可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have a Spring MVC application and a problem with JUnit tests combined with the file applicationContext.xml.
In my JUnit test class I write:
final ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
service = (TestServiceImpl) context.getBean("testServiceImpl");
The error I get is that aplicationContect.xml can not be found:
org.springframework.beans.factory.BeanDefinitionStoreException: IOException parsing XML document from class path resource [applicationContext.xml]; nested exception is java.io.FileNotFoundException: class path resource [applicationContext.xml] cannot be opened because it does not exist
But it exits in the WEB-INF folder. Here you can see the tree of my project with the applicationContext.xml file:
http://img28.imageshack.us/img28/2576/treexp.png
So, what's wrong here? Why does the file not exist for the JUnit test?
Thank you in advance & Best Regards.
回答1:
You should keep your Spring files in another folder, marked as "source" (just like "src" or "resources").
WEB-INF is not a source folder, therefore it will not be included in the classpath (i.e. JUnit will not look for anything there).
回答2:
The ClassPathXmlApplicationContext
isn't going to find the applicationContext.xml
in your WEB-INF
folder, it's not on the classpath. You could copy the application context into your classpath (could put it under src/test/resources
and let Maven copy it over) when running the tests.
回答3:
If you use maven, create a directory called resources
in the main directory, and then copy your applicationContext.xml
into it.
From your java code call:
ApplicationContext appCtx = new ClassPathXmlApplicationContext("applicationContext.xml");
回答4:
I got the same error.
I solved it moving the file applicationContext.xml
in a
sub-folder of the src
folder. e.g:
context = new ClassPathXmlApplicationContext("/com/ejemplo/dao/applicationContext.xml");
回答5:
I also found this problem. What do did to solve this is to copy/paste this file everywhere and run, one file a time. Finally it compiled and ran successfully, and then delete the unnecessary ones. The correct place in my situation is:
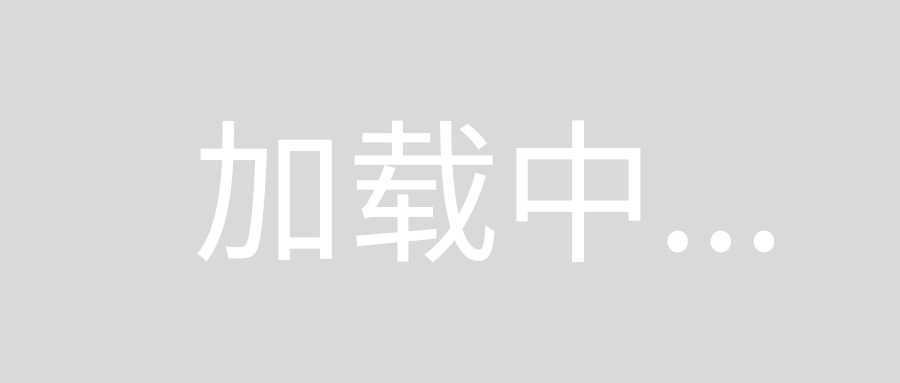
This is under the /src/ path (I am using Intellij Idea as the IDE). The other java source files are under /src/com/package/ path
Hope it helpes.
回答6:
I solved it moving the file spring-context.xml in a src folder.
ApplicationContext context = new ClassPathXmlApplicationContext("spring-context.xml");
回答7:
This happens to me from time to time when using eclipse
For some reason (eclipse bug??) the "excluded" parameter gets a value *.* (build path for my resources folder)
Just change the exclusion to none (see red rectangle vs green rectangle)
I hope this helps someone in the future because it was very frustrating to find.
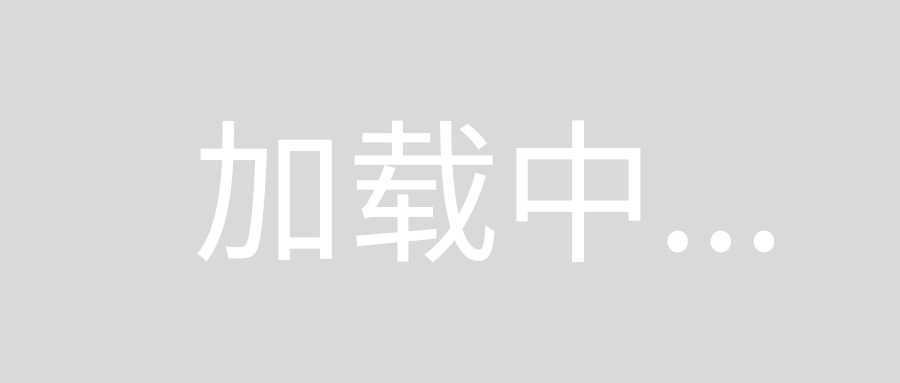
回答8:
I fixed it by adding applicationContext.xml into jar/target/test-classes for Maven project. And use
XmlBeanFactory bf = new XmlBeanFactory( new ClassPathResource(
"/applicationContext.xml", getClass() ) )
instead of
XmlBeanFactory bf = new XmlBeanFactory( new ClassPathResource(
"/WEB-INF/applicationContext.xml", getClass() ) )
回答9:
Click on the src/main/java
folder and right click and create the xml file. If you create the application.xml
file in a subpackage other than /
, it will not work.
Know your structure is look like this
package/
| subpackage/
| Abc.java
| Test.java
/application.xml
回答10:
I placed the applicationContext.xml in the src/main/java folder and it worked
回答11:
In Spring all source files are inside src/main/java.
Similarly, the resources are generally kept inside src/main/resources.
So keep your spring configuration file inside resources folder.
Make sure you have the ClassPath entry for your files inside src/main/resources as well.
In .classpath check for the following 2 lines. If they are missing add them.
<classpathentry path="src/main/java" kind="src"/>
<classpathentry path="src/main/resources" kind="src" />
So, if you have everything in place the below code should work.
ApplicationContext ctx = new ClassPathXmlApplicationContext("Spring-Module.xml");
回答12:
Please do This code - it worked
AbstractApplicationContext context= new ClassPathXmlApplicationContext("spring-config.xml");
o/w: Delete main method class and recreate it while recreating please uncheck Inherited abstract method its worked
回答13:
I'm using Netbeans, i solved my problem by putting the file in: Other Sources default package, then i called it in this way:
ApplicationContext context =new ClassPathXmlApplicationContext("bean.xml");
resources folder
回答14:
While working with Maven got same issue then I put XML file into src/main/java
path and it worked.
ApplicationContext context=new ClassPathXmlApplicationContext("spring.xml");
回答15:
You actually need to understand the ApplicationContext
. It is an interface and it will have different implementations based on configuration.
As you are using new ClassPathXmlApplicationContext("applicationContext.xml");
, kindly pay attention to initial right hand-side , it says ClassPathXmlApplicationContext , so the XML must be present in the class path.
So drag your applicationContext.xml wherever it is to the src folder.
Gist: new ClassPathXmlApplicationContext as the name
[ClassPathXml]will look for the xml file in the src folder of your
project, so drag your xml file there only.
ClassPathXmlApplicationContext
—Loads a context definition from an XML
file located in the classpath, treating context definition files as classpath
resources.
FileSystemXmlApplicationContext
—Loads a context definition from an XML
file in the file system.
XmlWebApplicationContext
—Loads context definitions from an XML file contained
within a web application.