可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I read the documentation: http://developer.android.com/guide/topics/ui/controls/pickers.html
but now in lollipop appears the calendar (it is ok for an event but horrible for set a date of birth, I would a spinner mode.) and I can't remove it!
In layout It's easy with this property:
<DatePicker
datePickerMode="spinner"...>
but from code of the DatePickerDialog if I try to set
dialogDatePicker.getDatePicker().setSpinnersShown(true);
dialogDatePicker.getDatePicker().setCalendarViewShown(false);
These properties do not work and the calendar continues to appear!
public static class MyDatePicker extends DialogFragment implements DatePickerDialog.OnDateSetListener {
int pYear;
int pDay;
int pMonth;
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
// Use the current date as the default date in the picker
final Calendar c = Calendar.getInstance();
int year = c.get(Calendar.YEAR);
int month = c.get(Calendar.MONTH);
int day = c.get(Calendar.DAY_OF_MONTH);
DatePickerDialog dialogDatePicker = new DatePickerDialog(getActivity(), this, year, month, day);
dialogDatePicker.getDatePicker().setSpinnersShown(true);
dialogDatePicker.getDatePicker().setCalendarViewShown(false);
return dialogDatePicker;
// Create a new instance of DatePickerDialog and return it
//return new DatePickerDialog(getActivity(), this, year, month, day);
}
public void onDateSet(DatePicker view, int year, int month, int day) {
pYear = year;
pDay = day;
pMonth = month;
}
}
回答1:
DatePickerDialog uses the dialog theme specified by your activity theme. This is a fully-specified theme, which means you need to re-specify any attributes -- such as the date picker style -- that you've set in your activity theme.
<style name="MyAppTheme" parent="android:Theme.Material">
<item name="android:dialogTheme">@style/MyDialogTheme</item>
<item name="android:datePickerStyle">@style/MyDatePicker</item>
</style>
<style name="MyDialogTheme" parent="android:Theme.Material.Dialog">
<item name="android:datePickerStyle">@style/MyDatePicker</item>
</style>
<style name="MyDatePicker" parent="android:Widget.Material.DatePicker">
<item name="android:datePickerMode">spinner</item>
</style>
Note: Due to Issue 222208, this will not work in Android N / API 24. It has already been fixed in the platform for the next release. There is no workaround available for API 24 devices.
回答2:
Apply the Holo_theme in the code level got worked for me.
new DatePickerDialog(getActivity(),android.R.style.Theme_Holo_Dialog,this, year,month, day);
回答3:
Add the attribute calendarViewShown and set it to false:
<DatePicker
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/date_field"
android:calendarViewShown="false"/>
回答4:
I think the best solution to this problem is define a new style in the XML file and then use it programatically in the code.
Though @alanv answer is perfect and will work correctly, the problem with it is that it will be applied for all the Date pickers. Suppose you have one DatePicker where you need to show Calendar view, and another DatePicker where you need to show spinner view, this will not work.
So define a new style in a styles.xml. Create this file in a folder named values-v21 (see naming structure here), since these attributes are introduced post lollipop only. Before lollipop, there was only spinner mode.
<style name="CustomDatePickerDialogTheme" parent="android:Theme.Material.Light.Dialog">
<item name="android:datePickerStyle">@style/MyDatePickerStyle</item>
</style>
<style name="MyDatePickerStyle" parent="@android:style/Widget.Material.DatePicker">
<item name="android:datePickerMode">spinner</item>
</style>
And finally, use it in the code like this
DatePickerDialog datePickerDialog = new DatePickerDialog(getActivity(),
R.style.CustomDatePickerDialogTheme, this, year, month, day);
return datePickerDialog;
回答5:
You can use a library to create the old "spinner" style DatePicker which is just three NumberPicker put together:
https://github.com/drawers/SpinnerDatePicker
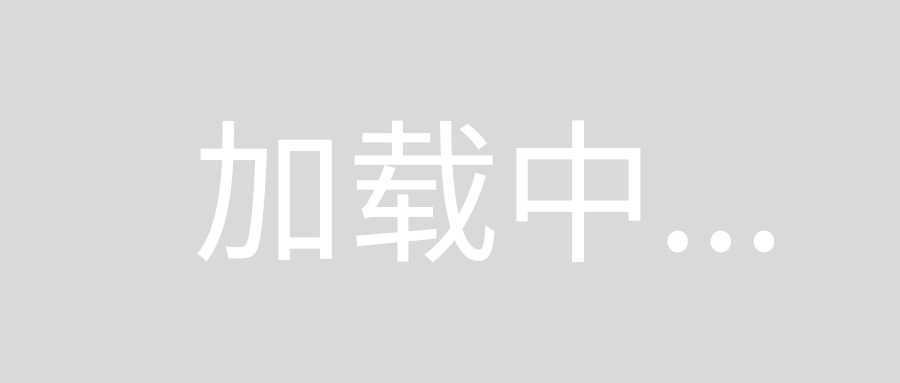
First, define a style:
<style name="NumbePickerStyle">
<item name="android:textSize">22dp</item>
<item name="android:textColorPrimary">@color/colorAccent</item>
<item name="android:colorControlNormal" tools:targetApi="lollipop">@color/colorAccent</item>
</style>
Then,
new SpinnerDatePickerDialogBuilder()
.context(MainActivity.this)
.callback(MainActivity.this)
.spinnerTheme(spinnerTheme)
.year(year)
.monthOfYear(monthOfYear)
.dayOfMonth(dayOfMonth)
.build()
.show();
Disclaimer: I am the author of this library
回答6:
Can you try this
DatePickerDialog dialogDatePicker = new DatePickerDialog(getActivity(), this, year, month, day);
dialogDatePicker.getDatePicker().setCalendarViewShown(false);
return dialogDatePicker;
removing
dialogDatePicker.getDatePicker().setSpinnersShown(true);
回答7:
All fancy stuff aside, use the DatePickerDialog constructor that takes an "int theme" parameter.
Without it, it seems to default to whichever int sets it to CalendarView mode.
With small integers (0, 1, 2, 3...) it will give you a spinner (at least in my API 19 app run on an emulator).
Basically, try
new DatePickerDialog( getContext(), 0, this, year, month, day )
Notice the 0 (or 1, or 2, etc) there
Caution:
I'm not sure if there's a list somewhere of static theme fields, and using a hardcoded "0" instead of "Theme.HOLO_LIGHT" or whatever could make a difference on different devices, but it gets the job done somehow.
回答8:
Try this it worked for me, do a custom datelistener:
@SuppressWarnings("deprecation")
public void setDate(View view) {
showDialog(999);
}
@SuppressWarnings("deprecation")
protected Dialog onCreateDialog(int id) {
// TODO Auto-generated method stub
if (id == 999) {
return new DatePickerDialog(this, myDateListener, year, month-1, day);
}
return null;
}
//THIS
private DatePickerDialog.OnDateSetListener myDateListener= new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker arg0, int arg1, int arg2, int arg3) {
showDate(arg1, arg2+1, arg3);
}
};
private void showDate(int year, int month, int day) {
//dateView.setText(new StringBuilder().append(day).append("-")
// .append(month).append("-").append(year));
}
回答9:
DatePicker methods related to showing spinner and/or calendar view are deprecated from API level 24. Method setSpinnersShown(boolean shown) and setCalendarViewShown(boolean shown) are deprecated because calendar mode material style doesn't support the feature.
If you want to use material style in spinner mode, use DatePicker widget.
Apply below style to your date picker xml element to get material spinner. For more information on date picker and styles see http://www.zoftino.com/android-datepicker-example .
<style name="MyDatePickerSpinnerStyle" parent="@android:style/Widget.Material.DatePicker">
<item name="android:datePickerMode">spinner</item>
<item name="android:calendarViewShown">false</item>
<item name="colorControlNormal">#d50000</item>
</style>