I have been using
UIApplication.sharedApplication().setStatusBarStyle()
In my appDelegate and it has worked fine, but since iOS 9, this method is deprecated and I can't find an alternative.
I want to change the statusbar style to .LightContent for my whole application,
but the only suggestion xCode gives me is to handle this in every VC separately with;
override func preferredStatusBarStyle() -> UIStatusBarStyle {
return .LightContent
}
Has anyone an idea how to do this for the whole application?
Thanks in advance
I think I have found a solution.
I ended up setting the
View controller-based status bar appearance
boolean to NO
In my info.plist file.
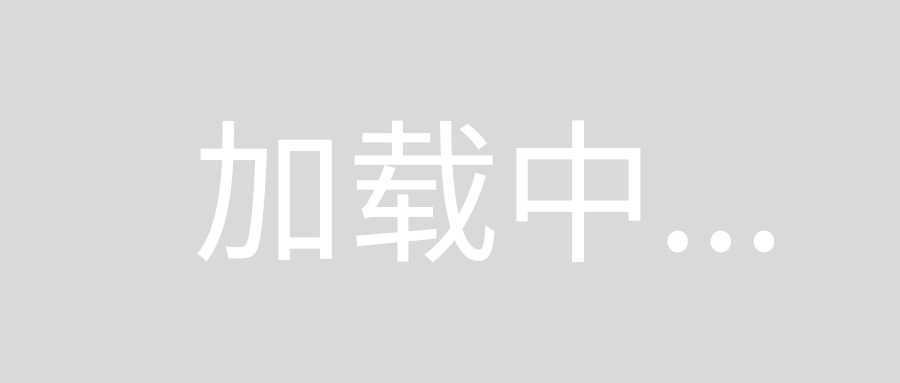
Then I went to my target's General settings -> Deployment info
and changed the dropdown option
Status Bar Style
to Light
instead of Default
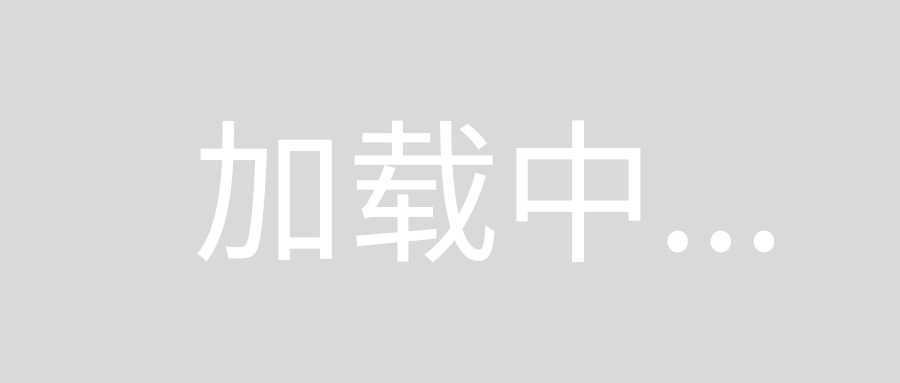
This changed the statusbar style to Light
for my whole application, just what I wanted.
I Hope this helps!
In Swift 3 is like that:
UIApplication.shared.statusBarStyle = .lightContent
In swift 3.
In your view controller:
override var preferredStatusBarStyle: UIStatusBarStyle {
return UIStatusBarStyle.lightContent
}
If you wish when the app run your launch screen also has the status bar in lightContent then:
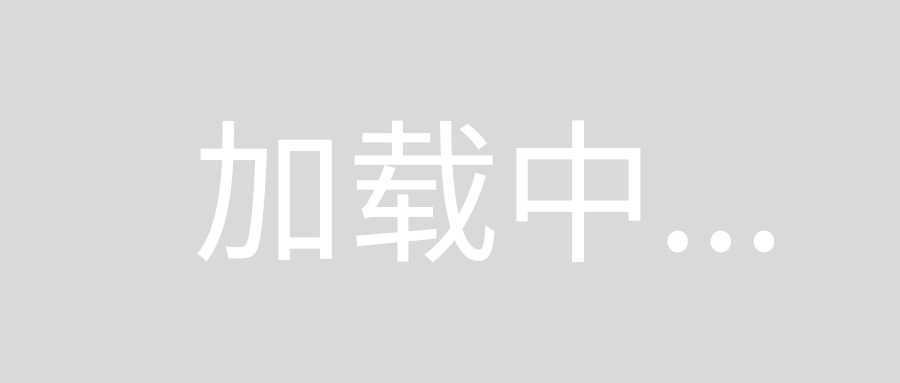
This worked fine for me in Xcode 7.
In AppDelegate:
UIApplication.sharedApplication().statusBarStyle = .LightContent
for those still working with Swift 3 in Xcode 8:
( slightly different to Marlon Ruiz's answer above, not an override function, but within viewDidLoad )
override func viewDidLoad() {
super.viewDidLoad()
var preferredStatusBarStyle: UIStatusBarStyle {
return .lightContent
}
}
To dynamically update UIStatusBarStyle on view controllers use this method
this will also remove deprecated warning
'setStatusBarStyle:' is deprecated: first deprecated in iOS 9.0 - Use -[UIViewController preferredStatusBarStyle]
for calling
[[UIApplication sharedApplication] setStatusBarStyle:style];
Let's Get Started
Objective - C
define UtilityFunction
+(void)setStatusBarStyle:(UIStatusBarStyle )style {
[[NSUserDefaults standardUserDefaults] setInteger:style forKey:@"UIStatusBarStyle"];
[[NSUserDefaults standardUserDefaults] synchronize];
}
over-ride this method in your BaseViewController
- (UIStatusBarStyle)preferredStatusBarStyle {
UIStatusBarStyle style = [[NSUserDefaults standardUserDefaults] integerForKey:@"UIStatusBarStyle"];
return style;
}
set UIStatusBarStyle value for the AnyViewController using a UtilityFunction like below:
[UtilityFunctions setStatusBarStyle:UIStatusBarStyleDefault];
// call below code for preferred style
[self preferredStatusBarStyle];
Swift 4.0
define UtilityFunction
class func setPreferedStyle(style:UIStatusBarStyle)->Void {
UserDefaults.standard.set(style, forKey: "UIStatusBarStyle")
UserDefaults.standard.synchronize()
}
over-ride this method in your BaseViewController
override var preferredStatusBarStyle: UIStatusBarStyle {
if let style: UIStatusBarStyle = UIStatusBarStyle(rawValue:UserDefaults.standard.integer(forKey: "UIStatusBarStyle")) {
return style
}
return UIStatusBarStyle.lightContent
}
set UIStatusBarStyle value for the AnyViewController using a UtilityFunction like below:
Utility.setPreferedStyle(style: .lightContent)
// call below code for preferred style
preferredStatusBarStyle()
This is the new way in AppDelegate:
UIApplication.sharedApplication().setStatusBarStyle(UIStatusBarStyle.LightContent, animated: true)
In info.plist, set:
View controller-based status bar appearance
boolean to NO
In app delegate's didFinishLaunchingWithOptions
, use function parameter application
(and not the [UIApplication sharedApplication]
or simillary the UIApplication.sharedApplication()
in swift) to set this like so:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
application.statusBarStyle = UIStatusBarStyleLightContent;
}