I need to check my Android app's Internet consumption. In my app I have a numerous number of web service APIs being called.
I want to know how much my app consumes the Internet in kB/MB at a full go.
How can I check that? Is there any tool to check that?
Android Studio 2.0 Introduce new Network
section in Android Monitor
which can help you with your problem.
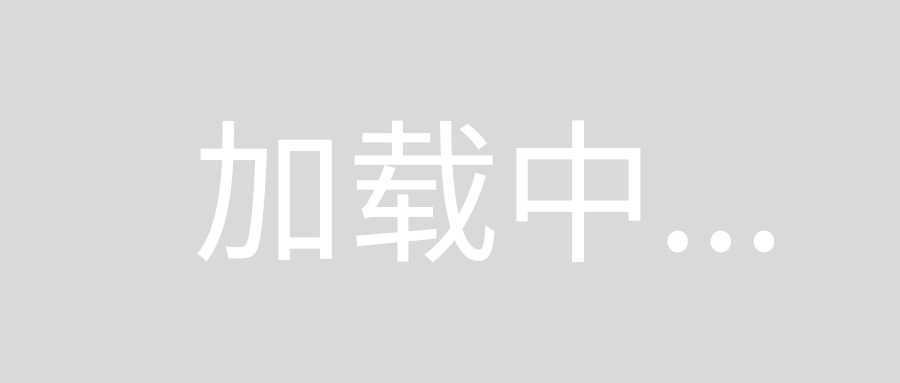
Tx == Transmit Bytes Rx == Receive Bytes
For view purposes, you can check it in the monitor as mentioned by MD.
To store, you can do that programmatically
int UID = android.os.Process.myUid();
rxBytes += getUidRxBytes(UID);
txBytes += getUidTxBytes(UID);
/**
* Read UID Rx Bytes
*
* @param uid
* @return rxBytes
*/
public Long getUidRxBytes(int uid) {
BufferedReader reader;
Long rxBytes = 0L;
try {
reader = new BufferedReader(new FileReader("/proc/uid_stat/" + uid
+ "/tcp_rcv"));
rxBytes = Long.parseLong(reader.readLine());
reader.close();
}
catch (FileNotFoundException e) {
rxBytes = TrafficStats.getUidRxBytes(uid);
//e.printStackTrace();
}
catch (IOException e) {
e.printStackTrace();
}
return rxBytes;
}
/**
* Read UID Tx Bytes
*
* @param uid
* @return txBytes
*/
public Long getUidTxBytes(int uid) {
BufferedReader reader;
Long txBytes = 0L;
try {
reader = new BufferedReader(new FileReader("/proc/uid_stat/" + uid
+ "/tcp_snd"));
txBytes = Long.parseLong(reader.readLine());
reader.close();
}
catch (FileNotFoundException e) {
txBytes = TrafficStats.getUidTxBytes(uid);
//e.printStackTrace();
}
catch (IOException e) {
e.printStackTrace();
}
return txBytes;
}
}
Have a look: Android Monitor.
In that there are many topics that you can monitor.
You will find Network Monitor.
Displaying a Running App in the Network Monitor:
Follow these steps:
- Connect a hardware device.
- Display Android Monitor.
- Click the Network tab.
- Open an app project and run it on the hardware device.
- To start the Network Monitor, click Pause Pause icon to deselect it.
Any network traffic begins to appear in the Network Monitor:
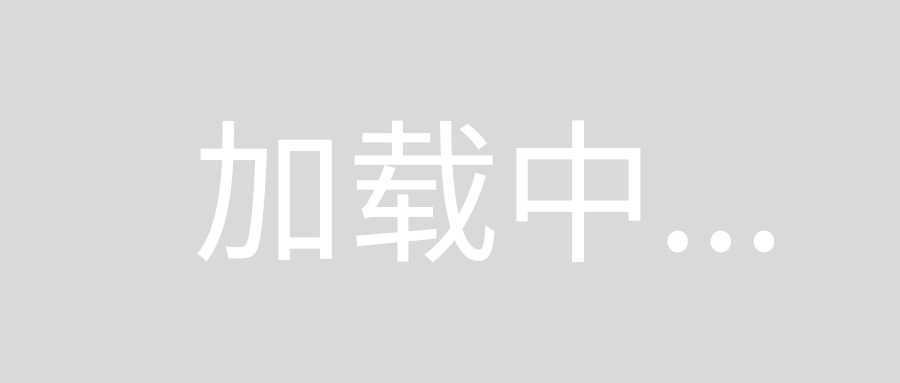
The Network Monitor adds up the amount of time it takes for the device to transmit and receive kilobytes of data. The y-axis is in kilobytes per second. The x-axis starts with seconds, and then minutes and seconds, and so on.
- To stop the Network Monitor, click Pause Pause icon again to select it.
Reference: Android Monitor