可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
(self asking and self-answering because I spent hours on the web looking for this, and most of the resources all say "I solved it in the end" without giving an explanation)
I had a very simple Core Data + Bindings application:
- An NSArrayController pulling items out of Core Data
- An NSTableView rendering them
- Another NSTableView that when you click on a row in the first table, displays the details of that item
Item 3 above was causing application crash, with the error:
[(my NSManagedObject) copyWithZone:]: unrecognized selector sent to instance
Implementing that method (!) and putting a breakpoint there, I found it was being invoked by Apple's NSCell class - this didn't much help :(.
回答1:
Turns out ALL the above are needed to trigger this, and XCode is allowing you to do something that's wrong in 99.9% of situations, if not 100%.
Core-Data objects cannot implement copyWithZone: - this causes the crash
When a tableview is populated using Bindings, it tries to copy the values of the objects in the NSArrayController to render each Column
...but if you fail to specify the Column binding fully (Xcode allows you to half specify it), then tableview tries the "copy" on the objects instead of their values
The bug in my bindings: I had specified that a Table Column had a "value" with:
Controller Key = "arrangedObjects"
Model Key Path = (blank)
(this is a bug in XCode4 autocomplete - it will delete the ModelKeyPath field sometimes when you tab away too quickly)
Finish typing-in the binding, e.g.:
Model Key Path = "value"
...and everything works again.
回答2:
I would also like to post here because I had a similar issue with the same type of thing
empty defined object:
Wrong code:
@property (nonatomic, copy, readwrite) MSender * sender;
this should trow a compile error because with reference counting Xcode has no idea how to copy my object. Instead it fails at run time...
Write code:
@property (nonatomic, strong, readwrite) MSender * sender;
Hope it helps someone.
回答3:
Thank you for this solution, it pointed me in the right direction. For those of you learning InterfaceBuilder I hope this additional information helps.
It turns out while building a tutorial I had inadvertently Bound a Text field Cell - Text Cell
to my ArrayController.ObjectValue
.
The real binding was supposed to happen at
Table Column > Table Cell View > Static Text - Table View Cell
That one was correct, but visually in the tree below it (for reasons I don't understand yet IB needs a Text Field Cell
.) I had also bound:
Table Column > Text Field Cell - Text Cell
It was that second binding which trying to copy the whole object because the path was objectValue
with no key, it was triggering this error.
Probably a newbie mistake, but it meant walking through EVERY object and checking for bindings and I came across this one.
回答4:
As a hint to avoid any kind of problems with the binding one can convert the NSManagedObject
into NSDictionary
while populating the controllers with:[object dictionaryWithValuesForKeys:[[object entity] attributeKeys]]
, it is a lot easier to connect and debug :-)
Thanks for the pointer by the way!
回答5:
I encountered this error when I tried to implement a master-detail binding. Something analogous to Department / Employee. I tried to bind an arrayController to "selection.employees" of Department and got:
-[Employee copyWithZone:]: unrecognized selector sent to instance
Turned out the employees relationship was not set to to-many, as intended, but to-one. Once I corrected this, all was well.
回答6:
I also had a similar problem and it came from calling a ProxyObject rather than the actual object from an array controller.
For example:
MYEntity *entity = EntityController.selection;
NSString *property = entity.property; // <--- causes the error
but
MYEntity *entity = EntityController.selectedObjects firstObject]; // <--- fixes the error
NSString *property = entity.property;
回答7:
Accidentally faced with this issue.
After a little investigation I found out that the reason was wrong cocoa binding option set on NSTextField
-cell object.
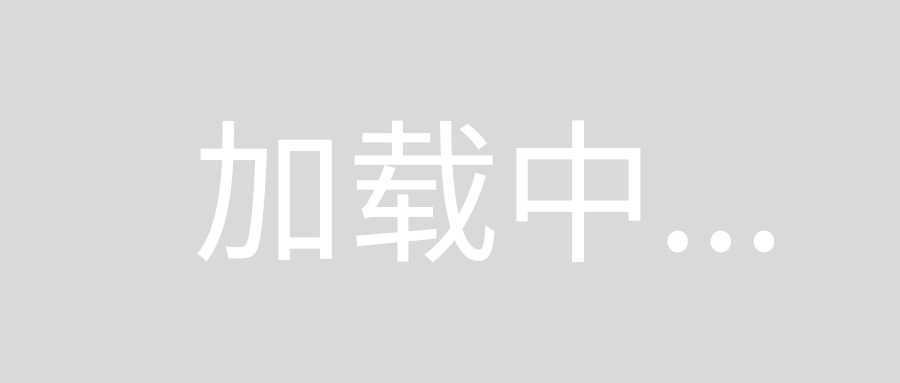
The problem was fixed by switch the binding off.
回答8:
I got this when I returned a view from the NSTableViewDataSource
function -tableView:objectValueForTableColumn:row:
. Instead, it is supposed to return a value (e.g. NSString*
). (Not surprising given its name, but the error is not helpful in discovering this. The function I was thinking of is -tableView:viewForTableColumn:row:
in NSTableViewDelegate
.)