I want to create a layout like the below image:
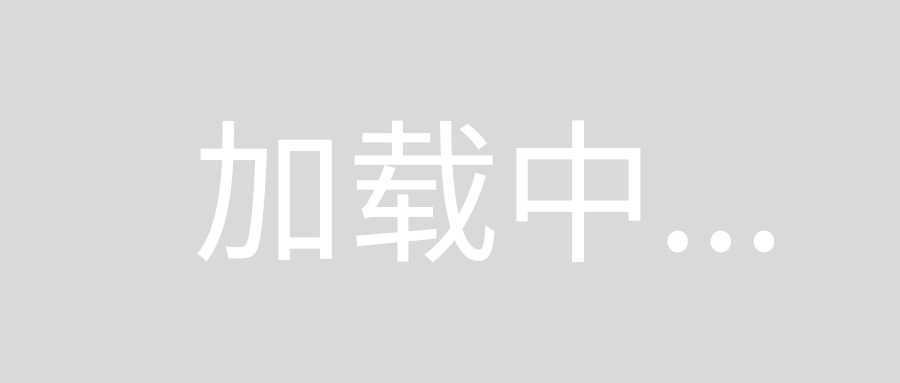
A CoordinatorLayout which contain :
- CollapsingToolbarLayout( contain ImageView & Toolbar)
- RecyclerView
- TabLayout
- ViewPager( that each fragment of it contain a RecyclerView)
I wanna responding to scroll events in this way:
- CollapsingToolbarLayout expand and collapse by scrolling
- Toolbar sticks to the top until TabLayout reach to the top
- After that toolbar scroll up and TabLayout stick to the top
I'm having trouble with the RecyclerView between CollapsingToolbarLayout and TabLayout. I can implement this layout without that RecyclerView( I put CollapsingToolbarLayout and TabLayout inside the AppBarLayout and the ViewPager outside it, inside the CoordinatorLayout).
My Question:
- Where should I put that RecyclerView?
- Which & where
layout_scrollFlags
and layout_behavior
should I set for each layouts?
It seems that AppBarLayout have a limited height. When I put the RecyclerView inside AppBarLayout, only a portion part of the RecyclerView is visible and also TabLayout disappear.
I read lots of tutorials like this one and lots of questions like this one and this one, but non of them help me.
use this as a main layout
activity_main
<?xml version="1.0" encoding="utf-8"?>
<android.support.v4.widget.SwipeRefreshLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/swipe_refresh_layout_profile"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:ignore="RtlHardcoded">
<android.support.design.widget.CoordinatorLayout
android:id="@+id/co_profile_activity_root_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/white"
android:visibility="visible">
<android.support.design.widget.AppBarLayout
android:id="@+id/appbar_profile"
android:layout_width="match_parent"
android:layout_height="@dimen/profile_img_placeholder_height"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar">
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/collapse_toolbar_profile"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_scrollFlags="scroll|exitUntilCollapsed|snap">
<RelativeLayout
android:id="@+id/rel_top"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop">
<ImageView
android:id="@+id/img_bg_placeholder_profile"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"
android:tint="#11000000"
app:layout_collapseMode="parallax"
app:layout_collapseParallaxMultiplier="0.9" />
<LinearLayout
android:id="@+id/lin_top_inner"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#BF473e6b"
android:orientation="vertical"
android:scaleType="centerCrop">
</LinearLayout>
</RelativeLayout>
<FrameLayout
android:id="@+id/frame_detail_profile"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center|center_horizontal"
android:orientation="vertical"
app:layout_collapseMode="parallax"
app:layout_collapseParallaxMultiplier="0.3">
<android.support.v7.widget.RecyclerView
android:layout_width="wrap_content"
android:layout_height="wrap_content">
</android.support.v7.widget.RecyclerView>
</FrameLayout>
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar_profile"
android:layout_width="match_parent"
android:layout_height="@dimen/profile_toolbar_height"
android:gravity="top|center"
app:layout_anchor="@id/frame_detail_profile"
app:layout_collapseMode="pin"
app:theme="@style/ThemeOverlay.AppCompat.Dark"
app:title="">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/tv_toolbar_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="@dimen/profile_toolbar_title_left_margin"
android:gravity="center_vertical|center"
android:ellipsize="end"
android:singleLine="true"
android:layout_gravity="center"
android:textColor="@android:color/white"
android:textSize="20sp" />
</LinearLayout>
</android.support.v7.widget.Toolbar>
<android.support.design.widget.TabLayout
android:id="@+id/tab_layout_profile"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:layout_gravity="bottom"
android:layout_marginTop="@dimen/profile_tab_layout_top_margin"
android:background="@color/white"
app:tabIndicatorColor="@color/colorPrimary"
app:tabSelectedTextColor="@color/colorPrimary"
app:tabTextColor="@color/charcoal_grey" />
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
<android.support.v4.view.ViewPager
android:id="@+id/view_pager_profile"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior" />
</android.support.design.widget.CoordinatorLayout>
</RelativeLayout>
</android.support.v4.widget.SwipeRefreshLayout>
and for grid layout of tabs use adapter classes.
Start with AppBarLayout
under which you add CollapsingToolbarLayout
with scrollFlags="scroll|exitUntilCollapsed",
add LinearLayout
with vertical orientation to CollapsingToolbarLayout
and add the
Two things to be done:-
- When the search button is clicked, set the visibility of recycler view to VISIBLE
- When the back button is pressed, set the visibility of recycler view to GONE
Following are the implementations:
1. Setting the visibility of recycler view to VISIBLE:
public boolean onOptionsItemSelected(MenuItem item) {
if (item.getItemId() == R.id.searchView) {
rView.setVisibility(VISIBLE);
}
return true;
}
2. Setting the visibility of recycle adapter to GONE
MenuItem searchMenuItem = menu.findItem(R.id.searchView);
MenuItemCompat.setOnActionExpandListener(searchMenuItem, new MenuItemCompat.OnActionExpandListener() {
@Override
public boolean onMenuItemActionExpand(MenuItem item) {
return true;
}
@Override
public boolean onMenuItemActionCollapse(MenuItem item) {
recyclerView.setVisibility(GONE);
return true;
}
});
NOTE: Do not forget to keep the visibilty as GONE initially when the activity is started