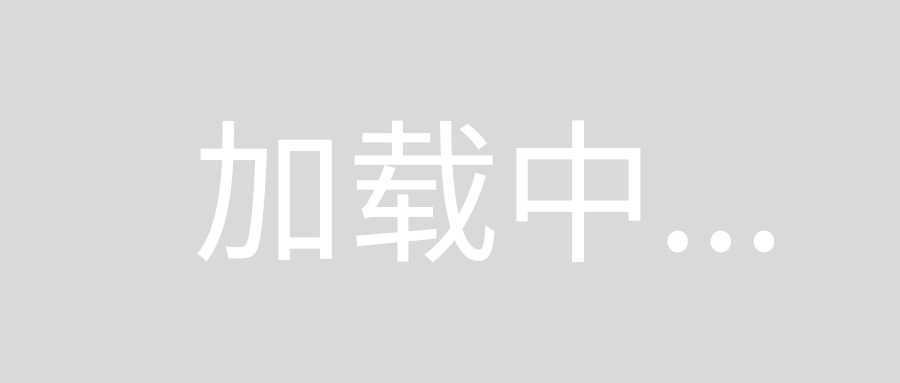
回答10:
I've tried all of above suggestion but it doesn't work while body
value is not String
return {
statusCode: 200,
headers: {
"Content-Type": "application/json",
"Access-Control-Allow-Origin": "*"
},
body: JSON.stringify({
success: true
}),
isBase64Encoded: false
};