Trying to write an Angular 2 pipe that will take a JSON object string and return it pretty-printed/formatted to display to the user.
For example, it would take this:
{
"id": 1,
"number": "K3483483344",
"state": "CA",
"active": true
}
And return something that looks like this when displayed in HTML:
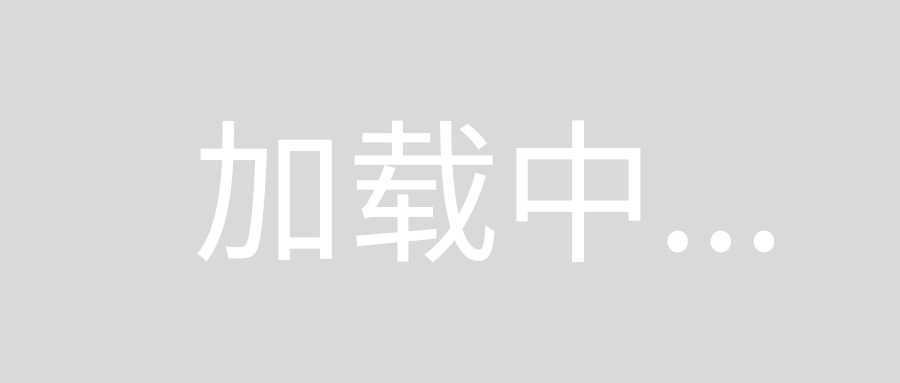
So in my view I could have something like:
<td> {{ record.jsonData | prettyprint }} </td>
I would like to add an even simpler way to do this, using the built-in json
pipe:
<pre>{{data | json}}</pre>
This way, the formatting is preserved.
I would create a custom pipe for this:
@Pipe({
name: 'prettyprint'
})
export class PrettyPrintPipe implements PipeTransform {
transform(val) {
return JSON.stringify(val, null, 2)
.replace(' ', ' ')
.replace('\n', '<br/>');
}
}
and use it this way:
@Component({
selector: 'my-app',
template: `
<div [innerHTML]="obj | prettyprint"></div>
`,
pipes: [ PrettyPrintPipe ]
})
export class AppComponent {
obj = {
test: 'testttt',
name: 'nameeee'
}
}
See this stackblitz: https://stackblitz.com/edit/angular-prettyprint