可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
The app I'm currently working on uses a lot of ImageViews as buttons. The graphics on these buttons use the alpha channel to fade out the edges of the button and make them look irregular. Currently, we have to generate 2 graphics for each button (1 for the selected/focused/pressed state and the other for the default unselected state) and use a StateListDrawable defined in an XML file for each button.
While this works fine it seems extremely wasteful since all of the selected graphics are simply tinted versions of the unselected buttons. These take time to produce (however little) and take up space in the final APK. It seems like there should be an easy way to this automatically.
The perfect solution, it would seem, is to use ImageViews for each button and specify in its tint attribute a ColorStateList. This approach has the advantage that only a single XML ColorStateList is needed for all of the buttons (that share the same tint). However it does not work. As mentioned here, ImageView throws a NumberFormatException when the value provided to tint is anything other than a single color.
My next attempt was to use a LayerDrawable for the selected drawable. Inside the layer list, we would have the original image at the bottom of the stack covered by a semi-transparent rectangle. This worked on the solid parts of the button graphic. However the edges which were supposed to be entirely transparent, were now the same color as the top layer.
Has anyone encountered this issue before and found a reasonable solution? I would like to stick to XML approaches but will probably code up a simple ImageView subclass that will do the required tinting in code.
回答1:
You can combine StateListDrawable
and LayerDrawable
for this.
public Drawable getDimmedDrawable(Drawable drawable) {
Resources resources = getContext().getResources();
StateListDrawable stateListDrawable = new StateListDrawable();
LayerDrawable layerDrawable = new LayerDrawable(new Drawable[]{
drawable,
new ColorDrawable(resources.getColor(R.color.translucent_black))
});
stateListDrawable.addState(new int[]{android.R.attr.state_pressed}, layerDrawable);
stateListDrawable.addState(new int[]{android.R.attr.state_focused}, layerDrawable);
stateListDrawable.addState(new int[]{android.R.attr.state_selected}, layerDrawable);
stateListDrawable.addState(new int[]{}, drawable);
return stateListDrawable;
}
I assume our colors.xml looks like this
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="translucent_black">#80000000</color>
</resources>
回答2:
For those who have encountered a similar need, solving this in code is fairly clean. Here is a sample:
public class TintableButton extends ImageView {
private boolean mIsSelected;
public TintableButton(Context context) {
super(context);
init();
}
public TintableButton(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public TintableButton(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
init();
}
private void init() {
mIsSelected = false;
}
@Override
public boolean onTouchEvent(MotionEvent event) {
if (event.getAction() == MotionEvent.ACTION_DOWN && !mIsSelected) {
setColorFilter(0x99000000);
mIsSelected = true;
} else if (event.getAction() == MotionEvent.ACTION_UP && mIsSelected) {
setColorFilter(Color.TRANSPARENT);
mIsSelected = false;
}
return super.onTouchEvent(event);
}
}
It's not finished but works well as a proof of concept.
回答3:
Your answer was excellent :)
However instead of creating a button object, I am only calling an OnTouchlistener to change the state of every view.
public boolean onTouch(View v, MotionEvent event) {
if (event.getAction() == MotionEvent.ACTION_DOWN) {
Drawable background = v.getBackground();
background.setColorFilter(0xBB000000, PorterDuff.Mode.SCREEN);
v.setBackgroundDrawable(background);
} else if (event.getAction() == MotionEvent.ACTION_UP) {
Drawable background = v.getBackground();
background.setColorFilter(null);
v.setBackgroundDrawable(background);
}
return true;
}
Gives the same results though
回答4:
I think this solution is simple!
Step 1: Create res/drawable/dim_image_view.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_focused="true" android:drawable="@drawable/dim_image_view_normal"/> <!-- focused -->
<item android:state_pressed="true" android:drawable="@drawable/dim_image_view_pressed"/> <!-- pressed -->
<item android:drawable="@drawable/dim_image_view_normal"/> <!--default -->
</selector>
Step 2: Create res/drawable/dim_image_view_normal.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item><bitmap android:src="@mipmap/your_icon"/></item>
</layer-list>
Step 3: Create res/drawable/dim_image_view_pressed.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android" >
<item><bitmap android:src="@mipmap/your_icon" android:alpha="0.5"></bitmap></item>
</layer-list>
Step 4: Try setting the image in xml layout as:
android:src="@drawable/dim_image_view"
回答5:
I too have irregular looking buttons and needed the tint. In the end, I just resorted to having a state colorlist as my ImageButton
background. Gives the impression that the button color is changing on press and is very straightforward and less compute intensive. I know that this isn't exactly a tint, but it does give it does give necessary visual feedback to the user in what usually is a fleeting moment.
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android" >
<item
android:state_pressed="true"
android:drawable="@android:color/darker_gray" />
<item android:drawable="@android:color/transparent" />
</selector>
回答6:
You can use a class I've created https://github.com/THRESHE/TintableImageButton
Not exactly the best solution but it works.
回答7:
Your answer was also excellent ;)
I am modifying little bit, it will give you result like this:
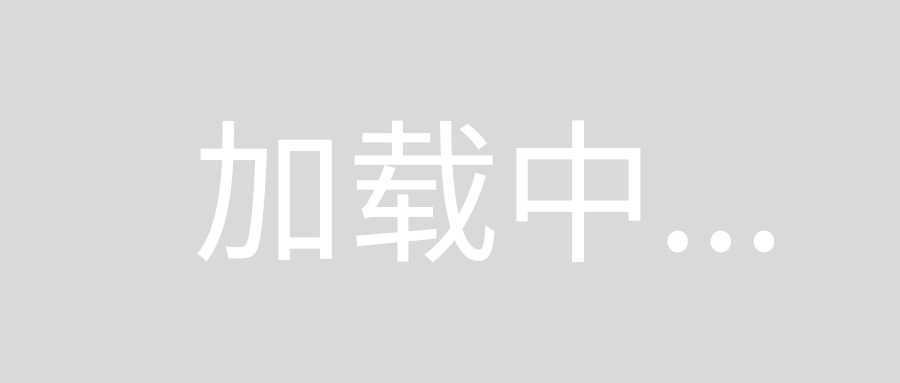
Rect rect;
Drawable background;
boolean hasTouchEventCompleted = false;
@Override
public boolean onTouch(View v, MotionEvent event) {
if (event.getAction() == MotionEvent.ACTION_DOWN) {
rect = new Rect(v.getLeft(), v.getTop(), v.getRight(),
v.getBottom());
hasTouchEventCompleted = false;
background = v.getBackground();
background.setColorFilter(0x99c7c7c7,
android.graphics.PorterDuff.Mode.MULTIPLY);
v.setBackgroundDrawable(background);
} else if (event.getAction() == MotionEvent.ACTION_UP
&& !hasTouchEventCompleted) {
background.setColorFilter(null);
v.setBackgroundDrawable(background);
} else if (event.getAction() == MotionEvent.ACTION_MOVE) {
if (!rect.contains(v.getLeft() + (int) event.getX(), v.getTop()
+ (int) event.getY())) {
// User moved outside bounds
background.setColorFilter(null);
v.setBackgroundDrawable(background);
hasTouchEventCompleted = true;
}
}
//Must return false, otherwise you need to handle Click events yourself.
return false;
}
回答8:
I think this solution is simple enough:
final Drawable drawable = ... ;
final int darkenValue = 0x3C000000;
mButton.setOnTouchListener(new OnTouchListener() {
Rect rect;
boolean hasColorFilter = false;
@Override
public boolean onTouch(final View v, final MotionEvent motionEvent) {
switch (motionEvent.getAction()) {
case MotionEvent.ACTION_DOWN:
rect = new Rect(v.getLeft(), v.getTop(), v.getRight(), v.getBottom());
drawable.setColorFilter(darkenValue, Mode.DARKEN);
hasColorFilter = true;
break;
case MotionEvent.ACTION_MOVE:
if (rect.contains(v.getLeft() + (int) motionEvent.getX(), v.getTop()
+ (int) motionEvent.getY())) {
if (!hasColorFilter)
drawable.setColorFilter(darkenValue, Mode.DARKEN);
hasColorFilter = true;
} else {
drawable.setColorFilter(null);
hasColorFilter = false;
}
break;
case MotionEvent.ACTION_UP:
case MotionEvent.ACTION_CANCEL:
drawable.setColorFilter(null);
hasColorFilter = false;
break;
}
return false;
}
});